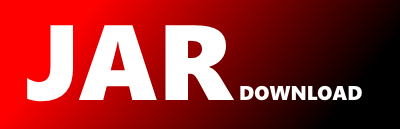
com.pulumi.azurenative.hdinsight.inputs.RoleArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.hdinsight.inputs;
import com.pulumi.azurenative.hdinsight.inputs.AutoscaleArgs;
import com.pulumi.azurenative.hdinsight.inputs.DataDisksGroupsArgs;
import com.pulumi.azurenative.hdinsight.inputs.HardwareProfileArgs;
import com.pulumi.azurenative.hdinsight.inputs.OsProfileArgs;
import com.pulumi.azurenative.hdinsight.inputs.ScriptActionArgs;
import com.pulumi.azurenative.hdinsight.inputs.VirtualNetworkProfileArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Describes a role on the cluster.
*
*/
public final class RoleArgs extends com.pulumi.resources.ResourceArgs {
public static final RoleArgs Empty = new RoleArgs();
/**
* The autoscale configurations.
*
*/
@Import(name="autoscaleConfiguration")
private @Nullable Output autoscaleConfiguration;
/**
* @return The autoscale configurations.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy