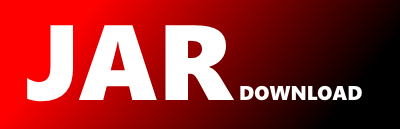
com.pulumi.azurenative.insights.outputs.MetricTriggerResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.insights.outputs;
import com.pulumi.azurenative.insights.outputs.ScaleRuleMetricDimensionResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class MetricTriggerResponse {
/**
* @return List of dimension conditions. For example: [{"DimensionName":"AppName","Operator":"Equals","Values":["App1"]},{"DimensionName":"Deployment","Operator":"Equals","Values":["default"]}].
*
*/
private @Nullable List dimensions;
/**
* @return a value indicating whether metric should divide per instance.
*
*/
private @Nullable Boolean dividePerInstance;
/**
* @return the name of the metric that defines what the rule monitors.
*
*/
private String metricName;
/**
* @return the namespace of the metric that defines what the rule monitors.
*
*/
private @Nullable String metricNamespace;
/**
* @return the location of the resource the rule monitors.
*
*/
private @Nullable String metricResourceLocation;
/**
* @return the resource identifier of the resource the rule monitors.
*
*/
private String metricResourceUri;
/**
* @return the operator that is used to compare the metric data and the threshold.
*
*/
private String operator;
/**
* @return the metric statistic type. How the metrics from multiple instances are combined.
*
*/
private String statistic;
/**
* @return the threshold of the metric that triggers the scale action.
*
*/
private Double threshold;
/**
* @return time aggregation type. How the data that is collected should be combined over time. The default value is Average.
*
*/
private String timeAggregation;
/**
* @return the granularity of metrics the rule monitors. Must be one of the predefined values returned from metric definitions for the metric. Must be between 12 hours and 1 minute.
*
*/
private String timeGrain;
/**
* @return the range of time in which instance data is collected. This value must be greater than the delay in metric collection, which can vary from resource-to-resource. Must be between 12 hours and 5 minutes.
*
*/
private String timeWindow;
private MetricTriggerResponse() {}
/**
* @return List of dimension conditions. For example: [{"DimensionName":"AppName","Operator":"Equals","Values":["App1"]},{"DimensionName":"Deployment","Operator":"Equals","Values":["default"]}].
*
*/
public List dimensions() {
return this.dimensions == null ? List.of() : this.dimensions;
}
/**
* @return a value indicating whether metric should divide per instance.
*
*/
public Optional dividePerInstance() {
return Optional.ofNullable(this.dividePerInstance);
}
/**
* @return the name of the metric that defines what the rule monitors.
*
*/
public String metricName() {
return this.metricName;
}
/**
* @return the namespace of the metric that defines what the rule monitors.
*
*/
public Optional metricNamespace() {
return Optional.ofNullable(this.metricNamespace);
}
/**
* @return the location of the resource the rule monitors.
*
*/
public Optional metricResourceLocation() {
return Optional.ofNullable(this.metricResourceLocation);
}
/**
* @return the resource identifier of the resource the rule monitors.
*
*/
public String metricResourceUri() {
return this.metricResourceUri;
}
/**
* @return the operator that is used to compare the metric data and the threshold.
*
*/
public String operator() {
return this.operator;
}
/**
* @return the metric statistic type. How the metrics from multiple instances are combined.
*
*/
public String statistic() {
return this.statistic;
}
/**
* @return the threshold of the metric that triggers the scale action.
*
*/
public Double threshold() {
return this.threshold;
}
/**
* @return time aggregation type. How the data that is collected should be combined over time. The default value is Average.
*
*/
public String timeAggregation() {
return this.timeAggregation;
}
/**
* @return the granularity of metrics the rule monitors. Must be one of the predefined values returned from metric definitions for the metric. Must be between 12 hours and 1 minute.
*
*/
public String timeGrain() {
return this.timeGrain;
}
/**
* @return the range of time in which instance data is collected. This value must be greater than the delay in metric collection, which can vary from resource-to-resource. Must be between 12 hours and 5 minutes.
*
*/
public String timeWindow() {
return this.timeWindow;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(MetricTriggerResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List dimensions;
private @Nullable Boolean dividePerInstance;
private String metricName;
private @Nullable String metricNamespace;
private @Nullable String metricResourceLocation;
private String metricResourceUri;
private String operator;
private String statistic;
private Double threshold;
private String timeAggregation;
private String timeGrain;
private String timeWindow;
public Builder() {}
public Builder(MetricTriggerResponse defaults) {
Objects.requireNonNull(defaults);
this.dimensions = defaults.dimensions;
this.dividePerInstance = defaults.dividePerInstance;
this.metricName = defaults.metricName;
this.metricNamespace = defaults.metricNamespace;
this.metricResourceLocation = defaults.metricResourceLocation;
this.metricResourceUri = defaults.metricResourceUri;
this.operator = defaults.operator;
this.statistic = defaults.statistic;
this.threshold = defaults.threshold;
this.timeAggregation = defaults.timeAggregation;
this.timeGrain = defaults.timeGrain;
this.timeWindow = defaults.timeWindow;
}
@CustomType.Setter
public Builder dimensions(@Nullable List dimensions) {
this.dimensions = dimensions;
return this;
}
public Builder dimensions(ScaleRuleMetricDimensionResponse... dimensions) {
return dimensions(List.of(dimensions));
}
@CustomType.Setter
public Builder dividePerInstance(@Nullable Boolean dividePerInstance) {
this.dividePerInstance = dividePerInstance;
return this;
}
@CustomType.Setter
public Builder metricName(String metricName) {
if (metricName == null) {
throw new MissingRequiredPropertyException("MetricTriggerResponse", "metricName");
}
this.metricName = metricName;
return this;
}
@CustomType.Setter
public Builder metricNamespace(@Nullable String metricNamespace) {
this.metricNamespace = metricNamespace;
return this;
}
@CustomType.Setter
public Builder metricResourceLocation(@Nullable String metricResourceLocation) {
this.metricResourceLocation = metricResourceLocation;
return this;
}
@CustomType.Setter
public Builder metricResourceUri(String metricResourceUri) {
if (metricResourceUri == null) {
throw new MissingRequiredPropertyException("MetricTriggerResponse", "metricResourceUri");
}
this.metricResourceUri = metricResourceUri;
return this;
}
@CustomType.Setter
public Builder operator(String operator) {
if (operator == null) {
throw new MissingRequiredPropertyException("MetricTriggerResponse", "operator");
}
this.operator = operator;
return this;
}
@CustomType.Setter
public Builder statistic(String statistic) {
if (statistic == null) {
throw new MissingRequiredPropertyException("MetricTriggerResponse", "statistic");
}
this.statistic = statistic;
return this;
}
@CustomType.Setter
public Builder threshold(Double threshold) {
if (threshold == null) {
throw new MissingRequiredPropertyException("MetricTriggerResponse", "threshold");
}
this.threshold = threshold;
return this;
}
@CustomType.Setter
public Builder timeAggregation(String timeAggregation) {
if (timeAggregation == null) {
throw new MissingRequiredPropertyException("MetricTriggerResponse", "timeAggregation");
}
this.timeAggregation = timeAggregation;
return this;
}
@CustomType.Setter
public Builder timeGrain(String timeGrain) {
if (timeGrain == null) {
throw new MissingRequiredPropertyException("MetricTriggerResponse", "timeGrain");
}
this.timeGrain = timeGrain;
return this;
}
@CustomType.Setter
public Builder timeWindow(String timeWindow) {
if (timeWindow == null) {
throw new MissingRequiredPropertyException("MetricTriggerResponse", "timeWindow");
}
this.timeWindow = timeWindow;
return this;
}
public MetricTriggerResponse build() {
final var _resultValue = new MetricTriggerResponse();
_resultValue.dimensions = dimensions;
_resultValue.dividePerInstance = dividePerInstance;
_resultValue.metricName = metricName;
_resultValue.metricNamespace = metricNamespace;
_resultValue.metricResourceLocation = metricResourceLocation;
_resultValue.metricResourceUri = metricResourceUri;
_resultValue.operator = operator;
_resultValue.statistic = statistic;
_resultValue.threshold = threshold;
_resultValue.timeAggregation = timeAggregation;
_resultValue.timeGrain = timeGrain;
_resultValue.timeWindow = timeWindow;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy