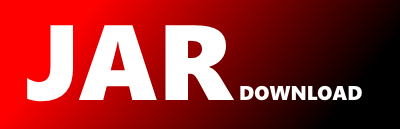
com.pulumi.azurenative.deviceregistry.outputs.GetAssetResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.deviceregistry.outputs;
import com.pulumi.azurenative.deviceregistry.outputs.AssetStatusResponse;
import com.pulumi.azurenative.deviceregistry.outputs.DataPointResponse;
import com.pulumi.azurenative.deviceregistry.outputs.EventResponse;
import com.pulumi.azurenative.deviceregistry.outputs.ExtendedLocationResponse;
import com.pulumi.azurenative.deviceregistry.outputs.SystemDataResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.Object;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetAssetResult {
/**
* @return A reference to the asset endpoint profile (connection information) used by brokers to connect to an endpoint that provides data points for this asset. Must have the format <ModuleCR.metadata.namespace>/<ModuleCR.metadata.name>.
*
*/
private String assetEndpointProfileUri;
/**
* @return Resource path to asset type (model) definition.
*
*/
private @Nullable String assetType;
/**
* @return A set of key-value pairs that contain custom attributes set by the customer.
*
*/
private @Nullable Object attributes;
/**
* @return Array of data points that are part of the asset. Each data point can reference an asset type capability and have per-data point configuration.
*
*/
private @Nullable List dataPoints;
/**
* @return Stringified JSON that contains protocol-specific default configuration for all data points. Each data point can have its own configuration that overrides the default settings here.
*
*/
private @Nullable String defaultDataPointsConfiguration;
/**
* @return Stringified JSON that contains connector-specific default configuration for all events. Each event can have its own configuration that overrides the default settings here.
*
*/
private @Nullable String defaultEventsConfiguration;
/**
* @return Human-readable description of the asset.
*
*/
private @Nullable String description;
/**
* @return Human-readable display name.
*
*/
private @Nullable String displayName;
/**
* @return Reference to the documentation.
*
*/
private @Nullable String documentationUri;
/**
* @return Enabled/Disabled status of the asset.
*
*/
private @Nullable Boolean enabled;
/**
* @return Array of events that are part of the asset. Each event can have per-event configuration.
*
*/
private @Nullable List events;
/**
* @return The extended location.
*
*/
private ExtendedLocationResponse extendedLocation;
/**
* @return Asset id provided by the customer.
*
*/
private @Nullable String externalAssetId;
/**
* @return Revision number of the hardware.
*
*/
private @Nullable String hardwareRevision;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return The geo-location where the resource lives
*
*/
private String location;
/**
* @return Asset manufacturer name.
*
*/
private @Nullable String manufacturer;
/**
* @return Asset manufacturer URI.
*
*/
private @Nullable String manufacturerUri;
/**
* @return Asset model name.
*
*/
private @Nullable String model;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return Asset product code.
*
*/
private @Nullable String productCode;
/**
* @return Provisioning state of the resource.
*
*/
private String provisioningState;
/**
* @return Asset serial number.
*
*/
private @Nullable String serialNumber;
/**
* @return Revision number of the software.
*
*/
private @Nullable String softwareRevision;
/**
* @return Read only object to reflect changes that have occurred on the Edge. Similar to Kubernetes status property for custom resources.
*
*/
private AssetStatusResponse status;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
private SystemDataResponse systemData;
/**
* @return Resource tags.
*
*/
private @Nullable Map tags;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
/**
* @return Globally unique, immutable, non-reusable id.
*
*/
private String uuid;
/**
* @return An integer that is incremented each time the resource is modified.
*
*/
private Integer version;
private GetAssetResult() {}
/**
* @return A reference to the asset endpoint profile (connection information) used by brokers to connect to an endpoint that provides data points for this asset. Must have the format <ModuleCR.metadata.namespace>/<ModuleCR.metadata.name>.
*
*/
public String assetEndpointProfileUri() {
return this.assetEndpointProfileUri;
}
/**
* @return Resource path to asset type (model) definition.
*
*/
public Optional assetType() {
return Optional.ofNullable(this.assetType);
}
/**
* @return A set of key-value pairs that contain custom attributes set by the customer.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy