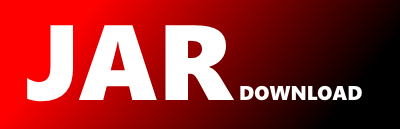
com.pulumi.azurenative.iotoperationsmq.KafkaConnectorArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.iotoperationsmq;
import com.pulumi.azurenative.iotoperationsmq.inputs.ContainerImageArgs;
import com.pulumi.azurenative.iotoperationsmq.inputs.ExtendedLocationPropertyArgs;
import com.pulumi.azurenative.iotoperationsmq.inputs.KafkaRemoteBrokerConnectionSpecArgs;
import com.pulumi.azurenative.iotoperationsmq.inputs.LocalBrokerConnectionSpecArgs;
import com.pulumi.azurenative.iotoperationsmq.inputs.NodeTolerationsArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class KafkaConnectorArgs extends com.pulumi.resources.ResourceArgs {
public static final KafkaConnectorArgs Empty = new KafkaConnectorArgs();
/**
* The client id prefix of the dynamically generated client ids.
*
*/
@Import(name="clientIdPrefix")
private @Nullable Output clientIdPrefix;
/**
* @return The client id prefix of the dynamically generated client ids.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy