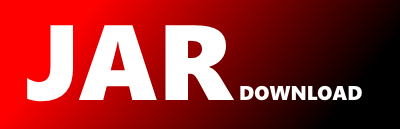
com.pulumi.azurenative.eventgrid.outputs.GetDomainResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.eventgrid.outputs;
import com.pulumi.azurenative.eventgrid.outputs.IdentityInfoResponse;
import com.pulumi.azurenative.eventgrid.outputs.InboundIpRuleResponse;
import com.pulumi.azurenative.eventgrid.outputs.JsonInputSchemaMappingResponse;
import com.pulumi.azurenative.eventgrid.outputs.PrivateEndpointConnectionResponse;
import com.pulumi.azurenative.eventgrid.outputs.SystemDataResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetDomainResult {
/**
* @return This Boolean is used to specify the creation mechanism for 'all' the Event Grid Domain Topics associated with this Event Grid Domain resource.
* In this context, creation of domain topic can be auto-managed (when true) or self-managed (when false). The default value for this property is true.
* When this property is null or set to true, Event Grid is responsible of automatically creating the domain topic when the first event subscription is
* created at the scope of the domain topic. If this property is set to false, then creating the first event subscription will require creating a domain topic
* by the user. The self-management mode can be used if the user wants full control of when the domain topic is created, while auto-managed mode provides the
* flexibility to perform less operations and manage fewer resources by the user. Also, note that in auto-managed creation mode, user is allowed to create the
* domain topic on demand if needed.
*
*/
private @Nullable Boolean autoCreateTopicWithFirstSubscription;
/**
* @return This Boolean is used to specify the deletion mechanism for 'all' the Event Grid Domain Topics associated with this Event Grid Domain resource.
* In this context, deletion of domain topic can be auto-managed (when true) or self-managed (when false). The default value for this property is true.
* When this property is set to true, Event Grid is responsible of automatically deleting the domain topic when the last event subscription at the scope
* of the domain topic is deleted. If this property is set to false, then the user needs to manually delete the domain topic when it is no longer needed
* (e.g., when last event subscription is deleted and the resource needs to be cleaned up). The self-management mode can be used if the user wants full
* control of when the domain topic needs to be deleted, while auto-managed mode provides the flexibility to perform less operations and manage fewer
* resources by the user.
*
*/
private @Nullable Boolean autoDeleteTopicWithLastSubscription;
/**
* @return Data Residency Boundary of the resource.
*
*/
private @Nullable String dataResidencyBoundary;
/**
* @return This boolean is used to enable or disable local auth. Default value is false. When the property is set to true, only AAD token will be used to authenticate if user is allowed to publish to the domain.
*
*/
private @Nullable Boolean disableLocalAuth;
/**
* @return Endpoint for the Event Grid Domain Resource which is used for publishing the events.
*
*/
private String endpoint;
/**
* @return Fully qualified identifier of the resource.
*
*/
private String id;
/**
* @return Identity information for the Event Grid Domain resource.
*
*/
private @Nullable IdentityInfoResponse identity;
/**
* @return This can be used to restrict traffic from specific IPs instead of all IPs. Note: These are considered only if PublicNetworkAccess is enabled.
*
*/
private @Nullable List inboundIpRules;
/**
* @return This determines the format that Event Grid should expect for incoming events published to the Event Grid Domain Resource.
*
*/
private @Nullable String inputSchema;
/**
* @return Information about the InputSchemaMapping which specified the info about mapping event payload.
*
*/
private @Nullable JsonInputSchemaMappingResponse inputSchemaMapping;
/**
* @return Location of the resource.
*
*/
private String location;
/**
* @return Metric resource id for the Event Grid Domain Resource.
*
*/
private String metricResourceId;
/**
* @return Name of the resource.
*
*/
private String name;
/**
* @return List of private endpoint connections.
*
*/
private List privateEndpointConnections;
/**
* @return Provisioning state of the Event Grid Domain Resource.
*
*/
private String provisioningState;
/**
* @return This determines if traffic is allowed over public network. By default it is enabled.
* You can further restrict to specific IPs by configuring <seealso cref="P:Microsoft.Azure.Events.ResourceProvider.Common.Contracts.DomainProperties.InboundIpRules" />
*
*/
private @Nullable String publicNetworkAccess;
/**
* @return The system metadata relating to the Event Grid Domain resource.
*
*/
private SystemDataResponse systemData;
/**
* @return Tags of the resource.
*
*/
private @Nullable Map tags;
/**
* @return Type of the resource.
*
*/
private String type;
private GetDomainResult() {}
/**
* @return This Boolean is used to specify the creation mechanism for 'all' the Event Grid Domain Topics associated with this Event Grid Domain resource.
* In this context, creation of domain topic can be auto-managed (when true) or self-managed (when false). The default value for this property is true.
* When this property is null or set to true, Event Grid is responsible of automatically creating the domain topic when the first event subscription is
* created at the scope of the domain topic. If this property is set to false, then creating the first event subscription will require creating a domain topic
* by the user. The self-management mode can be used if the user wants full control of when the domain topic is created, while auto-managed mode provides the
* flexibility to perform less operations and manage fewer resources by the user. Also, note that in auto-managed creation mode, user is allowed to create the
* domain topic on demand if needed.
*
*/
public Optional autoCreateTopicWithFirstSubscription() {
return Optional.ofNullable(this.autoCreateTopicWithFirstSubscription);
}
/**
* @return This Boolean is used to specify the deletion mechanism for 'all' the Event Grid Domain Topics associated with this Event Grid Domain resource.
* In this context, deletion of domain topic can be auto-managed (when true) or self-managed (when false). The default value for this property is true.
* When this property is set to true, Event Grid is responsible of automatically deleting the domain topic when the last event subscription at the scope
* of the domain topic is deleted. If this property is set to false, then the user needs to manually delete the domain topic when it is no longer needed
* (e.g., when last event subscription is deleted and the resource needs to be cleaned up). The self-management mode can be used if the user wants full
* control of when the domain topic needs to be deleted, while auto-managed mode provides the flexibility to perform less operations and manage fewer
* resources by the user.
*
*/
public Optional autoDeleteTopicWithLastSubscription() {
return Optional.ofNullable(this.autoDeleteTopicWithLastSubscription);
}
/**
* @return Data Residency Boundary of the resource.
*
*/
public Optional dataResidencyBoundary() {
return Optional.ofNullable(this.dataResidencyBoundary);
}
/**
* @return This boolean is used to enable or disable local auth. Default value is false. When the property is set to true, only AAD token will be used to authenticate if user is allowed to publish to the domain.
*
*/
public Optional disableLocalAuth() {
return Optional.ofNullable(this.disableLocalAuth);
}
/**
* @return Endpoint for the Event Grid Domain Resource which is used for publishing the events.
*
*/
public String endpoint() {
return this.endpoint;
}
/**
* @return Fully qualified identifier of the resource.
*
*/
public String id() {
return this.id;
}
/**
* @return Identity information for the Event Grid Domain resource.
*
*/
public Optional identity() {
return Optional.ofNullable(this.identity);
}
/**
* @return This can be used to restrict traffic from specific IPs instead of all IPs. Note: These are considered only if PublicNetworkAccess is enabled.
*
*/
public List inboundIpRules() {
return this.inboundIpRules == null ? List.of() : this.inboundIpRules;
}
/**
* @return This determines the format that Event Grid should expect for incoming events published to the Event Grid Domain Resource.
*
*/
public Optional inputSchema() {
return Optional.ofNullable(this.inputSchema);
}
/**
* @return Information about the InputSchemaMapping which specified the info about mapping event payload.
*
*/
public Optional inputSchemaMapping() {
return Optional.ofNullable(this.inputSchemaMapping);
}
/**
* @return Location of the resource.
*
*/
public String location() {
return this.location;
}
/**
* @return Metric resource id for the Event Grid Domain Resource.
*
*/
public String metricResourceId() {
return this.metricResourceId;
}
/**
* @return Name of the resource.
*
*/
public String name() {
return this.name;
}
/**
* @return List of private endpoint connections.
*
*/
public List privateEndpointConnections() {
return this.privateEndpointConnections;
}
/**
* @return Provisioning state of the Event Grid Domain Resource.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return This determines if traffic is allowed over public network. By default it is enabled.
* You can further restrict to specific IPs by configuring <seealso cref="P:Microsoft.Azure.Events.ResourceProvider.Common.Contracts.DomainProperties.InboundIpRules" />
*
*/
public Optional publicNetworkAccess() {
return Optional.ofNullable(this.publicNetworkAccess);
}
/**
* @return The system metadata relating to the Event Grid Domain resource.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return Tags of the resource.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return Type of the resource.
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetDomainResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Boolean autoCreateTopicWithFirstSubscription;
private @Nullable Boolean autoDeleteTopicWithLastSubscription;
private @Nullable String dataResidencyBoundary;
private @Nullable Boolean disableLocalAuth;
private String endpoint;
private String id;
private @Nullable IdentityInfoResponse identity;
private @Nullable List inboundIpRules;
private @Nullable String inputSchema;
private @Nullable JsonInputSchemaMappingResponse inputSchemaMapping;
private String location;
private String metricResourceId;
private String name;
private List privateEndpointConnections;
private String provisioningState;
private @Nullable String publicNetworkAccess;
private SystemDataResponse systemData;
private @Nullable Map tags;
private String type;
public Builder() {}
public Builder(GetDomainResult defaults) {
Objects.requireNonNull(defaults);
this.autoCreateTopicWithFirstSubscription = defaults.autoCreateTopicWithFirstSubscription;
this.autoDeleteTopicWithLastSubscription = defaults.autoDeleteTopicWithLastSubscription;
this.dataResidencyBoundary = defaults.dataResidencyBoundary;
this.disableLocalAuth = defaults.disableLocalAuth;
this.endpoint = defaults.endpoint;
this.id = defaults.id;
this.identity = defaults.identity;
this.inboundIpRules = defaults.inboundIpRules;
this.inputSchema = defaults.inputSchema;
this.inputSchemaMapping = defaults.inputSchemaMapping;
this.location = defaults.location;
this.metricResourceId = defaults.metricResourceId;
this.name = defaults.name;
this.privateEndpointConnections = defaults.privateEndpointConnections;
this.provisioningState = defaults.provisioningState;
this.publicNetworkAccess = defaults.publicNetworkAccess;
this.systemData = defaults.systemData;
this.tags = defaults.tags;
this.type = defaults.type;
}
@CustomType.Setter
public Builder autoCreateTopicWithFirstSubscription(@Nullable Boolean autoCreateTopicWithFirstSubscription) {
this.autoCreateTopicWithFirstSubscription = autoCreateTopicWithFirstSubscription;
return this;
}
@CustomType.Setter
public Builder autoDeleteTopicWithLastSubscription(@Nullable Boolean autoDeleteTopicWithLastSubscription) {
this.autoDeleteTopicWithLastSubscription = autoDeleteTopicWithLastSubscription;
return this;
}
@CustomType.Setter
public Builder dataResidencyBoundary(@Nullable String dataResidencyBoundary) {
this.dataResidencyBoundary = dataResidencyBoundary;
return this;
}
@CustomType.Setter
public Builder disableLocalAuth(@Nullable Boolean disableLocalAuth) {
this.disableLocalAuth = disableLocalAuth;
return this;
}
@CustomType.Setter
public Builder endpoint(String endpoint) {
if (endpoint == null) {
throw new MissingRequiredPropertyException("GetDomainResult", "endpoint");
}
this.endpoint = endpoint;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetDomainResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder identity(@Nullable IdentityInfoResponse identity) {
this.identity = identity;
return this;
}
@CustomType.Setter
public Builder inboundIpRules(@Nullable List inboundIpRules) {
this.inboundIpRules = inboundIpRules;
return this;
}
public Builder inboundIpRules(InboundIpRuleResponse... inboundIpRules) {
return inboundIpRules(List.of(inboundIpRules));
}
@CustomType.Setter
public Builder inputSchema(@Nullable String inputSchema) {
this.inputSchema = inputSchema;
return this;
}
@CustomType.Setter
public Builder inputSchemaMapping(@Nullable JsonInputSchemaMappingResponse inputSchemaMapping) {
this.inputSchemaMapping = inputSchemaMapping;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetDomainResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder metricResourceId(String metricResourceId) {
if (metricResourceId == null) {
throw new MissingRequiredPropertyException("GetDomainResult", "metricResourceId");
}
this.metricResourceId = metricResourceId;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetDomainResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder privateEndpointConnections(List privateEndpointConnections) {
if (privateEndpointConnections == null) {
throw new MissingRequiredPropertyException("GetDomainResult", "privateEndpointConnections");
}
this.privateEndpointConnections = privateEndpointConnections;
return this;
}
public Builder privateEndpointConnections(PrivateEndpointConnectionResponse... privateEndpointConnections) {
return privateEndpointConnections(List.of(privateEndpointConnections));
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetDomainResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder publicNetworkAccess(@Nullable String publicNetworkAccess) {
this.publicNetworkAccess = publicNetworkAccess;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetDomainResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetDomainResult", "type");
}
this.type = type;
return this;
}
public GetDomainResult build() {
final var _resultValue = new GetDomainResult();
_resultValue.autoCreateTopicWithFirstSubscription = autoCreateTopicWithFirstSubscription;
_resultValue.autoDeleteTopicWithLastSubscription = autoDeleteTopicWithLastSubscription;
_resultValue.dataResidencyBoundary = dataResidencyBoundary;
_resultValue.disableLocalAuth = disableLocalAuth;
_resultValue.endpoint = endpoint;
_resultValue.id = id;
_resultValue.identity = identity;
_resultValue.inboundIpRules = inboundIpRules;
_resultValue.inputSchema = inputSchema;
_resultValue.inputSchemaMapping = inputSchemaMapping;
_resultValue.location = location;
_resultValue.metricResourceId = metricResourceId;
_resultValue.name = name;
_resultValue.privateEndpointConnections = privateEndpointConnections;
_resultValue.provisioningState = provisioningState;
_resultValue.publicNetworkAccess = publicNetworkAccess;
_resultValue.systemData = systemData;
_resultValue.tags = tags;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy