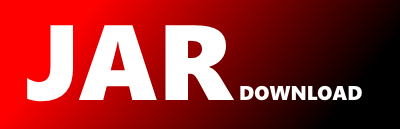
com.pulumi.azurenative.hybridcompute.outputs.AgentConfigurationResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.hybridcompute.outputs;
import com.pulumi.azurenative.hybridcompute.outputs.ConfigurationExtensionResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class AgentConfigurationResponse {
/**
* @return Name of configuration mode to use. Modes are pre-defined configurations of security controls, extension allowlists and guest configuration, maintained by Microsoft.
*
*/
private String configMode;
/**
* @return Array of extensions that are allowed to be installed or updated.
*
*/
private List extensionsAllowList;
/**
* @return Array of extensions that are blocked (cannot be installed or updated)
*
*/
private List extensionsBlockList;
/**
* @return Specifies whether the extension service is enabled or disabled.
*
*/
private String extensionsEnabled;
/**
* @return Specified whether the guest configuration service is enabled or disabled.
*
*/
private String guestConfigurationEnabled;
/**
* @return Specifies the list of ports that the agent will be able to listen on.
*
*/
private List incomingConnectionsPorts;
/**
* @return List of service names which should not use the specified proxy server.
*
*/
private List proxyBypass;
/**
* @return Specifies the URL of the proxy to be used.
*
*/
private String proxyUrl;
private AgentConfigurationResponse() {}
/**
* @return Name of configuration mode to use. Modes are pre-defined configurations of security controls, extension allowlists and guest configuration, maintained by Microsoft.
*
*/
public String configMode() {
return this.configMode;
}
/**
* @return Array of extensions that are allowed to be installed or updated.
*
*/
public List extensionsAllowList() {
return this.extensionsAllowList;
}
/**
* @return Array of extensions that are blocked (cannot be installed or updated)
*
*/
public List extensionsBlockList() {
return this.extensionsBlockList;
}
/**
* @return Specifies whether the extension service is enabled or disabled.
*
*/
public String extensionsEnabled() {
return this.extensionsEnabled;
}
/**
* @return Specified whether the guest configuration service is enabled or disabled.
*
*/
public String guestConfigurationEnabled() {
return this.guestConfigurationEnabled;
}
/**
* @return Specifies the list of ports that the agent will be able to listen on.
*
*/
public List incomingConnectionsPorts() {
return this.incomingConnectionsPorts;
}
/**
* @return List of service names which should not use the specified proxy server.
*
*/
public List proxyBypass() {
return this.proxyBypass;
}
/**
* @return Specifies the URL of the proxy to be used.
*
*/
public String proxyUrl() {
return this.proxyUrl;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(AgentConfigurationResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String configMode;
private List extensionsAllowList;
private List extensionsBlockList;
private String extensionsEnabled;
private String guestConfigurationEnabled;
private List incomingConnectionsPorts;
private List proxyBypass;
private String proxyUrl;
public Builder() {}
public Builder(AgentConfigurationResponse defaults) {
Objects.requireNonNull(defaults);
this.configMode = defaults.configMode;
this.extensionsAllowList = defaults.extensionsAllowList;
this.extensionsBlockList = defaults.extensionsBlockList;
this.extensionsEnabled = defaults.extensionsEnabled;
this.guestConfigurationEnabled = defaults.guestConfigurationEnabled;
this.incomingConnectionsPorts = defaults.incomingConnectionsPorts;
this.proxyBypass = defaults.proxyBypass;
this.proxyUrl = defaults.proxyUrl;
}
@CustomType.Setter
public Builder configMode(String configMode) {
if (configMode == null) {
throw new MissingRequiredPropertyException("AgentConfigurationResponse", "configMode");
}
this.configMode = configMode;
return this;
}
@CustomType.Setter
public Builder extensionsAllowList(List extensionsAllowList) {
if (extensionsAllowList == null) {
throw new MissingRequiredPropertyException("AgentConfigurationResponse", "extensionsAllowList");
}
this.extensionsAllowList = extensionsAllowList;
return this;
}
public Builder extensionsAllowList(ConfigurationExtensionResponse... extensionsAllowList) {
return extensionsAllowList(List.of(extensionsAllowList));
}
@CustomType.Setter
public Builder extensionsBlockList(List extensionsBlockList) {
if (extensionsBlockList == null) {
throw new MissingRequiredPropertyException("AgentConfigurationResponse", "extensionsBlockList");
}
this.extensionsBlockList = extensionsBlockList;
return this;
}
public Builder extensionsBlockList(ConfigurationExtensionResponse... extensionsBlockList) {
return extensionsBlockList(List.of(extensionsBlockList));
}
@CustomType.Setter
public Builder extensionsEnabled(String extensionsEnabled) {
if (extensionsEnabled == null) {
throw new MissingRequiredPropertyException("AgentConfigurationResponse", "extensionsEnabled");
}
this.extensionsEnabled = extensionsEnabled;
return this;
}
@CustomType.Setter
public Builder guestConfigurationEnabled(String guestConfigurationEnabled) {
if (guestConfigurationEnabled == null) {
throw new MissingRequiredPropertyException("AgentConfigurationResponse", "guestConfigurationEnabled");
}
this.guestConfigurationEnabled = guestConfigurationEnabled;
return this;
}
@CustomType.Setter
public Builder incomingConnectionsPorts(List incomingConnectionsPorts) {
if (incomingConnectionsPorts == null) {
throw new MissingRequiredPropertyException("AgentConfigurationResponse", "incomingConnectionsPorts");
}
this.incomingConnectionsPorts = incomingConnectionsPorts;
return this;
}
public Builder incomingConnectionsPorts(String... incomingConnectionsPorts) {
return incomingConnectionsPorts(List.of(incomingConnectionsPorts));
}
@CustomType.Setter
public Builder proxyBypass(List proxyBypass) {
if (proxyBypass == null) {
throw new MissingRequiredPropertyException("AgentConfigurationResponse", "proxyBypass");
}
this.proxyBypass = proxyBypass;
return this;
}
public Builder proxyBypass(String... proxyBypass) {
return proxyBypass(List.of(proxyBypass));
}
@CustomType.Setter
public Builder proxyUrl(String proxyUrl) {
if (proxyUrl == null) {
throw new MissingRequiredPropertyException("AgentConfigurationResponse", "proxyUrl");
}
this.proxyUrl = proxyUrl;
return this;
}
public AgentConfigurationResponse build() {
final var _resultValue = new AgentConfigurationResponse();
_resultValue.configMode = configMode;
_resultValue.extensionsAllowList = extensionsAllowList;
_resultValue.extensionsBlockList = extensionsBlockList;
_resultValue.extensionsEnabled = extensionsEnabled;
_resultValue.guestConfigurationEnabled = guestConfigurationEnabled;
_resultValue.incomingConnectionsPorts = incomingConnectionsPorts;
_resultValue.proxyBypass = proxyBypass;
_resultValue.proxyUrl = proxyUrl;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy