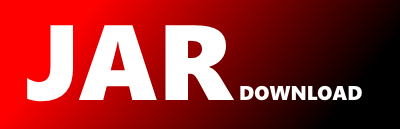
com.pulumi.azurenative.digitaltwins.outputs.ServiceBusResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.digitaltwins.outputs;
import com.pulumi.azurenative.digitaltwins.outputs.ManagedIdentityReferenceResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ServiceBusResponse {
/**
* @return Specifies the authentication type being used for connecting to the endpoint. Defaults to 'KeyBased'. If 'KeyBased' is selected, a connection string must be specified (at least the primary connection string). If 'IdentityBased' is select, the endpointUri and entityPath properties must be specified.
*
*/
private @Nullable String authenticationType;
/**
* @return Time when the Endpoint was added to DigitalTwinsInstance.
*
*/
private String createdTime;
/**
* @return Dead letter storage secret for key-based authentication. Will be obfuscated during read.
*
*/
private @Nullable String deadLetterSecret;
/**
* @return Dead letter storage URL for identity-based authentication.
*
*/
private @Nullable String deadLetterUri;
/**
* @return The type of Digital Twins endpoint
* Expected value is 'ServiceBus'.
*
*/
private String endpointType;
/**
* @return The URL of the ServiceBus namespace for identity-based authentication. It must include the protocol 'sb://'.
*
*/
private @Nullable String endpointUri;
/**
* @return The ServiceBus Topic name for identity-based authentication.
*
*/
private @Nullable String entityPath;
/**
* @return Managed identity properties for the endpoint.
*
*/
private @Nullable ManagedIdentityReferenceResponse identity;
/**
* @return PrimaryConnectionString of the endpoint for key-based authentication. Will be obfuscated during read.
*
*/
private @Nullable String primaryConnectionString;
/**
* @return The provisioning state.
*
*/
private String provisioningState;
/**
* @return SecondaryConnectionString of the endpoint for key-based authentication. Will be obfuscated during read.
*
*/
private @Nullable String secondaryConnectionString;
private ServiceBusResponse() {}
/**
* @return Specifies the authentication type being used for connecting to the endpoint. Defaults to 'KeyBased'. If 'KeyBased' is selected, a connection string must be specified (at least the primary connection string). If 'IdentityBased' is select, the endpointUri and entityPath properties must be specified.
*
*/
public Optional authenticationType() {
return Optional.ofNullable(this.authenticationType);
}
/**
* @return Time when the Endpoint was added to DigitalTwinsInstance.
*
*/
public String createdTime() {
return this.createdTime;
}
/**
* @return Dead letter storage secret for key-based authentication. Will be obfuscated during read.
*
*/
public Optional deadLetterSecret() {
return Optional.ofNullable(this.deadLetterSecret);
}
/**
* @return Dead letter storage URL for identity-based authentication.
*
*/
public Optional deadLetterUri() {
return Optional.ofNullable(this.deadLetterUri);
}
/**
* @return The type of Digital Twins endpoint
* Expected value is 'ServiceBus'.
*
*/
public String endpointType() {
return this.endpointType;
}
/**
* @return The URL of the ServiceBus namespace for identity-based authentication. It must include the protocol 'sb://'.
*
*/
public Optional endpointUri() {
return Optional.ofNullable(this.endpointUri);
}
/**
* @return The ServiceBus Topic name for identity-based authentication.
*
*/
public Optional entityPath() {
return Optional.ofNullable(this.entityPath);
}
/**
* @return Managed identity properties for the endpoint.
*
*/
public Optional identity() {
return Optional.ofNullable(this.identity);
}
/**
* @return PrimaryConnectionString of the endpoint for key-based authentication. Will be obfuscated during read.
*
*/
public Optional primaryConnectionString() {
return Optional.ofNullable(this.primaryConnectionString);
}
/**
* @return The provisioning state.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return SecondaryConnectionString of the endpoint for key-based authentication. Will be obfuscated during read.
*
*/
public Optional secondaryConnectionString() {
return Optional.ofNullable(this.secondaryConnectionString);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ServiceBusResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String authenticationType;
private String createdTime;
private @Nullable String deadLetterSecret;
private @Nullable String deadLetterUri;
private String endpointType;
private @Nullable String endpointUri;
private @Nullable String entityPath;
private @Nullable ManagedIdentityReferenceResponse identity;
private @Nullable String primaryConnectionString;
private String provisioningState;
private @Nullable String secondaryConnectionString;
public Builder() {}
public Builder(ServiceBusResponse defaults) {
Objects.requireNonNull(defaults);
this.authenticationType = defaults.authenticationType;
this.createdTime = defaults.createdTime;
this.deadLetterSecret = defaults.deadLetterSecret;
this.deadLetterUri = defaults.deadLetterUri;
this.endpointType = defaults.endpointType;
this.endpointUri = defaults.endpointUri;
this.entityPath = defaults.entityPath;
this.identity = defaults.identity;
this.primaryConnectionString = defaults.primaryConnectionString;
this.provisioningState = defaults.provisioningState;
this.secondaryConnectionString = defaults.secondaryConnectionString;
}
@CustomType.Setter
public Builder authenticationType(@Nullable String authenticationType) {
this.authenticationType = authenticationType;
return this;
}
@CustomType.Setter
public Builder createdTime(String createdTime) {
if (createdTime == null) {
throw new MissingRequiredPropertyException("ServiceBusResponse", "createdTime");
}
this.createdTime = createdTime;
return this;
}
@CustomType.Setter
public Builder deadLetterSecret(@Nullable String deadLetterSecret) {
this.deadLetterSecret = deadLetterSecret;
return this;
}
@CustomType.Setter
public Builder deadLetterUri(@Nullable String deadLetterUri) {
this.deadLetterUri = deadLetterUri;
return this;
}
@CustomType.Setter
public Builder endpointType(String endpointType) {
if (endpointType == null) {
throw new MissingRequiredPropertyException("ServiceBusResponse", "endpointType");
}
this.endpointType = endpointType;
return this;
}
@CustomType.Setter
public Builder endpointUri(@Nullable String endpointUri) {
this.endpointUri = endpointUri;
return this;
}
@CustomType.Setter
public Builder entityPath(@Nullable String entityPath) {
this.entityPath = entityPath;
return this;
}
@CustomType.Setter
public Builder identity(@Nullable ManagedIdentityReferenceResponse identity) {
this.identity = identity;
return this;
}
@CustomType.Setter
public Builder primaryConnectionString(@Nullable String primaryConnectionString) {
this.primaryConnectionString = primaryConnectionString;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("ServiceBusResponse", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder secondaryConnectionString(@Nullable String secondaryConnectionString) {
this.secondaryConnectionString = secondaryConnectionString;
return this;
}
public ServiceBusResponse build() {
final var _resultValue = new ServiceBusResponse();
_resultValue.authenticationType = authenticationType;
_resultValue.createdTime = createdTime;
_resultValue.deadLetterSecret = deadLetterSecret;
_resultValue.deadLetterUri = deadLetterUri;
_resultValue.endpointType = endpointType;
_resultValue.endpointUri = endpointUri;
_resultValue.entityPath = entityPath;
_resultValue.identity = identity;
_resultValue.primaryConnectionString = primaryConnectionString;
_resultValue.provisioningState = provisioningState;
_resultValue.secondaryConnectionString = secondaryConnectionString;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy