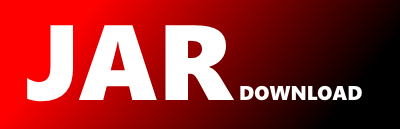
com.pulumi.azurenative.elastic.outputs.ElasticCloudDeploymentResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.elastic.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class ElasticCloudDeploymentResponse {
/**
* @return Associated Azure subscription Id for the elastic deployment.
*
*/
private String azureSubscriptionId;
/**
* @return Elastic deployment Id
*
*/
private String deploymentId;
/**
* @return Region where Deployment at Elastic side took place.
*
*/
private String elasticsearchRegion;
/**
* @return Elasticsearch ingestion endpoint of the Elastic deployment.
*
*/
private String elasticsearchServiceUrl;
/**
* @return Kibana endpoint of the Elastic deployment.
*
*/
private String kibanaServiceUrl;
/**
* @return Kibana dashboard sso URL of the Elastic deployment.
*
*/
private String kibanaSsoUrl;
/**
* @return Elastic deployment name
*
*/
private String name;
private ElasticCloudDeploymentResponse() {}
/**
* @return Associated Azure subscription Id for the elastic deployment.
*
*/
public String azureSubscriptionId() {
return this.azureSubscriptionId;
}
/**
* @return Elastic deployment Id
*
*/
public String deploymentId() {
return this.deploymentId;
}
/**
* @return Region where Deployment at Elastic side took place.
*
*/
public String elasticsearchRegion() {
return this.elasticsearchRegion;
}
/**
* @return Elasticsearch ingestion endpoint of the Elastic deployment.
*
*/
public String elasticsearchServiceUrl() {
return this.elasticsearchServiceUrl;
}
/**
* @return Kibana endpoint of the Elastic deployment.
*
*/
public String kibanaServiceUrl() {
return this.kibanaServiceUrl;
}
/**
* @return Kibana dashboard sso URL of the Elastic deployment.
*
*/
public String kibanaSsoUrl() {
return this.kibanaSsoUrl;
}
/**
* @return Elastic deployment name
*
*/
public String name() {
return this.name;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ElasticCloudDeploymentResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String azureSubscriptionId;
private String deploymentId;
private String elasticsearchRegion;
private String elasticsearchServiceUrl;
private String kibanaServiceUrl;
private String kibanaSsoUrl;
private String name;
public Builder() {}
public Builder(ElasticCloudDeploymentResponse defaults) {
Objects.requireNonNull(defaults);
this.azureSubscriptionId = defaults.azureSubscriptionId;
this.deploymentId = defaults.deploymentId;
this.elasticsearchRegion = defaults.elasticsearchRegion;
this.elasticsearchServiceUrl = defaults.elasticsearchServiceUrl;
this.kibanaServiceUrl = defaults.kibanaServiceUrl;
this.kibanaSsoUrl = defaults.kibanaSsoUrl;
this.name = defaults.name;
}
@CustomType.Setter
public Builder azureSubscriptionId(String azureSubscriptionId) {
if (azureSubscriptionId == null) {
throw new MissingRequiredPropertyException("ElasticCloudDeploymentResponse", "azureSubscriptionId");
}
this.azureSubscriptionId = azureSubscriptionId;
return this;
}
@CustomType.Setter
public Builder deploymentId(String deploymentId) {
if (deploymentId == null) {
throw new MissingRequiredPropertyException("ElasticCloudDeploymentResponse", "deploymentId");
}
this.deploymentId = deploymentId;
return this;
}
@CustomType.Setter
public Builder elasticsearchRegion(String elasticsearchRegion) {
if (elasticsearchRegion == null) {
throw new MissingRequiredPropertyException("ElasticCloudDeploymentResponse", "elasticsearchRegion");
}
this.elasticsearchRegion = elasticsearchRegion;
return this;
}
@CustomType.Setter
public Builder elasticsearchServiceUrl(String elasticsearchServiceUrl) {
if (elasticsearchServiceUrl == null) {
throw new MissingRequiredPropertyException("ElasticCloudDeploymentResponse", "elasticsearchServiceUrl");
}
this.elasticsearchServiceUrl = elasticsearchServiceUrl;
return this;
}
@CustomType.Setter
public Builder kibanaServiceUrl(String kibanaServiceUrl) {
if (kibanaServiceUrl == null) {
throw new MissingRequiredPropertyException("ElasticCloudDeploymentResponse", "kibanaServiceUrl");
}
this.kibanaServiceUrl = kibanaServiceUrl;
return this;
}
@CustomType.Setter
public Builder kibanaSsoUrl(String kibanaSsoUrl) {
if (kibanaSsoUrl == null) {
throw new MissingRequiredPropertyException("ElasticCloudDeploymentResponse", "kibanaSsoUrl");
}
this.kibanaSsoUrl = kibanaSsoUrl;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("ElasticCloudDeploymentResponse", "name");
}
this.name = name;
return this;
}
public ElasticCloudDeploymentResponse build() {
final var _resultValue = new ElasticCloudDeploymentResponse();
_resultValue.azureSubscriptionId = azureSubscriptionId;
_resultValue.deploymentId = deploymentId;
_resultValue.elasticsearchRegion = elasticsearchRegion;
_resultValue.elasticsearchServiceUrl = elasticsearchServiceUrl;
_resultValue.kibanaServiceUrl = kibanaServiceUrl;
_resultValue.kibanaSsoUrl = kibanaSsoUrl;
_resultValue.name = name;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy