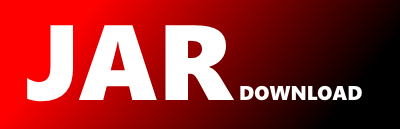
com.pulumi.azurenative.hdinsight.inputs.SecurityProfileArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.hdinsight.inputs;
import com.pulumi.azurenative.hdinsight.enums.DirectoryType;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* The security profile which contains Ssh public key for the HDInsight cluster.
*
*/
public final class SecurityProfileArgs extends com.pulumi.resources.ResourceArgs {
public static final SecurityProfileArgs Empty = new SecurityProfileArgs();
/**
* The resource ID of the user's Azure Active Directory Domain Service.
*
*/
@Import(name="aaddsResourceId")
private @Nullable Output aaddsResourceId;
/**
* @return The resource ID of the user's Azure Active Directory Domain Service.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy