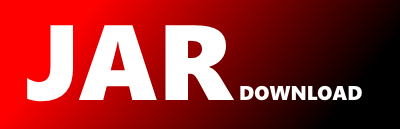
com.pulumi.azurenative.deviceregistry.outputs.EventResponse Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.deviceregistry.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class EventResponse {
/**
* @return The path to the type definition of the capability (e.g. DTMI, OPC UA information model node id, etc.), for example dtmi:com:example:Robot:_contents:__prop1;1.
*
*/
private @Nullable String capabilityId;
/**
* @return Stringified JSON that contains connector-specific configuration for the event. For OPC UA, this could include configuration like, publishingInterval, samplingInterval, and queueSize.
*
*/
private @Nullable String eventConfiguration;
/**
* @return The address of the notifier of the event in the asset (e.g. URL) so that a client can access the event on the asset.
*
*/
private String eventNotifier;
/**
* @return The name of the event.
*
*/
private @Nullable String name;
/**
* @return An indication of how the event should be mapped to OpenTelemetry.
*
*/
private @Nullable String observabilityMode;
private EventResponse() {}
/**
* @return The path to the type definition of the capability (e.g. DTMI, OPC UA information model node id, etc.), for example dtmi:com:example:Robot:_contents:__prop1;1.
*
*/
public Optional capabilityId() {
return Optional.ofNullable(this.capabilityId);
}
/**
* @return Stringified JSON that contains connector-specific configuration for the event. For OPC UA, this could include configuration like, publishingInterval, samplingInterval, and queueSize.
*
*/
public Optional eventConfiguration() {
return Optional.ofNullable(this.eventConfiguration);
}
/**
* @return The address of the notifier of the event in the asset (e.g. URL) so that a client can access the event on the asset.
*
*/
public String eventNotifier() {
return this.eventNotifier;
}
/**
* @return The name of the event.
*
*/
public Optional name() {
return Optional.ofNullable(this.name);
}
/**
* @return An indication of how the event should be mapped to OpenTelemetry.
*
*/
public Optional observabilityMode() {
return Optional.ofNullable(this.observabilityMode);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(EventResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String capabilityId;
private @Nullable String eventConfiguration;
private String eventNotifier;
private @Nullable String name;
private @Nullable String observabilityMode;
public Builder() {}
public Builder(EventResponse defaults) {
Objects.requireNonNull(defaults);
this.capabilityId = defaults.capabilityId;
this.eventConfiguration = defaults.eventConfiguration;
this.eventNotifier = defaults.eventNotifier;
this.name = defaults.name;
this.observabilityMode = defaults.observabilityMode;
}
@CustomType.Setter
public Builder capabilityId(@Nullable String capabilityId) {
this.capabilityId = capabilityId;
return this;
}
@CustomType.Setter
public Builder eventConfiguration(@Nullable String eventConfiguration) {
this.eventConfiguration = eventConfiguration;
return this;
}
@CustomType.Setter
public Builder eventNotifier(String eventNotifier) {
if (eventNotifier == null) {
throw new MissingRequiredPropertyException("EventResponse", "eventNotifier");
}
this.eventNotifier = eventNotifier;
return this;
}
@CustomType.Setter
public Builder name(@Nullable String name) {
this.name = name;
return this;
}
@CustomType.Setter
public Builder observabilityMode(@Nullable String observabilityMode) {
this.observabilityMode = observabilityMode;
return this;
}
public EventResponse build() {
final var _resultValue = new EventResponse();
_resultValue.capabilityId = capabilityId;
_resultValue.eventConfiguration = eventConfiguration;
_resultValue.eventNotifier = eventNotifier;
_resultValue.name = name;
_resultValue.observabilityMode = observabilityMode;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy