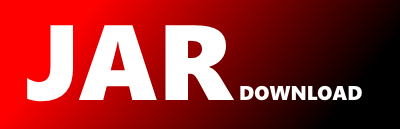
com.pulumi.azurenative.insights.outputs.GetFavoriteResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.insights.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetFavoriteResult {
/**
* @return Favorite category, as defined by the user at creation time.
*
*/
private @Nullable String category;
/**
* @return Configuration of this particular favorite, which are driven by the Azure portal UX. Configuration data is a string containing valid JSON
*
*/
private @Nullable String config;
/**
* @return Internally assigned unique id of the favorite definition.
*
*/
private String favoriteId;
/**
* @return Enum indicating if this favorite definition is owned by a specific user or is shared between all users with access to the Application Insights component.
*
*/
private @Nullable String favoriteType;
/**
* @return Flag denoting wether or not this favorite was generated from a template.
*
*/
private @Nullable Boolean isGeneratedFromTemplate;
/**
* @return The user-defined name of the favorite.
*
*/
private @Nullable String name;
/**
* @return The source of the favorite definition.
*
*/
private @Nullable String sourceType;
/**
* @return A list of 0 or more tags that are associated with this favorite definition
*
*/
private @Nullable List tags;
/**
* @return Date and time in UTC of the last modification that was made to this favorite definition.
*
*/
private String timeModified;
/**
* @return Unique user id of the specific user that owns this favorite.
*
*/
private String userId;
/**
* @return This instance's version of the data model. This can change as new features are added that can be marked favorite. Current examples include MetricsExplorer (ME) and Search.
*
*/
private @Nullable String version;
private GetFavoriteResult() {}
/**
* @return Favorite category, as defined by the user at creation time.
*
*/
public Optional category() {
return Optional.ofNullable(this.category);
}
/**
* @return Configuration of this particular favorite, which are driven by the Azure portal UX. Configuration data is a string containing valid JSON
*
*/
public Optional config() {
return Optional.ofNullable(this.config);
}
/**
* @return Internally assigned unique id of the favorite definition.
*
*/
public String favoriteId() {
return this.favoriteId;
}
/**
* @return Enum indicating if this favorite definition is owned by a specific user or is shared between all users with access to the Application Insights component.
*
*/
public Optional favoriteType() {
return Optional.ofNullable(this.favoriteType);
}
/**
* @return Flag denoting wether or not this favorite was generated from a template.
*
*/
public Optional isGeneratedFromTemplate() {
return Optional.ofNullable(this.isGeneratedFromTemplate);
}
/**
* @return The user-defined name of the favorite.
*
*/
public Optional name() {
return Optional.ofNullable(this.name);
}
/**
* @return The source of the favorite definition.
*
*/
public Optional sourceType() {
return Optional.ofNullable(this.sourceType);
}
/**
* @return A list of 0 or more tags that are associated with this favorite definition
*
*/
public List tags() {
return this.tags == null ? List.of() : this.tags;
}
/**
* @return Date and time in UTC of the last modification that was made to this favorite definition.
*
*/
public String timeModified() {
return this.timeModified;
}
/**
* @return Unique user id of the specific user that owns this favorite.
*
*/
public String userId() {
return this.userId;
}
/**
* @return This instance's version of the data model. This can change as new features are added that can be marked favorite. Current examples include MetricsExplorer (ME) and Search.
*
*/
public Optional version() {
return Optional.ofNullable(this.version);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetFavoriteResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String category;
private @Nullable String config;
private String favoriteId;
private @Nullable String favoriteType;
private @Nullable Boolean isGeneratedFromTemplate;
private @Nullable String name;
private @Nullable String sourceType;
private @Nullable List tags;
private String timeModified;
private String userId;
private @Nullable String version;
public Builder() {}
public Builder(GetFavoriteResult defaults) {
Objects.requireNonNull(defaults);
this.category = defaults.category;
this.config = defaults.config;
this.favoriteId = defaults.favoriteId;
this.favoriteType = defaults.favoriteType;
this.isGeneratedFromTemplate = defaults.isGeneratedFromTemplate;
this.name = defaults.name;
this.sourceType = defaults.sourceType;
this.tags = defaults.tags;
this.timeModified = defaults.timeModified;
this.userId = defaults.userId;
this.version = defaults.version;
}
@CustomType.Setter
public Builder category(@Nullable String category) {
this.category = category;
return this;
}
@CustomType.Setter
public Builder config(@Nullable String config) {
this.config = config;
return this;
}
@CustomType.Setter
public Builder favoriteId(String favoriteId) {
if (favoriteId == null) {
throw new MissingRequiredPropertyException("GetFavoriteResult", "favoriteId");
}
this.favoriteId = favoriteId;
return this;
}
@CustomType.Setter
public Builder favoriteType(@Nullable String favoriteType) {
this.favoriteType = favoriteType;
return this;
}
@CustomType.Setter
public Builder isGeneratedFromTemplate(@Nullable Boolean isGeneratedFromTemplate) {
this.isGeneratedFromTemplate = isGeneratedFromTemplate;
return this;
}
@CustomType.Setter
public Builder name(@Nullable String name) {
this.name = name;
return this;
}
@CustomType.Setter
public Builder sourceType(@Nullable String sourceType) {
this.sourceType = sourceType;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable List tags) {
this.tags = tags;
return this;
}
public Builder tags(String... tags) {
return tags(List.of(tags));
}
@CustomType.Setter
public Builder timeModified(String timeModified) {
if (timeModified == null) {
throw new MissingRequiredPropertyException("GetFavoriteResult", "timeModified");
}
this.timeModified = timeModified;
return this;
}
@CustomType.Setter
public Builder userId(String userId) {
if (userId == null) {
throw new MissingRequiredPropertyException("GetFavoriteResult", "userId");
}
this.userId = userId;
return this;
}
@CustomType.Setter
public Builder version(@Nullable String version) {
this.version = version;
return this;
}
public GetFavoriteResult build() {
final var _resultValue = new GetFavoriteResult();
_resultValue.category = category;
_resultValue.config = config;
_resultValue.favoriteId = favoriteId;
_resultValue.favoriteType = favoriteType;
_resultValue.isGeneratedFromTemplate = isGeneratedFromTemplate;
_resultValue.name = name;
_resultValue.sourceType = sourceType;
_resultValue.tags = tags;
_resultValue.timeModified = timeModified;
_resultValue.userId = userId;
_resultValue.version = version;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy