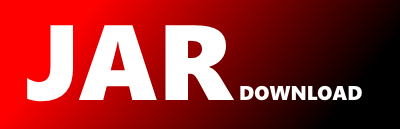
com.pulumi.azurenative.importexport.outputs.JobDetailsResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.importexport.outputs;
import com.pulumi.azurenative.importexport.outputs.DeliveryPackageInformationResponse;
import com.pulumi.azurenative.importexport.outputs.DriveStatusResponse;
import com.pulumi.azurenative.importexport.outputs.EncryptionKeyDetailsResponse;
import com.pulumi.azurenative.importexport.outputs.ExportResponse;
import com.pulumi.azurenative.importexport.outputs.PackageInformationResponse;
import com.pulumi.azurenative.importexport.outputs.ReturnAddressResponse;
import com.pulumi.azurenative.importexport.outputs.ReturnShippingResponse;
import com.pulumi.azurenative.importexport.outputs.ShippingInformationResponse;
import com.pulumi.core.annotations.CustomType;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class JobDetailsResponse {
/**
* @return Default value is false. Indicates whether the manifest files on the drives should be copied to block blobs.
*
*/
private @Nullable Boolean backupDriveManifest;
/**
* @return Indicates whether a request has been submitted to cancel the job.
*
*/
private @Nullable Boolean cancelRequested;
/**
* @return Contains information about the package being shipped by the customer to the Microsoft data center.
*
*/
private @Nullable DeliveryPackageInformationResponse deliveryPackage;
/**
* @return The virtual blob directory to which the copy logs and backups of drive manifest files (if enabled) will be stored.
*
*/
private @Nullable String diagnosticsPath;
/**
* @return List of up to ten drives that comprise the job. The drive list is a required element for an import job; it is not specified for export jobs.
*
*/
private @Nullable List driveList;
/**
* @return Contains information about the encryption key.
*
*/
private @Nullable EncryptionKeyDetailsResponse encryptionKey;
/**
* @return A property containing information about the blobs to be exported for an export job. This property is included for export jobs only.
*
*/
private @Nullable ExportResponse export;
/**
* @return A blob path that points to a block blob containing a list of blob names that were not exported due to insufficient drive space. If all blobs were exported successfully, then this element is not included in the response.
*
*/
private @Nullable String incompleteBlobListUri;
/**
* @return The type of job
*
*/
private @Nullable String jobType;
/**
* @return Default value is Error. Indicates whether error logging or verbose logging will be enabled.
*
*/
private @Nullable String logLevel;
/**
* @return Overall percentage completed for the job.
*
*/
private @Nullable Double percentComplete;
/**
* @return Specifies the provisioning state of the job.
*
*/
private @Nullable String provisioningState;
/**
* @return Specifies the return address information for the job.
*
*/
private @Nullable ReturnAddressResponse returnAddress;
/**
* @return Contains information about the package being shipped from the Microsoft data center to the customer to return the drives. The format is the same as the deliveryPackage property above. This property is not included if the drives have not yet been returned.
*
*/
private @Nullable PackageInformationResponse returnPackage;
/**
* @return Specifies the return carrier and customer's account with the carrier.
*
*/
private @Nullable ReturnShippingResponse returnShipping;
/**
* @return Contains information about the Microsoft datacenter to which the drives should be shipped.
*
*/
private @Nullable ShippingInformationResponse shippingInformation;
/**
* @return Current state of the job.
*
*/
private @Nullable String state;
/**
* @return The resource identifier of the storage account where data will be imported to or exported from.
*
*/
private @Nullable String storageAccountId;
private JobDetailsResponse() {}
/**
* @return Default value is false. Indicates whether the manifest files on the drives should be copied to block blobs.
*
*/
public Optional backupDriveManifest() {
return Optional.ofNullable(this.backupDriveManifest);
}
/**
* @return Indicates whether a request has been submitted to cancel the job.
*
*/
public Optional cancelRequested() {
return Optional.ofNullable(this.cancelRequested);
}
/**
* @return Contains information about the package being shipped by the customer to the Microsoft data center.
*
*/
public Optional deliveryPackage() {
return Optional.ofNullable(this.deliveryPackage);
}
/**
* @return The virtual blob directory to which the copy logs and backups of drive manifest files (if enabled) will be stored.
*
*/
public Optional diagnosticsPath() {
return Optional.ofNullable(this.diagnosticsPath);
}
/**
* @return List of up to ten drives that comprise the job. The drive list is a required element for an import job; it is not specified for export jobs.
*
*/
public List driveList() {
return this.driveList == null ? List.of() : this.driveList;
}
/**
* @return Contains information about the encryption key.
*
*/
public Optional encryptionKey() {
return Optional.ofNullable(this.encryptionKey);
}
/**
* @return A property containing information about the blobs to be exported for an export job. This property is included for export jobs only.
*
*/
public Optional export() {
return Optional.ofNullable(this.export);
}
/**
* @return A blob path that points to a block blob containing a list of blob names that were not exported due to insufficient drive space. If all blobs were exported successfully, then this element is not included in the response.
*
*/
public Optional incompleteBlobListUri() {
return Optional.ofNullable(this.incompleteBlobListUri);
}
/**
* @return The type of job
*
*/
public Optional jobType() {
return Optional.ofNullable(this.jobType);
}
/**
* @return Default value is Error. Indicates whether error logging or verbose logging will be enabled.
*
*/
public Optional logLevel() {
return Optional.ofNullable(this.logLevel);
}
/**
* @return Overall percentage completed for the job.
*
*/
public Optional percentComplete() {
return Optional.ofNullable(this.percentComplete);
}
/**
* @return Specifies the provisioning state of the job.
*
*/
public Optional provisioningState() {
return Optional.ofNullable(this.provisioningState);
}
/**
* @return Specifies the return address information for the job.
*
*/
public Optional returnAddress() {
return Optional.ofNullable(this.returnAddress);
}
/**
* @return Contains information about the package being shipped from the Microsoft data center to the customer to return the drives. The format is the same as the deliveryPackage property above. This property is not included if the drives have not yet been returned.
*
*/
public Optional returnPackage() {
return Optional.ofNullable(this.returnPackage);
}
/**
* @return Specifies the return carrier and customer's account with the carrier.
*
*/
public Optional returnShipping() {
return Optional.ofNullable(this.returnShipping);
}
/**
* @return Contains information about the Microsoft datacenter to which the drives should be shipped.
*
*/
public Optional shippingInformation() {
return Optional.ofNullable(this.shippingInformation);
}
/**
* @return Current state of the job.
*
*/
public Optional state() {
return Optional.ofNullable(this.state);
}
/**
* @return The resource identifier of the storage account where data will be imported to or exported from.
*
*/
public Optional storageAccountId() {
return Optional.ofNullable(this.storageAccountId);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(JobDetailsResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Boolean backupDriveManifest;
private @Nullable Boolean cancelRequested;
private @Nullable DeliveryPackageInformationResponse deliveryPackage;
private @Nullable String diagnosticsPath;
private @Nullable List driveList;
private @Nullable EncryptionKeyDetailsResponse encryptionKey;
private @Nullable ExportResponse export;
private @Nullable String incompleteBlobListUri;
private @Nullable String jobType;
private @Nullable String logLevel;
private @Nullable Double percentComplete;
private @Nullable String provisioningState;
private @Nullable ReturnAddressResponse returnAddress;
private @Nullable PackageInformationResponse returnPackage;
private @Nullable ReturnShippingResponse returnShipping;
private @Nullable ShippingInformationResponse shippingInformation;
private @Nullable String state;
private @Nullable String storageAccountId;
public Builder() {}
public Builder(JobDetailsResponse defaults) {
Objects.requireNonNull(defaults);
this.backupDriveManifest = defaults.backupDriveManifest;
this.cancelRequested = defaults.cancelRequested;
this.deliveryPackage = defaults.deliveryPackage;
this.diagnosticsPath = defaults.diagnosticsPath;
this.driveList = defaults.driveList;
this.encryptionKey = defaults.encryptionKey;
this.export = defaults.export;
this.incompleteBlobListUri = defaults.incompleteBlobListUri;
this.jobType = defaults.jobType;
this.logLevel = defaults.logLevel;
this.percentComplete = defaults.percentComplete;
this.provisioningState = defaults.provisioningState;
this.returnAddress = defaults.returnAddress;
this.returnPackage = defaults.returnPackage;
this.returnShipping = defaults.returnShipping;
this.shippingInformation = defaults.shippingInformation;
this.state = defaults.state;
this.storageAccountId = defaults.storageAccountId;
}
@CustomType.Setter
public Builder backupDriveManifest(@Nullable Boolean backupDriveManifest) {
this.backupDriveManifest = backupDriveManifest;
return this;
}
@CustomType.Setter
public Builder cancelRequested(@Nullable Boolean cancelRequested) {
this.cancelRequested = cancelRequested;
return this;
}
@CustomType.Setter
public Builder deliveryPackage(@Nullable DeliveryPackageInformationResponse deliveryPackage) {
this.deliveryPackage = deliveryPackage;
return this;
}
@CustomType.Setter
public Builder diagnosticsPath(@Nullable String diagnosticsPath) {
this.diagnosticsPath = diagnosticsPath;
return this;
}
@CustomType.Setter
public Builder driveList(@Nullable List driveList) {
this.driveList = driveList;
return this;
}
public Builder driveList(DriveStatusResponse... driveList) {
return driveList(List.of(driveList));
}
@CustomType.Setter
public Builder encryptionKey(@Nullable EncryptionKeyDetailsResponse encryptionKey) {
this.encryptionKey = encryptionKey;
return this;
}
@CustomType.Setter
public Builder export(@Nullable ExportResponse export) {
this.export = export;
return this;
}
@CustomType.Setter
public Builder incompleteBlobListUri(@Nullable String incompleteBlobListUri) {
this.incompleteBlobListUri = incompleteBlobListUri;
return this;
}
@CustomType.Setter
public Builder jobType(@Nullable String jobType) {
this.jobType = jobType;
return this;
}
@CustomType.Setter
public Builder logLevel(@Nullable String logLevel) {
this.logLevel = logLevel;
return this;
}
@CustomType.Setter
public Builder percentComplete(@Nullable Double percentComplete) {
this.percentComplete = percentComplete;
return this;
}
@CustomType.Setter
public Builder provisioningState(@Nullable String provisioningState) {
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder returnAddress(@Nullable ReturnAddressResponse returnAddress) {
this.returnAddress = returnAddress;
return this;
}
@CustomType.Setter
public Builder returnPackage(@Nullable PackageInformationResponse returnPackage) {
this.returnPackage = returnPackage;
return this;
}
@CustomType.Setter
public Builder returnShipping(@Nullable ReturnShippingResponse returnShipping) {
this.returnShipping = returnShipping;
return this;
}
@CustomType.Setter
public Builder shippingInformation(@Nullable ShippingInformationResponse shippingInformation) {
this.shippingInformation = shippingInformation;
return this;
}
@CustomType.Setter
public Builder state(@Nullable String state) {
this.state = state;
return this;
}
@CustomType.Setter
public Builder storageAccountId(@Nullable String storageAccountId) {
this.storageAccountId = storageAccountId;
return this;
}
public JobDetailsResponse build() {
final var _resultValue = new JobDetailsResponse();
_resultValue.backupDriveManifest = backupDriveManifest;
_resultValue.cancelRequested = cancelRequested;
_resultValue.deliveryPackage = deliveryPackage;
_resultValue.diagnosticsPath = diagnosticsPath;
_resultValue.driveList = driveList;
_resultValue.encryptionKey = encryptionKey;
_resultValue.export = export;
_resultValue.incompleteBlobListUri = incompleteBlobListUri;
_resultValue.jobType = jobType;
_resultValue.logLevel = logLevel;
_resultValue.percentComplete = percentComplete;
_resultValue.provisioningState = provisioningState;
_resultValue.returnAddress = returnAddress;
_resultValue.returnPackage = returnPackage;
_resultValue.returnShipping = returnShipping;
_resultValue.shippingInformation = shippingInformation;
_resultValue.state = state;
_resultValue.storageAccountId = storageAccountId;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy