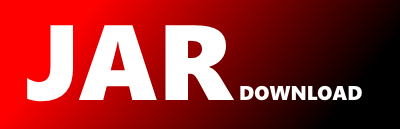
com.pulumi.azurenative.insights.outputs.DataCollectionRuleResponseDataSources Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.insights.outputs;
import com.pulumi.azurenative.insights.outputs.DataSourcesSpecResponseDataImports;
import com.pulumi.azurenative.insights.outputs.ExtensionDataSourceResponse;
import com.pulumi.azurenative.insights.outputs.IisLogsDataSourceResponse;
import com.pulumi.azurenative.insights.outputs.LogFilesDataSourceResponse;
import com.pulumi.azurenative.insights.outputs.PerfCounterDataSourceResponse;
import com.pulumi.azurenative.insights.outputs.PlatformTelemetryDataSourceResponse;
import com.pulumi.azurenative.insights.outputs.PrometheusForwarderDataSourceResponse;
import com.pulumi.azurenative.insights.outputs.SyslogDataSourceResponse;
import com.pulumi.azurenative.insights.outputs.WindowsEventLogDataSourceResponse;
import com.pulumi.azurenative.insights.outputs.WindowsFirewallLogsDataSourceResponse;
import com.pulumi.core.annotations.CustomType;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class DataCollectionRuleResponseDataSources {
/**
* @return Specifications of pull based data sources
*
*/
private @Nullable DataSourcesSpecResponseDataImports dataImports;
/**
* @return The list of Azure VM extension data source configurations.
*
*/
private @Nullable List extensions;
/**
* @return The list of IIS logs source configurations.
*
*/
private @Nullable List iisLogs;
/**
* @return The list of Log files source configurations.
*
*/
private @Nullable List logFiles;
/**
* @return The list of performance counter data source configurations.
*
*/
private @Nullable List performanceCounters;
/**
* @return The list of platform telemetry configurations
*
*/
private @Nullable List platformTelemetry;
/**
* @return The list of Prometheus forwarder data source configurations.
*
*/
private @Nullable List prometheusForwarder;
/**
* @return The list of Syslog data source configurations.
*
*/
private @Nullable List syslog;
/**
* @return The list of Windows Event Log data source configurations.
*
*/
private @Nullable List windowsEventLogs;
/**
* @return The list of Windows Firewall logs source configurations.
*
*/
private @Nullable List windowsFirewallLogs;
private DataCollectionRuleResponseDataSources() {}
/**
* @return Specifications of pull based data sources
*
*/
public Optional dataImports() {
return Optional.ofNullable(this.dataImports);
}
/**
* @return The list of Azure VM extension data source configurations.
*
*/
public List extensions() {
return this.extensions == null ? List.of() : this.extensions;
}
/**
* @return The list of IIS logs source configurations.
*
*/
public List iisLogs() {
return this.iisLogs == null ? List.of() : this.iisLogs;
}
/**
* @return The list of Log files source configurations.
*
*/
public List logFiles() {
return this.logFiles == null ? List.of() : this.logFiles;
}
/**
* @return The list of performance counter data source configurations.
*
*/
public List performanceCounters() {
return this.performanceCounters == null ? List.of() : this.performanceCounters;
}
/**
* @return The list of platform telemetry configurations
*
*/
public List platformTelemetry() {
return this.platformTelemetry == null ? List.of() : this.platformTelemetry;
}
/**
* @return The list of Prometheus forwarder data source configurations.
*
*/
public List prometheusForwarder() {
return this.prometheusForwarder == null ? List.of() : this.prometheusForwarder;
}
/**
* @return The list of Syslog data source configurations.
*
*/
public List syslog() {
return this.syslog == null ? List.of() : this.syslog;
}
/**
* @return The list of Windows Event Log data source configurations.
*
*/
public List windowsEventLogs() {
return this.windowsEventLogs == null ? List.of() : this.windowsEventLogs;
}
/**
* @return The list of Windows Firewall logs source configurations.
*
*/
public List windowsFirewallLogs() {
return this.windowsFirewallLogs == null ? List.of() : this.windowsFirewallLogs;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(DataCollectionRuleResponseDataSources defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable DataSourcesSpecResponseDataImports dataImports;
private @Nullable List extensions;
private @Nullable List iisLogs;
private @Nullable List logFiles;
private @Nullable List performanceCounters;
private @Nullable List platformTelemetry;
private @Nullable List prometheusForwarder;
private @Nullable List syslog;
private @Nullable List windowsEventLogs;
private @Nullable List windowsFirewallLogs;
public Builder() {}
public Builder(DataCollectionRuleResponseDataSources defaults) {
Objects.requireNonNull(defaults);
this.dataImports = defaults.dataImports;
this.extensions = defaults.extensions;
this.iisLogs = defaults.iisLogs;
this.logFiles = defaults.logFiles;
this.performanceCounters = defaults.performanceCounters;
this.platformTelemetry = defaults.platformTelemetry;
this.prometheusForwarder = defaults.prometheusForwarder;
this.syslog = defaults.syslog;
this.windowsEventLogs = defaults.windowsEventLogs;
this.windowsFirewallLogs = defaults.windowsFirewallLogs;
}
@CustomType.Setter
public Builder dataImports(@Nullable DataSourcesSpecResponseDataImports dataImports) {
this.dataImports = dataImports;
return this;
}
@CustomType.Setter
public Builder extensions(@Nullable List extensions) {
this.extensions = extensions;
return this;
}
public Builder extensions(ExtensionDataSourceResponse... extensions) {
return extensions(List.of(extensions));
}
@CustomType.Setter
public Builder iisLogs(@Nullable List iisLogs) {
this.iisLogs = iisLogs;
return this;
}
public Builder iisLogs(IisLogsDataSourceResponse... iisLogs) {
return iisLogs(List.of(iisLogs));
}
@CustomType.Setter
public Builder logFiles(@Nullable List logFiles) {
this.logFiles = logFiles;
return this;
}
public Builder logFiles(LogFilesDataSourceResponse... logFiles) {
return logFiles(List.of(logFiles));
}
@CustomType.Setter
public Builder performanceCounters(@Nullable List performanceCounters) {
this.performanceCounters = performanceCounters;
return this;
}
public Builder performanceCounters(PerfCounterDataSourceResponse... performanceCounters) {
return performanceCounters(List.of(performanceCounters));
}
@CustomType.Setter
public Builder platformTelemetry(@Nullable List platformTelemetry) {
this.platformTelemetry = platformTelemetry;
return this;
}
public Builder platformTelemetry(PlatformTelemetryDataSourceResponse... platformTelemetry) {
return platformTelemetry(List.of(platformTelemetry));
}
@CustomType.Setter
public Builder prometheusForwarder(@Nullable List prometheusForwarder) {
this.prometheusForwarder = prometheusForwarder;
return this;
}
public Builder prometheusForwarder(PrometheusForwarderDataSourceResponse... prometheusForwarder) {
return prometheusForwarder(List.of(prometheusForwarder));
}
@CustomType.Setter
public Builder syslog(@Nullable List syslog) {
this.syslog = syslog;
return this;
}
public Builder syslog(SyslogDataSourceResponse... syslog) {
return syslog(List.of(syslog));
}
@CustomType.Setter
public Builder windowsEventLogs(@Nullable List windowsEventLogs) {
this.windowsEventLogs = windowsEventLogs;
return this;
}
public Builder windowsEventLogs(WindowsEventLogDataSourceResponse... windowsEventLogs) {
return windowsEventLogs(List.of(windowsEventLogs));
}
@CustomType.Setter
public Builder windowsFirewallLogs(@Nullable List windowsFirewallLogs) {
this.windowsFirewallLogs = windowsFirewallLogs;
return this;
}
public Builder windowsFirewallLogs(WindowsFirewallLogsDataSourceResponse... windowsFirewallLogs) {
return windowsFirewallLogs(List.of(windowsFirewallLogs));
}
public DataCollectionRuleResponseDataSources build() {
final var _resultValue = new DataCollectionRuleResponseDataSources();
_resultValue.dataImports = dataImports;
_resultValue.extensions = extensions;
_resultValue.iisLogs = iisLogs;
_resultValue.logFiles = logFiles;
_resultValue.performanceCounters = performanceCounters;
_resultValue.platformTelemetry = platformTelemetry;
_resultValue.prometheusForwarder = prometheusForwarder;
_resultValue.syslog = syslog;
_resultValue.windowsEventLogs = windowsEventLogs;
_resultValue.windowsFirewallLogs = windowsFirewallLogs;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy