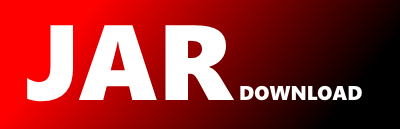
com.pulumi.azurenative.devices.inputs.RoutingPropertiesArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.devices.inputs;
import com.pulumi.azurenative.devices.inputs.EnrichmentPropertiesArgs;
import com.pulumi.azurenative.devices.inputs.FallbackRoutePropertiesArgs;
import com.pulumi.azurenative.devices.inputs.RoutePropertiesArgs;
import com.pulumi.azurenative.devices.inputs.RoutingEndpointsArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* The routing related properties of the IoT hub. See: https://docs.microsoft.com/azure/iot-hub/iot-hub-devguide-messaging
*
*/
public final class RoutingPropertiesArgs extends com.pulumi.resources.ResourceArgs {
public static final RoutingPropertiesArgs Empty = new RoutingPropertiesArgs();
/**
* The properties related to the custom endpoints to which your IoT hub routes messages based on the routing rules. A maximum of 10 custom endpoints are allowed across all endpoint types for paid hubs and only 1 custom endpoint is allowed across all endpoint types for free hubs.
*
*/
@Import(name="endpoints")
private @Nullable Output endpoints;
/**
* @return The properties related to the custom endpoints to which your IoT hub routes messages based on the routing rules. A maximum of 10 custom endpoints are allowed across all endpoint types for paid hubs and only 1 custom endpoint is allowed across all endpoint types for free hubs.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy