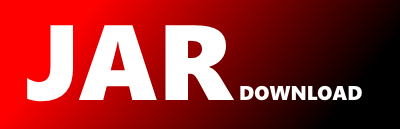
com.pulumi.azurenative.insights.ScheduledQueryRuleArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.insights;
import com.pulumi.azurenative.insights.enums.Kind;
import com.pulumi.azurenative.insights.inputs.ActionsArgs;
import com.pulumi.azurenative.insights.inputs.IdentityArgs;
import com.pulumi.azurenative.insights.inputs.RuleResolveConfigurationArgs;
import com.pulumi.azurenative.insights.inputs.ScheduledQueryRuleCriteriaArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ScheduledQueryRuleArgs extends com.pulumi.resources.ResourceArgs {
public static final ScheduledQueryRuleArgs Empty = new ScheduledQueryRuleArgs();
/**
* Actions to invoke when the alert fires.
*
*/
@Import(name="actions")
private @Nullable Output actions;
/**
* @return Actions to invoke when the alert fires.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy