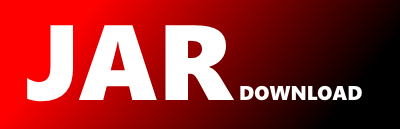
com.pulumi.azurenative.devtestlab.outputs.GetVirtualMachineResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.devtestlab.outputs;
import com.pulumi.azurenative.devtestlab.outputs.ApplicableScheduleResponse;
import com.pulumi.azurenative.devtestlab.outputs.ArtifactDeploymentStatusPropertiesResponse;
import com.pulumi.azurenative.devtestlab.outputs.ArtifactInstallPropertiesResponse;
import com.pulumi.azurenative.devtestlab.outputs.ComputeVmPropertiesResponse;
import com.pulumi.azurenative.devtestlab.outputs.DataDiskPropertiesResponse;
import com.pulumi.azurenative.devtestlab.outputs.GalleryImageReferenceResponse;
import com.pulumi.azurenative.devtestlab.outputs.NetworkInterfacePropertiesResponse;
import com.pulumi.azurenative.devtestlab.outputs.ScheduleCreationParameterResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetVirtualMachineResult {
/**
* @return Indicates whether another user can take ownership of the virtual machine
*
*/
private @Nullable Boolean allowClaim;
/**
* @return The applicable schedule for the virtual machine.
*
*/
private ApplicableScheduleResponse applicableSchedule;
/**
* @return The artifact deployment status for the virtual machine.
*
*/
private ArtifactDeploymentStatusPropertiesResponse artifactDeploymentStatus;
/**
* @return The artifacts to be installed on the virtual machine.
*
*/
private @Nullable List artifacts;
/**
* @return The resource identifier (Microsoft.Compute) of the virtual machine.
*
*/
private String computeId;
/**
* @return The compute virtual machine properties.
*
*/
private ComputeVmPropertiesResponse computeVm;
/**
* @return The email address of creator of the virtual machine.
*
*/
private String createdByUser;
/**
* @return The object identifier of the creator of the virtual machine.
*
*/
private String createdByUserId;
/**
* @return The creation date of the virtual machine.
*
*/
private @Nullable String createdDate;
/**
* @return The custom image identifier of the virtual machine.
*
*/
private @Nullable String customImageId;
/**
* @return New or existing data disks to attach to the virtual machine after creation
*
*/
private @Nullable List dataDiskParameters;
/**
* @return Indicates whether the virtual machine is to be created without a public IP address.
*
*/
private @Nullable Boolean disallowPublicIpAddress;
/**
* @return The resource ID of the environment that contains this virtual machine, if any.
*
*/
private @Nullable String environmentId;
/**
* @return The expiration date for VM.
*
*/
private @Nullable String expirationDate;
/**
* @return The fully-qualified domain name of the virtual machine.
*
*/
private String fqdn;
/**
* @return The Microsoft Azure Marketplace image reference of the virtual machine.
*
*/
private @Nullable GalleryImageReferenceResponse galleryImageReference;
/**
* @return The identifier of the resource.
*
*/
private String id;
/**
* @return Indicates whether this virtual machine uses an SSH key for authentication.
*
*/
private @Nullable Boolean isAuthenticationWithSshKey;
/**
* @return The lab subnet name of the virtual machine.
*
*/
private @Nullable String labSubnetName;
/**
* @return The lab virtual network identifier of the virtual machine.
*
*/
private @Nullable String labVirtualNetworkId;
/**
* @return Last known compute power state captured in DTL
*
*/
private String lastKnownPowerState;
/**
* @return The location of the resource.
*
*/
private @Nullable String location;
/**
* @return The name of the resource.
*
*/
private String name;
/**
* @return The network interface properties.
*
*/
private @Nullable NetworkInterfacePropertiesResponse networkInterface;
/**
* @return The notes of the virtual machine.
*
*/
private @Nullable String notes;
/**
* @return The OS type of the virtual machine.
*
*/
private String osType;
/**
* @return The object identifier of the owner of the virtual machine.
*
*/
private @Nullable String ownerObjectId;
/**
* @return The user principal name of the virtual machine owner.
*
*/
private @Nullable String ownerUserPrincipalName;
/**
* @return The password of the virtual machine administrator.
*
*/
private @Nullable String password;
/**
* @return The id of the plan associated with the virtual machine image
*
*/
private @Nullable String planId;
/**
* @return The provisioning status of the resource.
*
*/
private String provisioningState;
/**
* @return Virtual Machine schedules to be created
*
*/
private @Nullable List scheduleParameters;
/**
* @return The size of the virtual machine.
*
*/
private @Nullable String size;
/**
* @return The SSH key of the virtual machine administrator.
*
*/
private @Nullable String sshKey;
/**
* @return Storage type to use for virtual machine (i.e. Standard, Premium).
*
*/
private @Nullable String storageType;
/**
* @return The tags of the resource.
*
*/
private @Nullable Map tags;
/**
* @return The type of the resource.
*
*/
private String type;
/**
* @return The unique immutable identifier of a resource (Guid).
*
*/
private String uniqueIdentifier;
/**
* @return The user name of the virtual machine.
*
*/
private @Nullable String userName;
/**
* @return Tells source of creation of lab virtual machine. Output property only.
*
*/
private String virtualMachineCreationSource;
private GetVirtualMachineResult() {}
/**
* @return Indicates whether another user can take ownership of the virtual machine
*
*/
public Optional allowClaim() {
return Optional.ofNullable(this.allowClaim);
}
/**
* @return The applicable schedule for the virtual machine.
*
*/
public ApplicableScheduleResponse applicableSchedule() {
return this.applicableSchedule;
}
/**
* @return The artifact deployment status for the virtual machine.
*
*/
public ArtifactDeploymentStatusPropertiesResponse artifactDeploymentStatus() {
return this.artifactDeploymentStatus;
}
/**
* @return The artifacts to be installed on the virtual machine.
*
*/
public List artifacts() {
return this.artifacts == null ? List.of() : this.artifacts;
}
/**
* @return The resource identifier (Microsoft.Compute) of the virtual machine.
*
*/
public String computeId() {
return this.computeId;
}
/**
* @return The compute virtual machine properties.
*
*/
public ComputeVmPropertiesResponse computeVm() {
return this.computeVm;
}
/**
* @return The email address of creator of the virtual machine.
*
*/
public String createdByUser() {
return this.createdByUser;
}
/**
* @return The object identifier of the creator of the virtual machine.
*
*/
public String createdByUserId() {
return this.createdByUserId;
}
/**
* @return The creation date of the virtual machine.
*
*/
public Optional createdDate() {
return Optional.ofNullable(this.createdDate);
}
/**
* @return The custom image identifier of the virtual machine.
*
*/
public Optional customImageId() {
return Optional.ofNullable(this.customImageId);
}
/**
* @return New or existing data disks to attach to the virtual machine after creation
*
*/
public List dataDiskParameters() {
return this.dataDiskParameters == null ? List.of() : this.dataDiskParameters;
}
/**
* @return Indicates whether the virtual machine is to be created without a public IP address.
*
*/
public Optional disallowPublicIpAddress() {
return Optional.ofNullable(this.disallowPublicIpAddress);
}
/**
* @return The resource ID of the environment that contains this virtual machine, if any.
*
*/
public Optional environmentId() {
return Optional.ofNullable(this.environmentId);
}
/**
* @return The expiration date for VM.
*
*/
public Optional expirationDate() {
return Optional.ofNullable(this.expirationDate);
}
/**
* @return The fully-qualified domain name of the virtual machine.
*
*/
public String fqdn() {
return this.fqdn;
}
/**
* @return The Microsoft Azure Marketplace image reference of the virtual machine.
*
*/
public Optional galleryImageReference() {
return Optional.ofNullable(this.galleryImageReference);
}
/**
* @return The identifier of the resource.
*
*/
public String id() {
return this.id;
}
/**
* @return Indicates whether this virtual machine uses an SSH key for authentication.
*
*/
public Optional isAuthenticationWithSshKey() {
return Optional.ofNullable(this.isAuthenticationWithSshKey);
}
/**
* @return The lab subnet name of the virtual machine.
*
*/
public Optional labSubnetName() {
return Optional.ofNullable(this.labSubnetName);
}
/**
* @return The lab virtual network identifier of the virtual machine.
*
*/
public Optional labVirtualNetworkId() {
return Optional.ofNullable(this.labVirtualNetworkId);
}
/**
* @return Last known compute power state captured in DTL
*
*/
public String lastKnownPowerState() {
return this.lastKnownPowerState;
}
/**
* @return The location of the resource.
*
*/
public Optional location() {
return Optional.ofNullable(this.location);
}
/**
* @return The name of the resource.
*
*/
public String name() {
return this.name;
}
/**
* @return The network interface properties.
*
*/
public Optional networkInterface() {
return Optional.ofNullable(this.networkInterface);
}
/**
* @return The notes of the virtual machine.
*
*/
public Optional notes() {
return Optional.ofNullable(this.notes);
}
/**
* @return The OS type of the virtual machine.
*
*/
public String osType() {
return this.osType;
}
/**
* @return The object identifier of the owner of the virtual machine.
*
*/
public Optional ownerObjectId() {
return Optional.ofNullable(this.ownerObjectId);
}
/**
* @return The user principal name of the virtual machine owner.
*
*/
public Optional ownerUserPrincipalName() {
return Optional.ofNullable(this.ownerUserPrincipalName);
}
/**
* @return The password of the virtual machine administrator.
*
*/
public Optional password() {
return Optional.ofNullable(this.password);
}
/**
* @return The id of the plan associated with the virtual machine image
*
*/
public Optional planId() {
return Optional.ofNullable(this.planId);
}
/**
* @return The provisioning status of the resource.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return Virtual Machine schedules to be created
*
*/
public List scheduleParameters() {
return this.scheduleParameters == null ? List.of() : this.scheduleParameters;
}
/**
* @return The size of the virtual machine.
*
*/
public Optional size() {
return Optional.ofNullable(this.size);
}
/**
* @return The SSH key of the virtual machine administrator.
*
*/
public Optional sshKey() {
return Optional.ofNullable(this.sshKey);
}
/**
* @return Storage type to use for virtual machine (i.e. Standard, Premium).
*
*/
public Optional storageType() {
return Optional.ofNullable(this.storageType);
}
/**
* @return The tags of the resource.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return The type of the resource.
*
*/
public String type() {
return this.type;
}
/**
* @return The unique immutable identifier of a resource (Guid).
*
*/
public String uniqueIdentifier() {
return this.uniqueIdentifier;
}
/**
* @return The user name of the virtual machine.
*
*/
public Optional userName() {
return Optional.ofNullable(this.userName);
}
/**
* @return Tells source of creation of lab virtual machine. Output property only.
*
*/
public String virtualMachineCreationSource() {
return this.virtualMachineCreationSource;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetVirtualMachineResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Boolean allowClaim;
private ApplicableScheduleResponse applicableSchedule;
private ArtifactDeploymentStatusPropertiesResponse artifactDeploymentStatus;
private @Nullable List artifacts;
private String computeId;
private ComputeVmPropertiesResponse computeVm;
private String createdByUser;
private String createdByUserId;
private @Nullable String createdDate;
private @Nullable String customImageId;
private @Nullable List dataDiskParameters;
private @Nullable Boolean disallowPublicIpAddress;
private @Nullable String environmentId;
private @Nullable String expirationDate;
private String fqdn;
private @Nullable GalleryImageReferenceResponse galleryImageReference;
private String id;
private @Nullable Boolean isAuthenticationWithSshKey;
private @Nullable String labSubnetName;
private @Nullable String labVirtualNetworkId;
private String lastKnownPowerState;
private @Nullable String location;
private String name;
private @Nullable NetworkInterfacePropertiesResponse networkInterface;
private @Nullable String notes;
private String osType;
private @Nullable String ownerObjectId;
private @Nullable String ownerUserPrincipalName;
private @Nullable String password;
private @Nullable String planId;
private String provisioningState;
private @Nullable List scheduleParameters;
private @Nullable String size;
private @Nullable String sshKey;
private @Nullable String storageType;
private @Nullable Map tags;
private String type;
private String uniqueIdentifier;
private @Nullable String userName;
private String virtualMachineCreationSource;
public Builder() {}
public Builder(GetVirtualMachineResult defaults) {
Objects.requireNonNull(defaults);
this.allowClaim = defaults.allowClaim;
this.applicableSchedule = defaults.applicableSchedule;
this.artifactDeploymentStatus = defaults.artifactDeploymentStatus;
this.artifacts = defaults.artifacts;
this.computeId = defaults.computeId;
this.computeVm = defaults.computeVm;
this.createdByUser = defaults.createdByUser;
this.createdByUserId = defaults.createdByUserId;
this.createdDate = defaults.createdDate;
this.customImageId = defaults.customImageId;
this.dataDiskParameters = defaults.dataDiskParameters;
this.disallowPublicIpAddress = defaults.disallowPublicIpAddress;
this.environmentId = defaults.environmentId;
this.expirationDate = defaults.expirationDate;
this.fqdn = defaults.fqdn;
this.galleryImageReference = defaults.galleryImageReference;
this.id = defaults.id;
this.isAuthenticationWithSshKey = defaults.isAuthenticationWithSshKey;
this.labSubnetName = defaults.labSubnetName;
this.labVirtualNetworkId = defaults.labVirtualNetworkId;
this.lastKnownPowerState = defaults.lastKnownPowerState;
this.location = defaults.location;
this.name = defaults.name;
this.networkInterface = defaults.networkInterface;
this.notes = defaults.notes;
this.osType = defaults.osType;
this.ownerObjectId = defaults.ownerObjectId;
this.ownerUserPrincipalName = defaults.ownerUserPrincipalName;
this.password = defaults.password;
this.planId = defaults.planId;
this.provisioningState = defaults.provisioningState;
this.scheduleParameters = defaults.scheduleParameters;
this.size = defaults.size;
this.sshKey = defaults.sshKey;
this.storageType = defaults.storageType;
this.tags = defaults.tags;
this.type = defaults.type;
this.uniqueIdentifier = defaults.uniqueIdentifier;
this.userName = defaults.userName;
this.virtualMachineCreationSource = defaults.virtualMachineCreationSource;
}
@CustomType.Setter
public Builder allowClaim(@Nullable Boolean allowClaim) {
this.allowClaim = allowClaim;
return this;
}
@CustomType.Setter
public Builder applicableSchedule(ApplicableScheduleResponse applicableSchedule) {
if (applicableSchedule == null) {
throw new MissingRequiredPropertyException("GetVirtualMachineResult", "applicableSchedule");
}
this.applicableSchedule = applicableSchedule;
return this;
}
@CustomType.Setter
public Builder artifactDeploymentStatus(ArtifactDeploymentStatusPropertiesResponse artifactDeploymentStatus) {
if (artifactDeploymentStatus == null) {
throw new MissingRequiredPropertyException("GetVirtualMachineResult", "artifactDeploymentStatus");
}
this.artifactDeploymentStatus = artifactDeploymentStatus;
return this;
}
@CustomType.Setter
public Builder artifacts(@Nullable List artifacts) {
this.artifacts = artifacts;
return this;
}
public Builder artifacts(ArtifactInstallPropertiesResponse... artifacts) {
return artifacts(List.of(artifacts));
}
@CustomType.Setter
public Builder computeId(String computeId) {
if (computeId == null) {
throw new MissingRequiredPropertyException("GetVirtualMachineResult", "computeId");
}
this.computeId = computeId;
return this;
}
@CustomType.Setter
public Builder computeVm(ComputeVmPropertiesResponse computeVm) {
if (computeVm == null) {
throw new MissingRequiredPropertyException("GetVirtualMachineResult", "computeVm");
}
this.computeVm = computeVm;
return this;
}
@CustomType.Setter
public Builder createdByUser(String createdByUser) {
if (createdByUser == null) {
throw new MissingRequiredPropertyException("GetVirtualMachineResult", "createdByUser");
}
this.createdByUser = createdByUser;
return this;
}
@CustomType.Setter
public Builder createdByUserId(String createdByUserId) {
if (createdByUserId == null) {
throw new MissingRequiredPropertyException("GetVirtualMachineResult", "createdByUserId");
}
this.createdByUserId = createdByUserId;
return this;
}
@CustomType.Setter
public Builder createdDate(@Nullable String createdDate) {
this.createdDate = createdDate;
return this;
}
@CustomType.Setter
public Builder customImageId(@Nullable String customImageId) {
this.customImageId = customImageId;
return this;
}
@CustomType.Setter
public Builder dataDiskParameters(@Nullable List dataDiskParameters) {
this.dataDiskParameters = dataDiskParameters;
return this;
}
public Builder dataDiskParameters(DataDiskPropertiesResponse... dataDiskParameters) {
return dataDiskParameters(List.of(dataDiskParameters));
}
@CustomType.Setter
public Builder disallowPublicIpAddress(@Nullable Boolean disallowPublicIpAddress) {
this.disallowPublicIpAddress = disallowPublicIpAddress;
return this;
}
@CustomType.Setter
public Builder environmentId(@Nullable String environmentId) {
this.environmentId = environmentId;
return this;
}
@CustomType.Setter
public Builder expirationDate(@Nullable String expirationDate) {
this.expirationDate = expirationDate;
return this;
}
@CustomType.Setter
public Builder fqdn(String fqdn) {
if (fqdn == null) {
throw new MissingRequiredPropertyException("GetVirtualMachineResult", "fqdn");
}
this.fqdn = fqdn;
return this;
}
@CustomType.Setter
public Builder galleryImageReference(@Nullable GalleryImageReferenceResponse galleryImageReference) {
this.galleryImageReference = galleryImageReference;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetVirtualMachineResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder isAuthenticationWithSshKey(@Nullable Boolean isAuthenticationWithSshKey) {
this.isAuthenticationWithSshKey = isAuthenticationWithSshKey;
return this;
}
@CustomType.Setter
public Builder labSubnetName(@Nullable String labSubnetName) {
this.labSubnetName = labSubnetName;
return this;
}
@CustomType.Setter
public Builder labVirtualNetworkId(@Nullable String labVirtualNetworkId) {
this.labVirtualNetworkId = labVirtualNetworkId;
return this;
}
@CustomType.Setter
public Builder lastKnownPowerState(String lastKnownPowerState) {
if (lastKnownPowerState == null) {
throw new MissingRequiredPropertyException("GetVirtualMachineResult", "lastKnownPowerState");
}
this.lastKnownPowerState = lastKnownPowerState;
return this;
}
@CustomType.Setter
public Builder location(@Nullable String location) {
this.location = location;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetVirtualMachineResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder networkInterface(@Nullable NetworkInterfacePropertiesResponse networkInterface) {
this.networkInterface = networkInterface;
return this;
}
@CustomType.Setter
public Builder notes(@Nullable String notes) {
this.notes = notes;
return this;
}
@CustomType.Setter
public Builder osType(String osType) {
if (osType == null) {
throw new MissingRequiredPropertyException("GetVirtualMachineResult", "osType");
}
this.osType = osType;
return this;
}
@CustomType.Setter
public Builder ownerObjectId(@Nullable String ownerObjectId) {
this.ownerObjectId = ownerObjectId;
return this;
}
@CustomType.Setter
public Builder ownerUserPrincipalName(@Nullable String ownerUserPrincipalName) {
this.ownerUserPrincipalName = ownerUserPrincipalName;
return this;
}
@CustomType.Setter
public Builder password(@Nullable String password) {
this.password = password;
return this;
}
@CustomType.Setter
public Builder planId(@Nullable String planId) {
this.planId = planId;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetVirtualMachineResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder scheduleParameters(@Nullable List scheduleParameters) {
this.scheduleParameters = scheduleParameters;
return this;
}
public Builder scheduleParameters(ScheduleCreationParameterResponse... scheduleParameters) {
return scheduleParameters(List.of(scheduleParameters));
}
@CustomType.Setter
public Builder size(@Nullable String size) {
this.size = size;
return this;
}
@CustomType.Setter
public Builder sshKey(@Nullable String sshKey) {
this.sshKey = sshKey;
return this;
}
@CustomType.Setter
public Builder storageType(@Nullable String storageType) {
this.storageType = storageType;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetVirtualMachineResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder uniqueIdentifier(String uniqueIdentifier) {
if (uniqueIdentifier == null) {
throw new MissingRequiredPropertyException("GetVirtualMachineResult", "uniqueIdentifier");
}
this.uniqueIdentifier = uniqueIdentifier;
return this;
}
@CustomType.Setter
public Builder userName(@Nullable String userName) {
this.userName = userName;
return this;
}
@CustomType.Setter
public Builder virtualMachineCreationSource(String virtualMachineCreationSource) {
if (virtualMachineCreationSource == null) {
throw new MissingRequiredPropertyException("GetVirtualMachineResult", "virtualMachineCreationSource");
}
this.virtualMachineCreationSource = virtualMachineCreationSource;
return this;
}
public GetVirtualMachineResult build() {
final var _resultValue = new GetVirtualMachineResult();
_resultValue.allowClaim = allowClaim;
_resultValue.applicableSchedule = applicableSchedule;
_resultValue.artifactDeploymentStatus = artifactDeploymentStatus;
_resultValue.artifacts = artifacts;
_resultValue.computeId = computeId;
_resultValue.computeVm = computeVm;
_resultValue.createdByUser = createdByUser;
_resultValue.createdByUserId = createdByUserId;
_resultValue.createdDate = createdDate;
_resultValue.customImageId = customImageId;
_resultValue.dataDiskParameters = dataDiskParameters;
_resultValue.disallowPublicIpAddress = disallowPublicIpAddress;
_resultValue.environmentId = environmentId;
_resultValue.expirationDate = expirationDate;
_resultValue.fqdn = fqdn;
_resultValue.galleryImageReference = galleryImageReference;
_resultValue.id = id;
_resultValue.isAuthenticationWithSshKey = isAuthenticationWithSshKey;
_resultValue.labSubnetName = labSubnetName;
_resultValue.labVirtualNetworkId = labVirtualNetworkId;
_resultValue.lastKnownPowerState = lastKnownPowerState;
_resultValue.location = location;
_resultValue.name = name;
_resultValue.networkInterface = networkInterface;
_resultValue.notes = notes;
_resultValue.osType = osType;
_resultValue.ownerObjectId = ownerObjectId;
_resultValue.ownerUserPrincipalName = ownerUserPrincipalName;
_resultValue.password = password;
_resultValue.planId = planId;
_resultValue.provisioningState = provisioningState;
_resultValue.scheduleParameters = scheduleParameters;
_resultValue.size = size;
_resultValue.sshKey = sshKey;
_resultValue.storageType = storageType;
_resultValue.tags = tags;
_resultValue.type = type;
_resultValue.uniqueIdentifier = uniqueIdentifier;
_resultValue.userName = userName;
_resultValue.virtualMachineCreationSource = virtualMachineCreationSource;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy