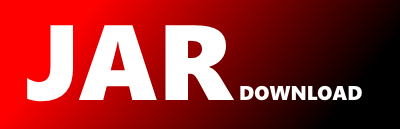
com.pulumi.azurenative.desktopvirtualization.outputs.GetScalingPlanPersonalScheduleResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.desktopvirtualization.outputs;
import com.pulumi.azurenative.desktopvirtualization.outputs.SystemDataResponse;
import com.pulumi.azurenative.desktopvirtualization.outputs.TimeResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetScalingPlanPersonalScheduleResult {
/**
* @return Set of days of the week on which this schedule is active.
*
*/
private @Nullable List daysOfWeek;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return Action to be taken after a user disconnect during the off-peak period.
*
*/
private @Nullable String offPeakActionOnDisconnect;
/**
* @return Action to be taken after a logoff during the off-peak period.
*
*/
private @Nullable String offPeakActionOnLogoff;
/**
* @return The time in minutes to wait before performing the desired session handling action when a user disconnects during the off-peak period.
*
*/
private @Nullable Integer offPeakMinutesToWaitOnDisconnect;
/**
* @return The time in minutes to wait before performing the desired session handling action when a user logs off during the off-peak period.
*
*/
private @Nullable Integer offPeakMinutesToWaitOnLogoff;
/**
* @return Starting time for off-peak period.
*
*/
private @Nullable TimeResponse offPeakStartTime;
/**
* @return The desired configuration of Start VM On Connect for the hostpool during the off-peak phase.
*
*/
private @Nullable String offPeakStartVMOnConnect;
/**
* @return Action to be taken after a user disconnect during the peak period.
*
*/
private @Nullable String peakActionOnDisconnect;
/**
* @return Action to be taken after a logoff during the peak period.
*
*/
private @Nullable String peakActionOnLogoff;
/**
* @return The time in minutes to wait before performing the desired session handling action when a user disconnects during the peak period.
*
*/
private @Nullable Integer peakMinutesToWaitOnDisconnect;
/**
* @return The time in minutes to wait before performing the desired session handling action when a user logs off during the peak period.
*
*/
private @Nullable Integer peakMinutesToWaitOnLogoff;
/**
* @return Starting time for peak period.
*
*/
private @Nullable TimeResponse peakStartTime;
/**
* @return The desired configuration of Start VM On Connect for the hostpool during the peak phase.
*
*/
private @Nullable String peakStartVMOnConnect;
/**
* @return Action to be taken after a user disconnect during the ramp down period.
*
*/
private @Nullable String rampDownActionOnDisconnect;
/**
* @return Action to be taken after a logoff during the ramp down period.
*
*/
private @Nullable String rampDownActionOnLogoff;
/**
* @return The time in minutes to wait before performing the desired session handling action when a user disconnects during the ramp down period.
*
*/
private @Nullable Integer rampDownMinutesToWaitOnDisconnect;
/**
* @return The time in minutes to wait before performing the desired session handling action when a user logs off during the ramp down period.
*
*/
private @Nullable Integer rampDownMinutesToWaitOnLogoff;
/**
* @return Starting time for ramp down period.
*
*/
private @Nullable TimeResponse rampDownStartTime;
/**
* @return The desired configuration of Start VM On Connect for the hostpool during the ramp down phase.
*
*/
private @Nullable String rampDownStartVMOnConnect;
/**
* @return Action to be taken after a user disconnect during the ramp up period.
*
*/
private @Nullable String rampUpActionOnDisconnect;
/**
* @return Action to be taken after a logoff during the ramp up period.
*
*/
private @Nullable String rampUpActionOnLogoff;
/**
* @return The desired startup behavior during the ramp up period for personal vms in the hostpool.
*
*/
private @Nullable String rampUpAutoStartHosts;
/**
* @return The time in minutes to wait before performing the desired session handling action when a user disconnects during the ramp up period.
*
*/
private @Nullable Integer rampUpMinutesToWaitOnDisconnect;
/**
* @return The time in minutes to wait before performing the desired session handling action when a user logs off during the ramp up period.
*
*/
private @Nullable Integer rampUpMinutesToWaitOnLogoff;
/**
* @return Starting time for ramp up period.
*
*/
private @Nullable TimeResponse rampUpStartTime;
/**
* @return The desired configuration of Start VM On Connect for the hostpool during the ramp up phase. If this is disabled, session hosts must be turned on using rampUpAutoStartHosts or by turning them on manually.
*
*/
private @Nullable String rampUpStartVMOnConnect;
/**
* @return Metadata pertaining to creation and last modification of the resource.
*
*/
private SystemDataResponse systemData;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
private GetScalingPlanPersonalScheduleResult() {}
/**
* @return Set of days of the week on which this schedule is active.
*
*/
public List daysOfWeek() {
return this.daysOfWeek == null ? List.of() : this.daysOfWeek;
}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return Action to be taken after a user disconnect during the off-peak period.
*
*/
public Optional offPeakActionOnDisconnect() {
return Optional.ofNullable(this.offPeakActionOnDisconnect);
}
/**
* @return Action to be taken after a logoff during the off-peak period.
*
*/
public Optional offPeakActionOnLogoff() {
return Optional.ofNullable(this.offPeakActionOnLogoff);
}
/**
* @return The time in minutes to wait before performing the desired session handling action when a user disconnects during the off-peak period.
*
*/
public Optional offPeakMinutesToWaitOnDisconnect() {
return Optional.ofNullable(this.offPeakMinutesToWaitOnDisconnect);
}
/**
* @return The time in minutes to wait before performing the desired session handling action when a user logs off during the off-peak period.
*
*/
public Optional offPeakMinutesToWaitOnLogoff() {
return Optional.ofNullable(this.offPeakMinutesToWaitOnLogoff);
}
/**
* @return Starting time for off-peak period.
*
*/
public Optional offPeakStartTime() {
return Optional.ofNullable(this.offPeakStartTime);
}
/**
* @return The desired configuration of Start VM On Connect for the hostpool during the off-peak phase.
*
*/
public Optional offPeakStartVMOnConnect() {
return Optional.ofNullable(this.offPeakStartVMOnConnect);
}
/**
* @return Action to be taken after a user disconnect during the peak period.
*
*/
public Optional peakActionOnDisconnect() {
return Optional.ofNullable(this.peakActionOnDisconnect);
}
/**
* @return Action to be taken after a logoff during the peak period.
*
*/
public Optional peakActionOnLogoff() {
return Optional.ofNullable(this.peakActionOnLogoff);
}
/**
* @return The time in minutes to wait before performing the desired session handling action when a user disconnects during the peak period.
*
*/
public Optional peakMinutesToWaitOnDisconnect() {
return Optional.ofNullable(this.peakMinutesToWaitOnDisconnect);
}
/**
* @return The time in minutes to wait before performing the desired session handling action when a user logs off during the peak period.
*
*/
public Optional peakMinutesToWaitOnLogoff() {
return Optional.ofNullable(this.peakMinutesToWaitOnLogoff);
}
/**
* @return Starting time for peak period.
*
*/
public Optional peakStartTime() {
return Optional.ofNullable(this.peakStartTime);
}
/**
* @return The desired configuration of Start VM On Connect for the hostpool during the peak phase.
*
*/
public Optional peakStartVMOnConnect() {
return Optional.ofNullable(this.peakStartVMOnConnect);
}
/**
* @return Action to be taken after a user disconnect during the ramp down period.
*
*/
public Optional rampDownActionOnDisconnect() {
return Optional.ofNullable(this.rampDownActionOnDisconnect);
}
/**
* @return Action to be taken after a logoff during the ramp down period.
*
*/
public Optional rampDownActionOnLogoff() {
return Optional.ofNullable(this.rampDownActionOnLogoff);
}
/**
* @return The time in minutes to wait before performing the desired session handling action when a user disconnects during the ramp down period.
*
*/
public Optional rampDownMinutesToWaitOnDisconnect() {
return Optional.ofNullable(this.rampDownMinutesToWaitOnDisconnect);
}
/**
* @return The time in minutes to wait before performing the desired session handling action when a user logs off during the ramp down period.
*
*/
public Optional rampDownMinutesToWaitOnLogoff() {
return Optional.ofNullable(this.rampDownMinutesToWaitOnLogoff);
}
/**
* @return Starting time for ramp down period.
*
*/
public Optional rampDownStartTime() {
return Optional.ofNullable(this.rampDownStartTime);
}
/**
* @return The desired configuration of Start VM On Connect for the hostpool during the ramp down phase.
*
*/
public Optional rampDownStartVMOnConnect() {
return Optional.ofNullable(this.rampDownStartVMOnConnect);
}
/**
* @return Action to be taken after a user disconnect during the ramp up period.
*
*/
public Optional rampUpActionOnDisconnect() {
return Optional.ofNullable(this.rampUpActionOnDisconnect);
}
/**
* @return Action to be taken after a logoff during the ramp up period.
*
*/
public Optional rampUpActionOnLogoff() {
return Optional.ofNullable(this.rampUpActionOnLogoff);
}
/**
* @return The desired startup behavior during the ramp up period for personal vms in the hostpool.
*
*/
public Optional rampUpAutoStartHosts() {
return Optional.ofNullable(this.rampUpAutoStartHosts);
}
/**
* @return The time in minutes to wait before performing the desired session handling action when a user disconnects during the ramp up period.
*
*/
public Optional rampUpMinutesToWaitOnDisconnect() {
return Optional.ofNullable(this.rampUpMinutesToWaitOnDisconnect);
}
/**
* @return The time in minutes to wait before performing the desired session handling action when a user logs off during the ramp up period.
*
*/
public Optional rampUpMinutesToWaitOnLogoff() {
return Optional.ofNullable(this.rampUpMinutesToWaitOnLogoff);
}
/**
* @return Starting time for ramp up period.
*
*/
public Optional rampUpStartTime() {
return Optional.ofNullable(this.rampUpStartTime);
}
/**
* @return The desired configuration of Start VM On Connect for the hostpool during the ramp up phase. If this is disabled, session hosts must be turned on using rampUpAutoStartHosts or by turning them on manually.
*
*/
public Optional rampUpStartVMOnConnect() {
return Optional.ofNullable(this.rampUpStartVMOnConnect);
}
/**
* @return Metadata pertaining to creation and last modification of the resource.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetScalingPlanPersonalScheduleResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List daysOfWeek;
private String id;
private String name;
private @Nullable String offPeakActionOnDisconnect;
private @Nullable String offPeakActionOnLogoff;
private @Nullable Integer offPeakMinutesToWaitOnDisconnect;
private @Nullable Integer offPeakMinutesToWaitOnLogoff;
private @Nullable TimeResponse offPeakStartTime;
private @Nullable String offPeakStartVMOnConnect;
private @Nullable String peakActionOnDisconnect;
private @Nullable String peakActionOnLogoff;
private @Nullable Integer peakMinutesToWaitOnDisconnect;
private @Nullable Integer peakMinutesToWaitOnLogoff;
private @Nullable TimeResponse peakStartTime;
private @Nullable String peakStartVMOnConnect;
private @Nullable String rampDownActionOnDisconnect;
private @Nullable String rampDownActionOnLogoff;
private @Nullable Integer rampDownMinutesToWaitOnDisconnect;
private @Nullable Integer rampDownMinutesToWaitOnLogoff;
private @Nullable TimeResponse rampDownStartTime;
private @Nullable String rampDownStartVMOnConnect;
private @Nullable String rampUpActionOnDisconnect;
private @Nullable String rampUpActionOnLogoff;
private @Nullable String rampUpAutoStartHosts;
private @Nullable Integer rampUpMinutesToWaitOnDisconnect;
private @Nullable Integer rampUpMinutesToWaitOnLogoff;
private @Nullable TimeResponse rampUpStartTime;
private @Nullable String rampUpStartVMOnConnect;
private SystemDataResponse systemData;
private String type;
public Builder() {}
public Builder(GetScalingPlanPersonalScheduleResult defaults) {
Objects.requireNonNull(defaults);
this.daysOfWeek = defaults.daysOfWeek;
this.id = defaults.id;
this.name = defaults.name;
this.offPeakActionOnDisconnect = defaults.offPeakActionOnDisconnect;
this.offPeakActionOnLogoff = defaults.offPeakActionOnLogoff;
this.offPeakMinutesToWaitOnDisconnect = defaults.offPeakMinutesToWaitOnDisconnect;
this.offPeakMinutesToWaitOnLogoff = defaults.offPeakMinutesToWaitOnLogoff;
this.offPeakStartTime = defaults.offPeakStartTime;
this.offPeakStartVMOnConnect = defaults.offPeakStartVMOnConnect;
this.peakActionOnDisconnect = defaults.peakActionOnDisconnect;
this.peakActionOnLogoff = defaults.peakActionOnLogoff;
this.peakMinutesToWaitOnDisconnect = defaults.peakMinutesToWaitOnDisconnect;
this.peakMinutesToWaitOnLogoff = defaults.peakMinutesToWaitOnLogoff;
this.peakStartTime = defaults.peakStartTime;
this.peakStartVMOnConnect = defaults.peakStartVMOnConnect;
this.rampDownActionOnDisconnect = defaults.rampDownActionOnDisconnect;
this.rampDownActionOnLogoff = defaults.rampDownActionOnLogoff;
this.rampDownMinutesToWaitOnDisconnect = defaults.rampDownMinutesToWaitOnDisconnect;
this.rampDownMinutesToWaitOnLogoff = defaults.rampDownMinutesToWaitOnLogoff;
this.rampDownStartTime = defaults.rampDownStartTime;
this.rampDownStartVMOnConnect = defaults.rampDownStartVMOnConnect;
this.rampUpActionOnDisconnect = defaults.rampUpActionOnDisconnect;
this.rampUpActionOnLogoff = defaults.rampUpActionOnLogoff;
this.rampUpAutoStartHosts = defaults.rampUpAutoStartHosts;
this.rampUpMinutesToWaitOnDisconnect = defaults.rampUpMinutesToWaitOnDisconnect;
this.rampUpMinutesToWaitOnLogoff = defaults.rampUpMinutesToWaitOnLogoff;
this.rampUpStartTime = defaults.rampUpStartTime;
this.rampUpStartVMOnConnect = defaults.rampUpStartVMOnConnect;
this.systemData = defaults.systemData;
this.type = defaults.type;
}
@CustomType.Setter
public Builder daysOfWeek(@Nullable List daysOfWeek) {
this.daysOfWeek = daysOfWeek;
return this;
}
public Builder daysOfWeek(String... daysOfWeek) {
return daysOfWeek(List.of(daysOfWeek));
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetScalingPlanPersonalScheduleResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetScalingPlanPersonalScheduleResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder offPeakActionOnDisconnect(@Nullable String offPeakActionOnDisconnect) {
this.offPeakActionOnDisconnect = offPeakActionOnDisconnect;
return this;
}
@CustomType.Setter
public Builder offPeakActionOnLogoff(@Nullable String offPeakActionOnLogoff) {
this.offPeakActionOnLogoff = offPeakActionOnLogoff;
return this;
}
@CustomType.Setter
public Builder offPeakMinutesToWaitOnDisconnect(@Nullable Integer offPeakMinutesToWaitOnDisconnect) {
this.offPeakMinutesToWaitOnDisconnect = offPeakMinutesToWaitOnDisconnect;
return this;
}
@CustomType.Setter
public Builder offPeakMinutesToWaitOnLogoff(@Nullable Integer offPeakMinutesToWaitOnLogoff) {
this.offPeakMinutesToWaitOnLogoff = offPeakMinutesToWaitOnLogoff;
return this;
}
@CustomType.Setter
public Builder offPeakStartTime(@Nullable TimeResponse offPeakStartTime) {
this.offPeakStartTime = offPeakStartTime;
return this;
}
@CustomType.Setter
public Builder offPeakStartVMOnConnect(@Nullable String offPeakStartVMOnConnect) {
this.offPeakStartVMOnConnect = offPeakStartVMOnConnect;
return this;
}
@CustomType.Setter
public Builder peakActionOnDisconnect(@Nullable String peakActionOnDisconnect) {
this.peakActionOnDisconnect = peakActionOnDisconnect;
return this;
}
@CustomType.Setter
public Builder peakActionOnLogoff(@Nullable String peakActionOnLogoff) {
this.peakActionOnLogoff = peakActionOnLogoff;
return this;
}
@CustomType.Setter
public Builder peakMinutesToWaitOnDisconnect(@Nullable Integer peakMinutesToWaitOnDisconnect) {
this.peakMinutesToWaitOnDisconnect = peakMinutesToWaitOnDisconnect;
return this;
}
@CustomType.Setter
public Builder peakMinutesToWaitOnLogoff(@Nullable Integer peakMinutesToWaitOnLogoff) {
this.peakMinutesToWaitOnLogoff = peakMinutesToWaitOnLogoff;
return this;
}
@CustomType.Setter
public Builder peakStartTime(@Nullable TimeResponse peakStartTime) {
this.peakStartTime = peakStartTime;
return this;
}
@CustomType.Setter
public Builder peakStartVMOnConnect(@Nullable String peakStartVMOnConnect) {
this.peakStartVMOnConnect = peakStartVMOnConnect;
return this;
}
@CustomType.Setter
public Builder rampDownActionOnDisconnect(@Nullable String rampDownActionOnDisconnect) {
this.rampDownActionOnDisconnect = rampDownActionOnDisconnect;
return this;
}
@CustomType.Setter
public Builder rampDownActionOnLogoff(@Nullable String rampDownActionOnLogoff) {
this.rampDownActionOnLogoff = rampDownActionOnLogoff;
return this;
}
@CustomType.Setter
public Builder rampDownMinutesToWaitOnDisconnect(@Nullable Integer rampDownMinutesToWaitOnDisconnect) {
this.rampDownMinutesToWaitOnDisconnect = rampDownMinutesToWaitOnDisconnect;
return this;
}
@CustomType.Setter
public Builder rampDownMinutesToWaitOnLogoff(@Nullable Integer rampDownMinutesToWaitOnLogoff) {
this.rampDownMinutesToWaitOnLogoff = rampDownMinutesToWaitOnLogoff;
return this;
}
@CustomType.Setter
public Builder rampDownStartTime(@Nullable TimeResponse rampDownStartTime) {
this.rampDownStartTime = rampDownStartTime;
return this;
}
@CustomType.Setter
public Builder rampDownStartVMOnConnect(@Nullable String rampDownStartVMOnConnect) {
this.rampDownStartVMOnConnect = rampDownStartVMOnConnect;
return this;
}
@CustomType.Setter
public Builder rampUpActionOnDisconnect(@Nullable String rampUpActionOnDisconnect) {
this.rampUpActionOnDisconnect = rampUpActionOnDisconnect;
return this;
}
@CustomType.Setter
public Builder rampUpActionOnLogoff(@Nullable String rampUpActionOnLogoff) {
this.rampUpActionOnLogoff = rampUpActionOnLogoff;
return this;
}
@CustomType.Setter
public Builder rampUpAutoStartHosts(@Nullable String rampUpAutoStartHosts) {
this.rampUpAutoStartHosts = rampUpAutoStartHosts;
return this;
}
@CustomType.Setter
public Builder rampUpMinutesToWaitOnDisconnect(@Nullable Integer rampUpMinutesToWaitOnDisconnect) {
this.rampUpMinutesToWaitOnDisconnect = rampUpMinutesToWaitOnDisconnect;
return this;
}
@CustomType.Setter
public Builder rampUpMinutesToWaitOnLogoff(@Nullable Integer rampUpMinutesToWaitOnLogoff) {
this.rampUpMinutesToWaitOnLogoff = rampUpMinutesToWaitOnLogoff;
return this;
}
@CustomType.Setter
public Builder rampUpStartTime(@Nullable TimeResponse rampUpStartTime) {
this.rampUpStartTime = rampUpStartTime;
return this;
}
@CustomType.Setter
public Builder rampUpStartVMOnConnect(@Nullable String rampUpStartVMOnConnect) {
this.rampUpStartVMOnConnect = rampUpStartVMOnConnect;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetScalingPlanPersonalScheduleResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetScalingPlanPersonalScheduleResult", "type");
}
this.type = type;
return this;
}
public GetScalingPlanPersonalScheduleResult build() {
final var _resultValue = new GetScalingPlanPersonalScheduleResult();
_resultValue.daysOfWeek = daysOfWeek;
_resultValue.id = id;
_resultValue.name = name;
_resultValue.offPeakActionOnDisconnect = offPeakActionOnDisconnect;
_resultValue.offPeakActionOnLogoff = offPeakActionOnLogoff;
_resultValue.offPeakMinutesToWaitOnDisconnect = offPeakMinutesToWaitOnDisconnect;
_resultValue.offPeakMinutesToWaitOnLogoff = offPeakMinutesToWaitOnLogoff;
_resultValue.offPeakStartTime = offPeakStartTime;
_resultValue.offPeakStartVMOnConnect = offPeakStartVMOnConnect;
_resultValue.peakActionOnDisconnect = peakActionOnDisconnect;
_resultValue.peakActionOnLogoff = peakActionOnLogoff;
_resultValue.peakMinutesToWaitOnDisconnect = peakMinutesToWaitOnDisconnect;
_resultValue.peakMinutesToWaitOnLogoff = peakMinutesToWaitOnLogoff;
_resultValue.peakStartTime = peakStartTime;
_resultValue.peakStartVMOnConnect = peakStartVMOnConnect;
_resultValue.rampDownActionOnDisconnect = rampDownActionOnDisconnect;
_resultValue.rampDownActionOnLogoff = rampDownActionOnLogoff;
_resultValue.rampDownMinutesToWaitOnDisconnect = rampDownMinutesToWaitOnDisconnect;
_resultValue.rampDownMinutesToWaitOnLogoff = rampDownMinutesToWaitOnLogoff;
_resultValue.rampDownStartTime = rampDownStartTime;
_resultValue.rampDownStartVMOnConnect = rampDownStartVMOnConnect;
_resultValue.rampUpActionOnDisconnect = rampUpActionOnDisconnect;
_resultValue.rampUpActionOnLogoff = rampUpActionOnLogoff;
_resultValue.rampUpAutoStartHosts = rampUpAutoStartHosts;
_resultValue.rampUpMinutesToWaitOnDisconnect = rampUpMinutesToWaitOnDisconnect;
_resultValue.rampUpMinutesToWaitOnLogoff = rampUpMinutesToWaitOnLogoff;
_resultValue.rampUpStartTime = rampUpStartTime;
_resultValue.rampUpStartVMOnConnect = rampUpStartVMOnConnect;
_resultValue.systemData = systemData;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy