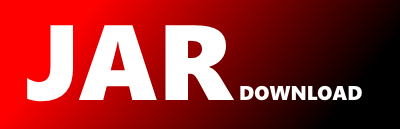
com.pulumi.azurenative.extendedlocation.outputs.GetResourceSyncRuleResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.extendedlocation.outputs;
import com.pulumi.azurenative.extendedlocation.outputs.ResourceSyncRulePropertiesResponseSelector;
import com.pulumi.azurenative.extendedlocation.outputs.SystemDataResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetResourceSyncRuleResult {
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return The geo-location where the resource lives
*
*/
private String location;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return Priority represents a priority of the Resource Sync Rule
*
*/
private @Nullable Integer priority;
/**
* @return Provisioning State for the Resource Sync Rule.
*
*/
private String provisioningState;
/**
* @return A label selector is composed of two parts, matchLabels and matchExpressions. The first part, matchLabels is a map of {key,value} pairs. A single {key,value} in the matchLabels map is equivalent to an element of matchExpressions, whose key field is 'key', the operator is 'In', and the values array contains only 'value'. The second part, matchExpressions is a list of resource selector requirements. Valid operators include In, NotIn, Exists, and DoesNotExist. The values set must be non-empty in the case of In and NotIn. The values set must be empty in the case of Exists and DoesNotExist. All of the requirements, from both matchLabels and matchExpressions must all be satisfied in order to match.
*
*/
private @Nullable ResourceSyncRulePropertiesResponseSelector selector;
/**
* @return Metadata pertaining to creation and last modification of the resource
*
*/
private SystemDataResponse systemData;
/**
* @return Resource tags.
*
*/
private @Nullable Map tags;
/**
* @return For an unmapped custom resource, its labels will be used to find matching resource sync rules. If this resource sync rule is one of the matching rules with highest priority, then the unmapped custom resource will be projected to the target resource group associated with this resource sync rule. The user creating this resource sync rule should have write permissions on the target resource group and this write permission will be validated when creating the resource sync rule.
*
*/
private @Nullable String targetResourceGroup;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
private GetResourceSyncRuleResult() {}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return The geo-location where the resource lives
*
*/
public String location() {
return this.location;
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return Priority represents a priority of the Resource Sync Rule
*
*/
public Optional priority() {
return Optional.ofNullable(this.priority);
}
/**
* @return Provisioning State for the Resource Sync Rule.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return A label selector is composed of two parts, matchLabels and matchExpressions. The first part, matchLabels is a map of {key,value} pairs. A single {key,value} in the matchLabels map is equivalent to an element of matchExpressions, whose key field is 'key', the operator is 'In', and the values array contains only 'value'. The second part, matchExpressions is a list of resource selector requirements. Valid operators include In, NotIn, Exists, and DoesNotExist. The values set must be non-empty in the case of In and NotIn. The values set must be empty in the case of Exists and DoesNotExist. All of the requirements, from both matchLabels and matchExpressions must all be satisfied in order to match.
*
*/
public Optional selector() {
return Optional.ofNullable(this.selector);
}
/**
* @return Metadata pertaining to creation and last modification of the resource
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return Resource tags.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return For an unmapped custom resource, its labels will be used to find matching resource sync rules. If this resource sync rule is one of the matching rules with highest priority, then the unmapped custom resource will be projected to the target resource group associated with this resource sync rule. The user creating this resource sync rule should have write permissions on the target resource group and this write permission will be validated when creating the resource sync rule.
*
*/
public Optional targetResourceGroup() {
return Optional.ofNullable(this.targetResourceGroup);
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetResourceSyncRuleResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String id;
private String location;
private String name;
private @Nullable Integer priority;
private String provisioningState;
private @Nullable ResourceSyncRulePropertiesResponseSelector selector;
private SystemDataResponse systemData;
private @Nullable Map tags;
private @Nullable String targetResourceGroup;
private String type;
public Builder() {}
public Builder(GetResourceSyncRuleResult defaults) {
Objects.requireNonNull(defaults);
this.id = defaults.id;
this.location = defaults.location;
this.name = defaults.name;
this.priority = defaults.priority;
this.provisioningState = defaults.provisioningState;
this.selector = defaults.selector;
this.systemData = defaults.systemData;
this.tags = defaults.tags;
this.targetResourceGroup = defaults.targetResourceGroup;
this.type = defaults.type;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetResourceSyncRuleResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetResourceSyncRuleResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetResourceSyncRuleResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder priority(@Nullable Integer priority) {
this.priority = priority;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetResourceSyncRuleResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder selector(@Nullable ResourceSyncRulePropertiesResponseSelector selector) {
this.selector = selector;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetResourceSyncRuleResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder targetResourceGroup(@Nullable String targetResourceGroup) {
this.targetResourceGroup = targetResourceGroup;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetResourceSyncRuleResult", "type");
}
this.type = type;
return this;
}
public GetResourceSyncRuleResult build() {
final var _resultValue = new GetResourceSyncRuleResult();
_resultValue.id = id;
_resultValue.location = location;
_resultValue.name = name;
_resultValue.priority = priority;
_resultValue.provisioningState = provisioningState;
_resultValue.selector = selector;
_resultValue.systemData = systemData;
_resultValue.tags = tags;
_resultValue.targetResourceGroup = targetResourceGroup;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy