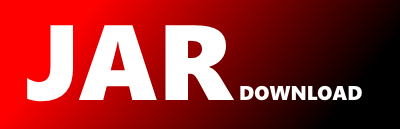
com.pulumi.azurenative.devices.inputs.RoutingEndpointsArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.devices.inputs;
import com.pulumi.azurenative.devices.inputs.RoutingCosmosDBSqlApiPropertiesArgs;
import com.pulumi.azurenative.devices.inputs.RoutingEventHubPropertiesArgs;
import com.pulumi.azurenative.devices.inputs.RoutingServiceBusQueueEndpointPropertiesArgs;
import com.pulumi.azurenative.devices.inputs.RoutingServiceBusTopicEndpointPropertiesArgs;
import com.pulumi.azurenative.devices.inputs.RoutingStorageContainerPropertiesArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* The properties related to the custom endpoints to which your IoT hub routes messages based on the routing rules. A maximum of 10 custom endpoints are allowed across all endpoint types for paid hubs and only 1 custom endpoint is allowed across all endpoint types for free hubs.
*
*/
public final class RoutingEndpointsArgs extends com.pulumi.resources.ResourceArgs {
public static final RoutingEndpointsArgs Empty = new RoutingEndpointsArgs();
/**
* The list of Cosmos DB collection endpoints that IoT hub routes messages to, based on the routing rules.
*
*/
@Import(name="cosmosDBSqlCollections")
private @Nullable Output> cosmosDBSqlCollections;
/**
* @return The list of Cosmos DB collection endpoints that IoT hub routes messages to, based on the routing rules.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy