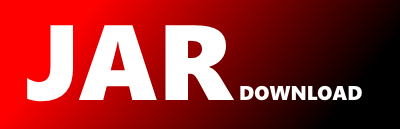
com.pulumi.azurenative.hybridcontainerservice.outputs.NetworkProfileResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.hybridcontainerservice.outputs;
import com.pulumi.azurenative.hybridcontainerservice.outputs.LoadBalancerProfileResponse;
import com.pulumi.core.annotations.CustomType;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class NetworkProfileResponse {
/**
* @return DNSServiceIP - An IP address assigned to the Kubernetes DNS service. It must be within the Kubernetes service address range specified in serviceCidr.
*
*/
private @Nullable String dnsServiceIP;
/**
* @return LoadBalancerProfile - Profile of the cluster load balancer.
*
*/
private @Nullable LoadBalancerProfileResponse loadBalancerProfile;
/**
* @return LoadBalancerSku - The load balancer sku for the provisioned cluster. Possible values: 'unstacked-haproxy', 'stacked-kube-vip', 'stacked-metallb', 'unmanaged'. The default is 'unmanaged'.
*
*/
private @Nullable String loadBalancerSku;
/**
* @return NetworkPolicy - Network policy used for building Kubernetes network. Possible values include: 'calico', 'flannel'. Default is 'calico'
*
*/
private @Nullable String networkPolicy;
/**
* @return PodCidr - A CIDR notation IP range from which to assign pod IPs when kubenet is used.
*
*/
private @Nullable String podCidr;
/**
* @return The CIDR notation IP ranges from which to assign pod IPs. One IPv4 CIDR is expected for single-stack networking. Two CIDRs, one for each IP family (IPv4/IPv6), is expected for dual-stack networking.
*
*/
private @Nullable List podCidrs;
/**
* @return ServiceCidr - A CIDR notation IP range from which to assign service cluster IPs. It must not overlap with any Subnet IP ranges.
*
*/
private @Nullable String serviceCidr;
/**
* @return The CIDR notation IP ranges from which to assign service cluster IPs. One IPv4 CIDR is expected for single-stack networking. Two CIDRs, one for each IP family (IPv4/IPv6), is expected for dual-stack networking. They must not overlap with any Subnet IP ranges.
*
*/
private @Nullable List serviceCidrs;
private NetworkProfileResponse() {}
/**
* @return DNSServiceIP - An IP address assigned to the Kubernetes DNS service. It must be within the Kubernetes service address range specified in serviceCidr.
*
*/
public Optional dnsServiceIP() {
return Optional.ofNullable(this.dnsServiceIP);
}
/**
* @return LoadBalancerProfile - Profile of the cluster load balancer.
*
*/
public Optional loadBalancerProfile() {
return Optional.ofNullable(this.loadBalancerProfile);
}
/**
* @return LoadBalancerSku - The load balancer sku for the provisioned cluster. Possible values: 'unstacked-haproxy', 'stacked-kube-vip', 'stacked-metallb', 'unmanaged'. The default is 'unmanaged'.
*
*/
public Optional loadBalancerSku() {
return Optional.ofNullable(this.loadBalancerSku);
}
/**
* @return NetworkPolicy - Network policy used for building Kubernetes network. Possible values include: 'calico', 'flannel'. Default is 'calico'
*
*/
public Optional networkPolicy() {
return Optional.ofNullable(this.networkPolicy);
}
/**
* @return PodCidr - A CIDR notation IP range from which to assign pod IPs when kubenet is used.
*
*/
public Optional podCidr() {
return Optional.ofNullable(this.podCidr);
}
/**
* @return The CIDR notation IP ranges from which to assign pod IPs. One IPv4 CIDR is expected for single-stack networking. Two CIDRs, one for each IP family (IPv4/IPv6), is expected for dual-stack networking.
*
*/
public List podCidrs() {
return this.podCidrs == null ? List.of() : this.podCidrs;
}
/**
* @return ServiceCidr - A CIDR notation IP range from which to assign service cluster IPs. It must not overlap with any Subnet IP ranges.
*
*/
public Optional serviceCidr() {
return Optional.ofNullable(this.serviceCidr);
}
/**
* @return The CIDR notation IP ranges from which to assign service cluster IPs. One IPv4 CIDR is expected for single-stack networking. Two CIDRs, one for each IP family (IPv4/IPv6), is expected for dual-stack networking. They must not overlap with any Subnet IP ranges.
*
*/
public List serviceCidrs() {
return this.serviceCidrs == null ? List.of() : this.serviceCidrs;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(NetworkProfileResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String dnsServiceIP;
private @Nullable LoadBalancerProfileResponse loadBalancerProfile;
private @Nullable String loadBalancerSku;
private @Nullable String networkPolicy;
private @Nullable String podCidr;
private @Nullable List podCidrs;
private @Nullable String serviceCidr;
private @Nullable List serviceCidrs;
public Builder() {}
public Builder(NetworkProfileResponse defaults) {
Objects.requireNonNull(defaults);
this.dnsServiceIP = defaults.dnsServiceIP;
this.loadBalancerProfile = defaults.loadBalancerProfile;
this.loadBalancerSku = defaults.loadBalancerSku;
this.networkPolicy = defaults.networkPolicy;
this.podCidr = defaults.podCidr;
this.podCidrs = defaults.podCidrs;
this.serviceCidr = defaults.serviceCidr;
this.serviceCidrs = defaults.serviceCidrs;
}
@CustomType.Setter
public Builder dnsServiceIP(@Nullable String dnsServiceIP) {
this.dnsServiceIP = dnsServiceIP;
return this;
}
@CustomType.Setter
public Builder loadBalancerProfile(@Nullable LoadBalancerProfileResponse loadBalancerProfile) {
this.loadBalancerProfile = loadBalancerProfile;
return this;
}
@CustomType.Setter
public Builder loadBalancerSku(@Nullable String loadBalancerSku) {
this.loadBalancerSku = loadBalancerSku;
return this;
}
@CustomType.Setter
public Builder networkPolicy(@Nullable String networkPolicy) {
this.networkPolicy = networkPolicy;
return this;
}
@CustomType.Setter
public Builder podCidr(@Nullable String podCidr) {
this.podCidr = podCidr;
return this;
}
@CustomType.Setter
public Builder podCidrs(@Nullable List podCidrs) {
this.podCidrs = podCidrs;
return this;
}
public Builder podCidrs(String... podCidrs) {
return podCidrs(List.of(podCidrs));
}
@CustomType.Setter
public Builder serviceCidr(@Nullable String serviceCidr) {
this.serviceCidr = serviceCidr;
return this;
}
@CustomType.Setter
public Builder serviceCidrs(@Nullable List serviceCidrs) {
this.serviceCidrs = serviceCidrs;
return this;
}
public Builder serviceCidrs(String... serviceCidrs) {
return serviceCidrs(List.of(serviceCidrs));
}
public NetworkProfileResponse build() {
final var _resultValue = new NetworkProfileResponse();
_resultValue.dnsServiceIP = dnsServiceIP;
_resultValue.loadBalancerProfile = loadBalancerProfile;
_resultValue.loadBalancerSku = loadBalancerSku;
_resultValue.networkPolicy = networkPolicy;
_resultValue.podCidr = podCidr;
_resultValue.podCidrs = podCidrs;
_resultValue.serviceCidr = serviceCidr;
_resultValue.serviceCidrs = serviceCidrs;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy