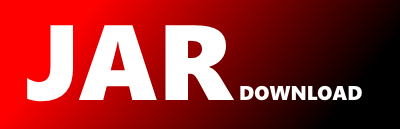
com.pulumi.azurenative.domainregistration.inputs.AddressArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.domainregistration.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Address information for domain registration.
*
*/
public final class AddressArgs extends com.pulumi.resources.ResourceArgs {
public static final AddressArgs Empty = new AddressArgs();
/**
* First line of an Address.
*
*/
@Import(name="address1", required=true)
private Output address1;
/**
* @return First line of an Address.
*
*/
public Output address1() {
return this.address1;
}
/**
* The second line of the Address. Optional.
*
*/
@Import(name="address2")
private @Nullable Output address2;
/**
* @return The second line of the Address. Optional.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy