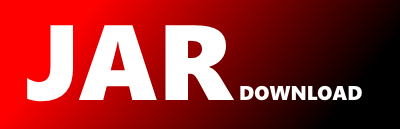
com.pulumi.azurenative.devhub.DevhubFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.devhub;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.devhub.inputs.GetADOOAuthInfoArgs;
import com.pulumi.azurenative.devhub.inputs.GetADOOAuthInfoPlainArgs;
import com.pulumi.azurenative.devhub.inputs.GetGitHubOAuthArgs;
import com.pulumi.azurenative.devhub.inputs.GetGitHubOAuthPlainArgs;
import com.pulumi.azurenative.devhub.inputs.GetIacProfileArgs;
import com.pulumi.azurenative.devhub.inputs.GetIacProfilePlainArgs;
import com.pulumi.azurenative.devhub.inputs.GetWorkflowArgs;
import com.pulumi.azurenative.devhub.inputs.GetWorkflowPlainArgs;
import com.pulumi.azurenative.devhub.outputs.GetADOOAuthInfoResult;
import com.pulumi.azurenative.devhub.outputs.GetGitHubOAuthResult;
import com.pulumi.azurenative.devhub.outputs.GetIacProfileResult;
import com.pulumi.azurenative.devhub.outputs.GetWorkflowResult;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import java.util.concurrent.CompletableFuture;
public final class DevhubFunctions {
/**
* Response containing ADO OAuth information
* Azure REST API version: 2024-08-01-preview.
*
*/
public static Output getADOOAuthInfo(GetADOOAuthInfoArgs args) {
return getADOOAuthInfo(args, InvokeOptions.Empty);
}
/**
* Response containing ADO OAuth information
* Azure REST API version: 2024-08-01-preview.
*
*/
public static CompletableFuture getADOOAuthInfoPlain(GetADOOAuthInfoPlainArgs args) {
return getADOOAuthInfoPlain(args, InvokeOptions.Empty);
}
/**
* Response containing ADO OAuth information
* Azure REST API version: 2024-08-01-preview.
*
*/
public static Output getADOOAuthInfo(GetADOOAuthInfoArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:devhub:getADOOAuthInfo", TypeShape.of(GetADOOAuthInfoResult.class), args, Utilities.withVersion(options));
}
/**
* Response containing ADO OAuth information
* Azure REST API version: 2024-08-01-preview.
*
*/
public static CompletableFuture getADOOAuthInfoPlain(GetADOOAuthInfoPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:devhub:getADOOAuthInfo", TypeShape.of(GetADOOAuthInfoResult.class), args, Utilities.withVersion(options));
}
/**
* URL used to authorize the Developer Hub GitHub App
* Azure REST API version: 2022-10-11-preview.
*
* Other available API versions: 2022-04-01-preview, 2023-08-01, 2024-05-01-preview, 2024-08-01-preview.
*
*/
public static Output getGitHubOAuth(GetGitHubOAuthArgs args) {
return getGitHubOAuth(args, InvokeOptions.Empty);
}
/**
* URL used to authorize the Developer Hub GitHub App
* Azure REST API version: 2022-10-11-preview.
*
* Other available API versions: 2022-04-01-preview, 2023-08-01, 2024-05-01-preview, 2024-08-01-preview.
*
*/
public static CompletableFuture getGitHubOAuthPlain(GetGitHubOAuthPlainArgs args) {
return getGitHubOAuthPlain(args, InvokeOptions.Empty);
}
/**
* URL used to authorize the Developer Hub GitHub App
* Azure REST API version: 2022-10-11-preview.
*
* Other available API versions: 2022-04-01-preview, 2023-08-01, 2024-05-01-preview, 2024-08-01-preview.
*
*/
public static Output getGitHubOAuth(GetGitHubOAuthArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:devhub:getGitHubOAuth", TypeShape.of(GetGitHubOAuthResult.class), args, Utilities.withVersion(options));
}
/**
* URL used to authorize the Developer Hub GitHub App
* Azure REST API version: 2022-10-11-preview.
*
* Other available API versions: 2022-04-01-preview, 2023-08-01, 2024-05-01-preview, 2024-08-01-preview.
*
*/
public static CompletableFuture getGitHubOAuthPlain(GetGitHubOAuthPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:devhub:getGitHubOAuth", TypeShape.of(GetGitHubOAuthResult.class), args, Utilities.withVersion(options));
}
/**
* Resource representation of a IacProfile.
* Azure REST API version: 2024-05-01-preview.
*
* Other available API versions: 2024-08-01-preview.
*
*/
public static Output getIacProfile(GetIacProfileArgs args) {
return getIacProfile(args, InvokeOptions.Empty);
}
/**
* Resource representation of a IacProfile.
* Azure REST API version: 2024-05-01-preview.
*
* Other available API versions: 2024-08-01-preview.
*
*/
public static CompletableFuture getIacProfilePlain(GetIacProfilePlainArgs args) {
return getIacProfilePlain(args, InvokeOptions.Empty);
}
/**
* Resource representation of a IacProfile.
* Azure REST API version: 2024-05-01-preview.
*
* Other available API versions: 2024-08-01-preview.
*
*/
public static Output getIacProfile(GetIacProfileArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:devhub:getIacProfile", TypeShape.of(GetIacProfileResult.class), args, Utilities.withVersion(options));
}
/**
* Resource representation of a IacProfile.
* Azure REST API version: 2024-05-01-preview.
*
* Other available API versions: 2024-08-01-preview.
*
*/
public static CompletableFuture getIacProfilePlain(GetIacProfilePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:devhub:getIacProfile", TypeShape.of(GetIacProfileResult.class), args, Utilities.withVersion(options));
}
/**
* Resource representation of a workflow
* Azure REST API version: 2022-10-11-preview.
*
* Other available API versions: 2023-08-01, 2024-05-01-preview, 2024-08-01-preview.
*
*/
public static Output getWorkflow(GetWorkflowArgs args) {
return getWorkflow(args, InvokeOptions.Empty);
}
/**
* Resource representation of a workflow
* Azure REST API version: 2022-10-11-preview.
*
* Other available API versions: 2023-08-01, 2024-05-01-preview, 2024-08-01-preview.
*
*/
public static CompletableFuture getWorkflowPlain(GetWorkflowPlainArgs args) {
return getWorkflowPlain(args, InvokeOptions.Empty);
}
/**
* Resource representation of a workflow
* Azure REST API version: 2022-10-11-preview.
*
* Other available API versions: 2023-08-01, 2024-05-01-preview, 2024-08-01-preview.
*
*/
public static Output getWorkflow(GetWorkflowArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:devhub:getWorkflow", TypeShape.of(GetWorkflowResult.class), args, Utilities.withVersion(options));
}
/**
* Resource representation of a workflow
* Azure REST API version: 2022-10-11-preview.
*
* Other available API versions: 2023-08-01, 2024-05-01-preview, 2024-08-01-preview.
*
*/
public static CompletableFuture getWorkflowPlain(GetWorkflowPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:devhub:getWorkflow", TypeShape.of(GetWorkflowResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy