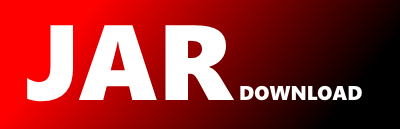
com.pulumi.azurenative.devices.outputs.RoutingEndpointsResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.devices.outputs;
import com.pulumi.azurenative.devices.outputs.RoutingCosmosDBSqlApiPropertiesResponse;
import com.pulumi.azurenative.devices.outputs.RoutingEventHubPropertiesResponse;
import com.pulumi.azurenative.devices.outputs.RoutingServiceBusQueueEndpointPropertiesResponse;
import com.pulumi.azurenative.devices.outputs.RoutingServiceBusTopicEndpointPropertiesResponse;
import com.pulumi.azurenative.devices.outputs.RoutingStorageContainerPropertiesResponse;
import com.pulumi.core.annotations.CustomType;
import java.util.List;
import java.util.Objects;
import javax.annotation.Nullable;
@CustomType
public final class RoutingEndpointsResponse {
/**
* @return The list of Cosmos DB collection endpoints that IoT hub routes messages to, based on the routing rules.
*
*/
private @Nullable List cosmosDBSqlCollections;
/**
* @return The list of Event Hubs endpoints that IoT hub routes messages to, based on the routing rules. This list does not include the built-in Event Hubs endpoint.
*
*/
private @Nullable List eventHubs;
/**
* @return The list of Service Bus queue endpoints that IoT hub routes the messages to, based on the routing rules.
*
*/
private @Nullable List serviceBusQueues;
/**
* @return The list of Service Bus topic endpoints that the IoT hub routes the messages to, based on the routing rules.
*
*/
private @Nullable List serviceBusTopics;
/**
* @return The list of storage container endpoints that IoT hub routes messages to, based on the routing rules.
*
*/
private @Nullable List storageContainers;
private RoutingEndpointsResponse() {}
/**
* @return The list of Cosmos DB collection endpoints that IoT hub routes messages to, based on the routing rules.
*
*/
public List cosmosDBSqlCollections() {
return this.cosmosDBSqlCollections == null ? List.of() : this.cosmosDBSqlCollections;
}
/**
* @return The list of Event Hubs endpoints that IoT hub routes messages to, based on the routing rules. This list does not include the built-in Event Hubs endpoint.
*
*/
public List eventHubs() {
return this.eventHubs == null ? List.of() : this.eventHubs;
}
/**
* @return The list of Service Bus queue endpoints that IoT hub routes the messages to, based on the routing rules.
*
*/
public List serviceBusQueues() {
return this.serviceBusQueues == null ? List.of() : this.serviceBusQueues;
}
/**
* @return The list of Service Bus topic endpoints that the IoT hub routes the messages to, based on the routing rules.
*
*/
public List serviceBusTopics() {
return this.serviceBusTopics == null ? List.of() : this.serviceBusTopics;
}
/**
* @return The list of storage container endpoints that IoT hub routes messages to, based on the routing rules.
*
*/
public List storageContainers() {
return this.storageContainers == null ? List.of() : this.storageContainers;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(RoutingEndpointsResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List cosmosDBSqlCollections;
private @Nullable List eventHubs;
private @Nullable List serviceBusQueues;
private @Nullable List serviceBusTopics;
private @Nullable List storageContainers;
public Builder() {}
public Builder(RoutingEndpointsResponse defaults) {
Objects.requireNonNull(defaults);
this.cosmosDBSqlCollections = defaults.cosmosDBSqlCollections;
this.eventHubs = defaults.eventHubs;
this.serviceBusQueues = defaults.serviceBusQueues;
this.serviceBusTopics = defaults.serviceBusTopics;
this.storageContainers = defaults.storageContainers;
}
@CustomType.Setter
public Builder cosmosDBSqlCollections(@Nullable List cosmosDBSqlCollections) {
this.cosmosDBSqlCollections = cosmosDBSqlCollections;
return this;
}
public Builder cosmosDBSqlCollections(RoutingCosmosDBSqlApiPropertiesResponse... cosmosDBSqlCollections) {
return cosmosDBSqlCollections(List.of(cosmosDBSqlCollections));
}
@CustomType.Setter
public Builder eventHubs(@Nullable List eventHubs) {
this.eventHubs = eventHubs;
return this;
}
public Builder eventHubs(RoutingEventHubPropertiesResponse... eventHubs) {
return eventHubs(List.of(eventHubs));
}
@CustomType.Setter
public Builder serviceBusQueues(@Nullable List serviceBusQueues) {
this.serviceBusQueues = serviceBusQueues;
return this;
}
public Builder serviceBusQueues(RoutingServiceBusQueueEndpointPropertiesResponse... serviceBusQueues) {
return serviceBusQueues(List.of(serviceBusQueues));
}
@CustomType.Setter
public Builder serviceBusTopics(@Nullable List serviceBusTopics) {
this.serviceBusTopics = serviceBusTopics;
return this;
}
public Builder serviceBusTopics(RoutingServiceBusTopicEndpointPropertiesResponse... serviceBusTopics) {
return serviceBusTopics(List.of(serviceBusTopics));
}
@CustomType.Setter
public Builder storageContainers(@Nullable List storageContainers) {
this.storageContainers = storageContainers;
return this;
}
public Builder storageContainers(RoutingStorageContainerPropertiesResponse... storageContainers) {
return storageContainers(List.of(storageContainers));
}
public RoutingEndpointsResponse build() {
final var _resultValue = new RoutingEndpointsResponse();
_resultValue.cosmosDBSqlCollections = cosmosDBSqlCollections;
_resultValue.eventHubs = eventHubs;
_resultValue.serviceBusQueues = serviceBusQueues;
_resultValue.serviceBusTopics = serviceBusTopics;
_resultValue.storageContainers = storageContainers;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy