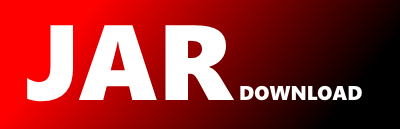
com.pulumi.azurenative.devtestlab.outputs.GetLabResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.devtestlab.outputs;
import com.pulumi.azurenative.devtestlab.outputs.LabAnnouncementPropertiesResponse;
import com.pulumi.azurenative.devtestlab.outputs.LabSupportPropertiesResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetLabResult {
/**
* @return The properties of any lab announcement associated with this lab
*
*/
private @Nullable LabAnnouncementPropertiesResponse announcement;
/**
* @return The lab's artifact storage account.
*
*/
private String artifactsStorageAccount;
/**
* @return The creation date of the lab.
*
*/
private String createdDate;
/**
* @return The lab's default premium storage account.
*
*/
private String defaultPremiumStorageAccount;
/**
* @return The lab's default storage account.
*
*/
private String defaultStorageAccount;
/**
* @return The access rights to be granted to the user when provisioning an environment
*
*/
private @Nullable String environmentPermission;
/**
* @return Extended properties of the lab used for experimental features
*
*/
private @Nullable Map extendedProperties;
/**
* @return The identifier of the resource.
*
*/
private String id;
/**
* @return Type of storage used by the lab. It can be either Premium or Standard. Default is Premium.
*
*/
private @Nullable String labStorageType;
/**
* @return The load balancer used to for lab VMs that use shared IP address.
*
*/
private String loadBalancerId;
/**
* @return The location of the resource.
*
*/
private @Nullable String location;
/**
* @return The ordered list of artifact resource IDs that should be applied on all Linux VM creations by default, prior to the artifacts specified by the user.
*
*/
private @Nullable List mandatoryArtifactsResourceIdsLinux;
/**
* @return The ordered list of artifact resource IDs that should be applied on all Windows VM creations by default, prior to the artifacts specified by the user.
*
*/
private @Nullable List mandatoryArtifactsResourceIdsWindows;
/**
* @return The name of the resource.
*
*/
private String name;
/**
* @return The Network Security Group attached to the lab VMs Network interfaces to restrict open ports.
*
*/
private String networkSecurityGroupId;
/**
* @return The lab's premium data disk storage account.
*
*/
private String premiumDataDiskStorageAccount;
/**
* @return The setting to enable usage of premium data disks.
* When its value is 'Enabled', creation of standard or premium data disks is allowed.
* When its value is 'Disabled', only creation of standard data disks is allowed.
*
*/
private @Nullable String premiumDataDisks;
/**
* @return The provisioning status of the resource.
*
*/
private String provisioningState;
/**
* @return The public IP address for the lab's load balancer.
*
*/
private String publicIpId;
/**
* @return The properties of any lab support message associated with this lab
*
*/
private @Nullable LabSupportPropertiesResponse support;
/**
* @return The tags of the resource.
*
*/
private @Nullable Map tags;
/**
* @return The type of the resource.
*
*/
private String type;
/**
* @return The unique immutable identifier of a resource (Guid).
*
*/
private String uniqueIdentifier;
/**
* @return The lab's Key vault.
*
*/
private String vaultName;
/**
* @return The resource group in which all new lab virtual machines will be created. To let DevTest Labs manage resource group creation, set this value to null.
*
*/
private String vmCreationResourceGroup;
private GetLabResult() {}
/**
* @return The properties of any lab announcement associated with this lab
*
*/
public Optional announcement() {
return Optional.ofNullable(this.announcement);
}
/**
* @return The lab's artifact storage account.
*
*/
public String artifactsStorageAccount() {
return this.artifactsStorageAccount;
}
/**
* @return The creation date of the lab.
*
*/
public String createdDate() {
return this.createdDate;
}
/**
* @return The lab's default premium storage account.
*
*/
public String defaultPremiumStorageAccount() {
return this.defaultPremiumStorageAccount;
}
/**
* @return The lab's default storage account.
*
*/
public String defaultStorageAccount() {
return this.defaultStorageAccount;
}
/**
* @return The access rights to be granted to the user when provisioning an environment
*
*/
public Optional environmentPermission() {
return Optional.ofNullable(this.environmentPermission);
}
/**
* @return Extended properties of the lab used for experimental features
*
*/
public Map extendedProperties() {
return this.extendedProperties == null ? Map.of() : this.extendedProperties;
}
/**
* @return The identifier of the resource.
*
*/
public String id() {
return this.id;
}
/**
* @return Type of storage used by the lab. It can be either Premium or Standard. Default is Premium.
*
*/
public Optional labStorageType() {
return Optional.ofNullable(this.labStorageType);
}
/**
* @return The load balancer used to for lab VMs that use shared IP address.
*
*/
public String loadBalancerId() {
return this.loadBalancerId;
}
/**
* @return The location of the resource.
*
*/
public Optional location() {
return Optional.ofNullable(this.location);
}
/**
* @return The ordered list of artifact resource IDs that should be applied on all Linux VM creations by default, prior to the artifacts specified by the user.
*
*/
public List mandatoryArtifactsResourceIdsLinux() {
return this.mandatoryArtifactsResourceIdsLinux == null ? List.of() : this.mandatoryArtifactsResourceIdsLinux;
}
/**
* @return The ordered list of artifact resource IDs that should be applied on all Windows VM creations by default, prior to the artifacts specified by the user.
*
*/
public List mandatoryArtifactsResourceIdsWindows() {
return this.mandatoryArtifactsResourceIdsWindows == null ? List.of() : this.mandatoryArtifactsResourceIdsWindows;
}
/**
* @return The name of the resource.
*
*/
public String name() {
return this.name;
}
/**
* @return The Network Security Group attached to the lab VMs Network interfaces to restrict open ports.
*
*/
public String networkSecurityGroupId() {
return this.networkSecurityGroupId;
}
/**
* @return The lab's premium data disk storage account.
*
*/
public String premiumDataDiskStorageAccount() {
return this.premiumDataDiskStorageAccount;
}
/**
* @return The setting to enable usage of premium data disks.
* When its value is 'Enabled', creation of standard or premium data disks is allowed.
* When its value is 'Disabled', only creation of standard data disks is allowed.
*
*/
public Optional premiumDataDisks() {
return Optional.ofNullable(this.premiumDataDisks);
}
/**
* @return The provisioning status of the resource.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return The public IP address for the lab's load balancer.
*
*/
public String publicIpId() {
return this.publicIpId;
}
/**
* @return The properties of any lab support message associated with this lab
*
*/
public Optional support() {
return Optional.ofNullable(this.support);
}
/**
* @return The tags of the resource.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return The type of the resource.
*
*/
public String type() {
return this.type;
}
/**
* @return The unique immutable identifier of a resource (Guid).
*
*/
public String uniqueIdentifier() {
return this.uniqueIdentifier;
}
/**
* @return The lab's Key vault.
*
*/
public String vaultName() {
return this.vaultName;
}
/**
* @return The resource group in which all new lab virtual machines will be created. To let DevTest Labs manage resource group creation, set this value to null.
*
*/
public String vmCreationResourceGroup() {
return this.vmCreationResourceGroup;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetLabResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable LabAnnouncementPropertiesResponse announcement;
private String artifactsStorageAccount;
private String createdDate;
private String defaultPremiumStorageAccount;
private String defaultStorageAccount;
private @Nullable String environmentPermission;
private @Nullable Map extendedProperties;
private String id;
private @Nullable String labStorageType;
private String loadBalancerId;
private @Nullable String location;
private @Nullable List mandatoryArtifactsResourceIdsLinux;
private @Nullable List mandatoryArtifactsResourceIdsWindows;
private String name;
private String networkSecurityGroupId;
private String premiumDataDiskStorageAccount;
private @Nullable String premiumDataDisks;
private String provisioningState;
private String publicIpId;
private @Nullable LabSupportPropertiesResponse support;
private @Nullable Map tags;
private String type;
private String uniqueIdentifier;
private String vaultName;
private String vmCreationResourceGroup;
public Builder() {}
public Builder(GetLabResult defaults) {
Objects.requireNonNull(defaults);
this.announcement = defaults.announcement;
this.artifactsStorageAccount = defaults.artifactsStorageAccount;
this.createdDate = defaults.createdDate;
this.defaultPremiumStorageAccount = defaults.defaultPremiumStorageAccount;
this.defaultStorageAccount = defaults.defaultStorageAccount;
this.environmentPermission = defaults.environmentPermission;
this.extendedProperties = defaults.extendedProperties;
this.id = defaults.id;
this.labStorageType = defaults.labStorageType;
this.loadBalancerId = defaults.loadBalancerId;
this.location = defaults.location;
this.mandatoryArtifactsResourceIdsLinux = defaults.mandatoryArtifactsResourceIdsLinux;
this.mandatoryArtifactsResourceIdsWindows = defaults.mandatoryArtifactsResourceIdsWindows;
this.name = defaults.name;
this.networkSecurityGroupId = defaults.networkSecurityGroupId;
this.premiumDataDiskStorageAccount = defaults.premiumDataDiskStorageAccount;
this.premiumDataDisks = defaults.premiumDataDisks;
this.provisioningState = defaults.provisioningState;
this.publicIpId = defaults.publicIpId;
this.support = defaults.support;
this.tags = defaults.tags;
this.type = defaults.type;
this.uniqueIdentifier = defaults.uniqueIdentifier;
this.vaultName = defaults.vaultName;
this.vmCreationResourceGroup = defaults.vmCreationResourceGroup;
}
@CustomType.Setter
public Builder announcement(@Nullable LabAnnouncementPropertiesResponse announcement) {
this.announcement = announcement;
return this;
}
@CustomType.Setter
public Builder artifactsStorageAccount(String artifactsStorageAccount) {
if (artifactsStorageAccount == null) {
throw new MissingRequiredPropertyException("GetLabResult", "artifactsStorageAccount");
}
this.artifactsStorageAccount = artifactsStorageAccount;
return this;
}
@CustomType.Setter
public Builder createdDate(String createdDate) {
if (createdDate == null) {
throw new MissingRequiredPropertyException("GetLabResult", "createdDate");
}
this.createdDate = createdDate;
return this;
}
@CustomType.Setter
public Builder defaultPremiumStorageAccount(String defaultPremiumStorageAccount) {
if (defaultPremiumStorageAccount == null) {
throw new MissingRequiredPropertyException("GetLabResult", "defaultPremiumStorageAccount");
}
this.defaultPremiumStorageAccount = defaultPremiumStorageAccount;
return this;
}
@CustomType.Setter
public Builder defaultStorageAccount(String defaultStorageAccount) {
if (defaultStorageAccount == null) {
throw new MissingRequiredPropertyException("GetLabResult", "defaultStorageAccount");
}
this.defaultStorageAccount = defaultStorageAccount;
return this;
}
@CustomType.Setter
public Builder environmentPermission(@Nullable String environmentPermission) {
this.environmentPermission = environmentPermission;
return this;
}
@CustomType.Setter
public Builder extendedProperties(@Nullable Map extendedProperties) {
this.extendedProperties = extendedProperties;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetLabResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder labStorageType(@Nullable String labStorageType) {
this.labStorageType = labStorageType;
return this;
}
@CustomType.Setter
public Builder loadBalancerId(String loadBalancerId) {
if (loadBalancerId == null) {
throw new MissingRequiredPropertyException("GetLabResult", "loadBalancerId");
}
this.loadBalancerId = loadBalancerId;
return this;
}
@CustomType.Setter
public Builder location(@Nullable String location) {
this.location = location;
return this;
}
@CustomType.Setter
public Builder mandatoryArtifactsResourceIdsLinux(@Nullable List mandatoryArtifactsResourceIdsLinux) {
this.mandatoryArtifactsResourceIdsLinux = mandatoryArtifactsResourceIdsLinux;
return this;
}
public Builder mandatoryArtifactsResourceIdsLinux(String... mandatoryArtifactsResourceIdsLinux) {
return mandatoryArtifactsResourceIdsLinux(List.of(mandatoryArtifactsResourceIdsLinux));
}
@CustomType.Setter
public Builder mandatoryArtifactsResourceIdsWindows(@Nullable List mandatoryArtifactsResourceIdsWindows) {
this.mandatoryArtifactsResourceIdsWindows = mandatoryArtifactsResourceIdsWindows;
return this;
}
public Builder mandatoryArtifactsResourceIdsWindows(String... mandatoryArtifactsResourceIdsWindows) {
return mandatoryArtifactsResourceIdsWindows(List.of(mandatoryArtifactsResourceIdsWindows));
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetLabResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder networkSecurityGroupId(String networkSecurityGroupId) {
if (networkSecurityGroupId == null) {
throw new MissingRequiredPropertyException("GetLabResult", "networkSecurityGroupId");
}
this.networkSecurityGroupId = networkSecurityGroupId;
return this;
}
@CustomType.Setter
public Builder premiumDataDiskStorageAccount(String premiumDataDiskStorageAccount) {
if (premiumDataDiskStorageAccount == null) {
throw new MissingRequiredPropertyException("GetLabResult", "premiumDataDiskStorageAccount");
}
this.premiumDataDiskStorageAccount = premiumDataDiskStorageAccount;
return this;
}
@CustomType.Setter
public Builder premiumDataDisks(@Nullable String premiumDataDisks) {
this.premiumDataDisks = premiumDataDisks;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetLabResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder publicIpId(String publicIpId) {
if (publicIpId == null) {
throw new MissingRequiredPropertyException("GetLabResult", "publicIpId");
}
this.publicIpId = publicIpId;
return this;
}
@CustomType.Setter
public Builder support(@Nullable LabSupportPropertiesResponse support) {
this.support = support;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetLabResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder uniqueIdentifier(String uniqueIdentifier) {
if (uniqueIdentifier == null) {
throw new MissingRequiredPropertyException("GetLabResult", "uniqueIdentifier");
}
this.uniqueIdentifier = uniqueIdentifier;
return this;
}
@CustomType.Setter
public Builder vaultName(String vaultName) {
if (vaultName == null) {
throw new MissingRequiredPropertyException("GetLabResult", "vaultName");
}
this.vaultName = vaultName;
return this;
}
@CustomType.Setter
public Builder vmCreationResourceGroup(String vmCreationResourceGroup) {
if (vmCreationResourceGroup == null) {
throw new MissingRequiredPropertyException("GetLabResult", "vmCreationResourceGroup");
}
this.vmCreationResourceGroup = vmCreationResourceGroup;
return this;
}
public GetLabResult build() {
final var _resultValue = new GetLabResult();
_resultValue.announcement = announcement;
_resultValue.artifactsStorageAccount = artifactsStorageAccount;
_resultValue.createdDate = createdDate;
_resultValue.defaultPremiumStorageAccount = defaultPremiumStorageAccount;
_resultValue.defaultStorageAccount = defaultStorageAccount;
_resultValue.environmentPermission = environmentPermission;
_resultValue.extendedProperties = extendedProperties;
_resultValue.id = id;
_resultValue.labStorageType = labStorageType;
_resultValue.loadBalancerId = loadBalancerId;
_resultValue.location = location;
_resultValue.mandatoryArtifactsResourceIdsLinux = mandatoryArtifactsResourceIdsLinux;
_resultValue.mandatoryArtifactsResourceIdsWindows = mandatoryArtifactsResourceIdsWindows;
_resultValue.name = name;
_resultValue.networkSecurityGroupId = networkSecurityGroupId;
_resultValue.premiumDataDiskStorageAccount = premiumDataDiskStorageAccount;
_resultValue.premiumDataDisks = premiumDataDisks;
_resultValue.provisioningState = provisioningState;
_resultValue.publicIpId = publicIpId;
_resultValue.support = support;
_resultValue.tags = tags;
_resultValue.type = type;
_resultValue.uniqueIdentifier = uniqueIdentifier;
_resultValue.vaultName = vaultName;
_resultValue.vmCreationResourceGroup = vmCreationResourceGroup;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy