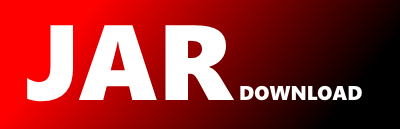
com.pulumi.azurenative.iotoperations.outputs.DataFlowEndpointMqttResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.iotoperations.outputs;
import com.pulumi.azurenative.iotoperations.outputs.TlsPropertiesResponse;
import com.pulumi.core.annotations.CustomType;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class DataFlowEndpointMqttResponse {
/**
* @return Client ID prefix. Client ID generated by the dataflow is <prefix>-TBD. Optional; no prefix if omitted.
*
*/
private @Nullable String clientIdPrefix;
/**
* @return Host of the Broker in the form of <hostname>:<port>. Optional; connects to Broker if omitted.
*
*/
private @Nullable String host;
/**
* @return Broker KeepAlive for connection in seconds.
*
*/
private @Nullable Integer keepAliveSeconds;
/**
* @return The max number of messages to keep in flight. For subscribe, this is the receive maximum. For publish, this is the maximum number of messages to send before waiting for an ack.
*
*/
private @Nullable Integer maxInflightMessages;
/**
* @return Enable or disable websockets.
*
*/
private @Nullable String protocol;
/**
* @return Qos for Broker connection.
*
*/
private @Nullable Integer qos;
/**
* @return Whether or not to keep the retain setting.
*
*/
private @Nullable String retain;
/**
* @return Session expiry in seconds.
*
*/
private @Nullable Integer sessionExpirySeconds;
/**
* @return TLS configuration.
*
*/
private @Nullable TlsPropertiesResponse tls;
private DataFlowEndpointMqttResponse() {}
/**
* @return Client ID prefix. Client ID generated by the dataflow is <prefix>-TBD. Optional; no prefix if omitted.
*
*/
public Optional clientIdPrefix() {
return Optional.ofNullable(this.clientIdPrefix);
}
/**
* @return Host of the Broker in the form of <hostname>:<port>. Optional; connects to Broker if omitted.
*
*/
public Optional host() {
return Optional.ofNullable(this.host);
}
/**
* @return Broker KeepAlive for connection in seconds.
*
*/
public Optional keepAliveSeconds() {
return Optional.ofNullable(this.keepAliveSeconds);
}
/**
* @return The max number of messages to keep in flight. For subscribe, this is the receive maximum. For publish, this is the maximum number of messages to send before waiting for an ack.
*
*/
public Optional maxInflightMessages() {
return Optional.ofNullable(this.maxInflightMessages);
}
/**
* @return Enable or disable websockets.
*
*/
public Optional protocol() {
return Optional.ofNullable(this.protocol);
}
/**
* @return Qos for Broker connection.
*
*/
public Optional qos() {
return Optional.ofNullable(this.qos);
}
/**
* @return Whether or not to keep the retain setting.
*
*/
public Optional retain() {
return Optional.ofNullable(this.retain);
}
/**
* @return Session expiry in seconds.
*
*/
public Optional sessionExpirySeconds() {
return Optional.ofNullable(this.sessionExpirySeconds);
}
/**
* @return TLS configuration.
*
*/
public Optional tls() {
return Optional.ofNullable(this.tls);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(DataFlowEndpointMqttResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String clientIdPrefix;
private @Nullable String host;
private @Nullable Integer keepAliveSeconds;
private @Nullable Integer maxInflightMessages;
private @Nullable String protocol;
private @Nullable Integer qos;
private @Nullable String retain;
private @Nullable Integer sessionExpirySeconds;
private @Nullable TlsPropertiesResponse tls;
public Builder() {}
public Builder(DataFlowEndpointMqttResponse defaults) {
Objects.requireNonNull(defaults);
this.clientIdPrefix = defaults.clientIdPrefix;
this.host = defaults.host;
this.keepAliveSeconds = defaults.keepAliveSeconds;
this.maxInflightMessages = defaults.maxInflightMessages;
this.protocol = defaults.protocol;
this.qos = defaults.qos;
this.retain = defaults.retain;
this.sessionExpirySeconds = defaults.sessionExpirySeconds;
this.tls = defaults.tls;
}
@CustomType.Setter
public Builder clientIdPrefix(@Nullable String clientIdPrefix) {
this.clientIdPrefix = clientIdPrefix;
return this;
}
@CustomType.Setter
public Builder host(@Nullable String host) {
this.host = host;
return this;
}
@CustomType.Setter
public Builder keepAliveSeconds(@Nullable Integer keepAliveSeconds) {
this.keepAliveSeconds = keepAliveSeconds;
return this;
}
@CustomType.Setter
public Builder maxInflightMessages(@Nullable Integer maxInflightMessages) {
this.maxInflightMessages = maxInflightMessages;
return this;
}
@CustomType.Setter
public Builder protocol(@Nullable String protocol) {
this.protocol = protocol;
return this;
}
@CustomType.Setter
public Builder qos(@Nullable Integer qos) {
this.qos = qos;
return this;
}
@CustomType.Setter
public Builder retain(@Nullable String retain) {
this.retain = retain;
return this;
}
@CustomType.Setter
public Builder sessionExpirySeconds(@Nullable Integer sessionExpirySeconds) {
this.sessionExpirySeconds = sessionExpirySeconds;
return this;
}
@CustomType.Setter
public Builder tls(@Nullable TlsPropertiesResponse tls) {
this.tls = tls;
return this;
}
public DataFlowEndpointMqttResponse build() {
final var _resultValue = new DataFlowEndpointMqttResponse();
_resultValue.clientIdPrefix = clientIdPrefix;
_resultValue.host = host;
_resultValue.keepAliveSeconds = keepAliveSeconds;
_resultValue.maxInflightMessages = maxInflightMessages;
_resultValue.protocol = protocol;
_resultValue.qos = qos;
_resultValue.retain = retain;
_resultValue.sessionExpirySeconds = sessionExpirySeconds;
_resultValue.tls = tls;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy