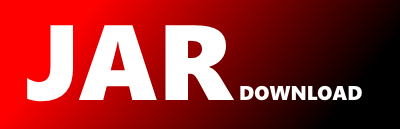
com.pulumi.azurenative.hybridcompute.MachineRunCommand Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.hybridcompute;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.hybridcompute.MachineRunCommandArgs;
import com.pulumi.azurenative.hybridcompute.outputs.MachineRunCommandInstanceViewResponse;
import com.pulumi.azurenative.hybridcompute.outputs.MachineRunCommandScriptSourceResponse;
import com.pulumi.azurenative.hybridcompute.outputs.RunCommandInputParameterResponse;
import com.pulumi.azurenative.hybridcompute.outputs.RunCommandManagedIdentityResponse;
import com.pulumi.azurenative.hybridcompute.outputs.SystemDataResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Describes a Run Command
* Azure REST API version: 2023-10-03-preview.
*
* Other available API versions: 2024-03-31-preview, 2024-05-20-preview, 2024-07-31-preview.
*
* ## Example Usage
* ### Create or Update a Run Command
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.hybridcompute.MachineRunCommand;
* import com.pulumi.azurenative.hybridcompute.MachineRunCommandArgs;
* import com.pulumi.azurenative.hybridcompute.inputs.RunCommandInputParameterArgs;
* import com.pulumi.azurenative.hybridcompute.inputs.MachineRunCommandScriptSourceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var machineRunCommand = new MachineRunCommand("machineRunCommand", MachineRunCommandArgs.builder()
* .asyncExecution(false)
* .errorBlobUri("https://mystorageaccount.blob.core.windows.net/mycontainer/MyScriptError.txt")
* .location("eastus2")
* .machineName("myMachine")
* .outputBlobUri("https://mystorageaccount.blob.core.windows.net/myscriptoutputcontainer/MyScriptoutput.txt")
* .parameters(
* RunCommandInputParameterArgs.builder()
* .name("param1")
* .value("value1")
* .build(),
* RunCommandInputParameterArgs.builder()
* .name("param2")
* .value("value2")
* .build())
* .resourceGroupName("myResourceGroup")
* .runAsPassword("")
* .runAsUser("user1")
* .runCommandName("myRunCommand")
* .source(MachineRunCommandScriptSourceArgs.builder()
* .script("Write-Host Hello World!")
* .build())
* .timeoutInSeconds(3600)
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:hybridcompute:MachineRunCommand myRunCommand /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.HybridCompute/machines/{machineName}/runCommands/{runCommandName}
* ```
*
*/
@ResourceType(type="azure-native:hybridcompute:MachineRunCommand")
public class MachineRunCommand extends com.pulumi.resources.CustomResource {
/**
* Optional. If set to true, provisioning will complete as soon as script starts and will not wait for script to complete.
*
*/
@Export(name="asyncExecution", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> asyncExecution;
/**
* @return Optional. If set to true, provisioning will complete as soon as script starts and will not wait for script to complete.
*
*/
public Output> asyncExecution() {
return Codegen.optional(this.asyncExecution);
}
/**
* User-assigned managed identity that has access to errorBlobUri storage blob. Use an empty object in case of system-assigned identity. Make sure managed identity has been given access to blob's container with 'Storage Blob Data Contributor' role assignment. In case of user-assigned identity, make sure you add it under VM's identity. For more info on managed identity and Run Command, refer https://aka.ms/ManagedIdentity and https://aka.ms/RunCommandManaged
*
*/
@Export(name="errorBlobManagedIdentity", refs={RunCommandManagedIdentityResponse.class}, tree="[0]")
private Output* @Nullable */ RunCommandManagedIdentityResponse> errorBlobManagedIdentity;
/**
* @return User-assigned managed identity that has access to errorBlobUri storage blob. Use an empty object in case of system-assigned identity. Make sure managed identity has been given access to blob's container with 'Storage Blob Data Contributor' role assignment. In case of user-assigned identity, make sure you add it under VM's identity. For more info on managed identity and Run Command, refer https://aka.ms/ManagedIdentity and https://aka.ms/RunCommandManaged
*
*/
public Output> errorBlobManagedIdentity() {
return Codegen.optional(this.errorBlobManagedIdentity);
}
/**
* Specifies the Azure storage blob where script error stream will be uploaded. Use a SAS URI with read, append, create, write access OR use managed identity to provide the VM access to the blob. Refer errorBlobManagedIdentity parameter.
*
*/
@Export(name="errorBlobUri", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> errorBlobUri;
/**
* @return Specifies the Azure storage blob where script error stream will be uploaded. Use a SAS URI with read, append, create, write access OR use managed identity to provide the VM access to the blob. Refer errorBlobManagedIdentity parameter.
*
*/
public Output> errorBlobUri() {
return Codegen.optional(this.errorBlobUri);
}
/**
* The machine run command instance view.
*
*/
@Export(name="instanceView", refs={MachineRunCommandInstanceViewResponse.class}, tree="[0]")
private Output instanceView;
/**
* @return The machine run command instance view.
*
*/
public Output instanceView() {
return this.instanceView;
}
/**
* The geo-location where the resource lives
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output location;
/**
* @return The geo-location where the resource lives
*
*/
public Output location() {
return this.location;
}
/**
* The name of the resource
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the resource
*
*/
public Output name() {
return this.name;
}
/**
* User-assigned managed identity that has access to outputBlobUri storage blob. Use an empty object in case of system-assigned identity. Make sure managed identity has been given access to blob's container with 'Storage Blob Data Contributor' role assignment. In case of user-assigned identity, make sure you add it under VM's identity. For more info on managed identity and Run Command, refer https://aka.ms/ManagedIdentity and https://aka.ms/RunCommandManaged
*
*/
@Export(name="outputBlobManagedIdentity", refs={RunCommandManagedIdentityResponse.class}, tree="[0]")
private Output* @Nullable */ RunCommandManagedIdentityResponse> outputBlobManagedIdentity;
/**
* @return User-assigned managed identity that has access to outputBlobUri storage blob. Use an empty object in case of system-assigned identity. Make sure managed identity has been given access to blob's container with 'Storage Blob Data Contributor' role assignment. In case of user-assigned identity, make sure you add it under VM's identity. For more info on managed identity and Run Command, refer https://aka.ms/ManagedIdentity and https://aka.ms/RunCommandManaged
*
*/
public Output> outputBlobManagedIdentity() {
return Codegen.optional(this.outputBlobManagedIdentity);
}
/**
* Specifies the Azure storage blob where script output stream will be uploaded. Use a SAS URI with read, append, create, write access OR use managed identity to provide the VM access to the blob. Refer outputBlobManagedIdentity parameter.
*
*/
@Export(name="outputBlobUri", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> outputBlobUri;
/**
* @return Specifies the Azure storage blob where script output stream will be uploaded. Use a SAS URI with read, append, create, write access OR use managed identity to provide the VM access to the blob. Refer outputBlobManagedIdentity parameter.
*
*/
public Output> outputBlobUri() {
return Codegen.optional(this.outputBlobUri);
}
/**
* The parameters used by the script.
*
*/
@Export(name="parameters", refs={List.class,RunCommandInputParameterResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> parameters;
/**
* @return The parameters used by the script.
*
*/
public Output>> parameters() {
return Codegen.optional(this.parameters);
}
/**
* The parameters used by the script.
*
*/
@Export(name="protectedParameters", refs={List.class,RunCommandInputParameterResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> protectedParameters;
/**
* @return The parameters used by the script.
*
*/
public Output>> protectedParameters() {
return Codegen.optional(this.protectedParameters);
}
/**
* The provisioning state, which only appears in the response.
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return The provisioning state, which only appears in the response.
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* Specifies the user account password on the machine when executing the run command.
*
*/
@Export(name="runAsPassword", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> runAsPassword;
/**
* @return Specifies the user account password on the machine when executing the run command.
*
*/
public Output> runAsPassword() {
return Codegen.optional(this.runAsPassword);
}
/**
* Specifies the user account on the machine when executing the run command.
*
*/
@Export(name="runAsUser", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> runAsUser;
/**
* @return Specifies the user account on the machine when executing the run command.
*
*/
public Output> runAsUser() {
return Codegen.optional(this.runAsUser);
}
/**
* The source of the run command script.
*
*/
@Export(name="source", refs={MachineRunCommandScriptSourceResponse.class}, tree="[0]")
private Output* @Nullable */ MachineRunCommandScriptSourceResponse> source;
/**
* @return The source of the run command script.
*
*/
public Output> source() {
return Codegen.optional(this.source);
}
/**
* Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
@Export(name="systemData", refs={SystemDataResponse.class}, tree="[0]")
private Output systemData;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public Output systemData() {
return this.systemData;
}
/**
* Resource tags.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Resource tags.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* The timeout in seconds to execute the run command.
*
*/
@Export(name="timeoutInSeconds", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> timeoutInSeconds;
/**
* @return The timeout in seconds to execute the run command.
*
*/
public Output> timeoutInSeconds() {
return Codegen.optional(this.timeoutInSeconds);
}
/**
* The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public Output type() {
return this.type;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public MachineRunCommand(java.lang.String name) {
this(name, MachineRunCommandArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public MachineRunCommand(java.lang.String name, MachineRunCommandArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public MachineRunCommand(java.lang.String name, MachineRunCommandArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:hybridcompute:MachineRunCommand", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private MachineRunCommand(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:hybridcompute:MachineRunCommand", name, null, makeResourceOptions(options, id), false);
}
private static MachineRunCommandArgs makeArgs(MachineRunCommandArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? MachineRunCommandArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:hybridcompute/v20231003preview:MachineRunCommand").build()),
Output.of(Alias.builder().type("azure-native:hybridcompute/v20240331preview:MachineRunCommand").build()),
Output.of(Alias.builder().type("azure-native:hybridcompute/v20240520preview:MachineRunCommand").build()),
Output.of(Alias.builder().type("azure-native:hybridcompute/v20240731preview:MachineRunCommand").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static MachineRunCommand get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new MachineRunCommand(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy