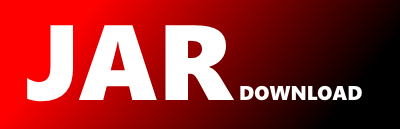
com.pulumi.azurenative.insights.TenantActionGroupArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.insights;
import com.pulumi.azurenative.insights.inputs.AzureAppPushReceiverArgs;
import com.pulumi.azurenative.insights.inputs.EmailReceiverArgs;
import com.pulumi.azurenative.insights.inputs.SmsReceiverArgs;
import com.pulumi.azurenative.insights.inputs.VoiceReceiverArgs;
import com.pulumi.azurenative.insights.inputs.WebhookReceiverArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class TenantActionGroupArgs extends com.pulumi.resources.ResourceArgs {
public static final TenantActionGroupArgs Empty = new TenantActionGroupArgs();
/**
* The list of AzureAppPush receivers that are part of this tenant action group.
*
*/
@Import(name="azureAppPushReceivers")
private @Nullable Output> azureAppPushReceivers;
/**
* @return The list of AzureAppPush receivers that are part of this tenant action group.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy