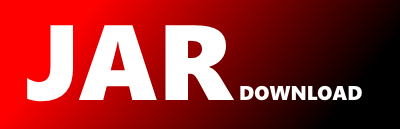
com.pulumi.azurenative.iotoperationsmq.inputs.DiskBackedMessageBufferSettingsArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.iotoperationsmq.inputs;
import com.pulumi.azurenative.iotoperationsmq.inputs.VolumeClaimSpecArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* DiskBackedMessageBufferSettings properties
*
*/
public final class DiskBackedMessageBufferSettingsArgs extends com.pulumi.resources.ResourceArgs {
public static final DiskBackedMessageBufferSettingsArgs Empty = new DiskBackedMessageBufferSettingsArgs();
/**
* Use the specified persistent volume claim template to mount a "generic ephemeral volume" for the message buffer. See <https://kubernetes.io/docs/concepts/storage/ephemeral-volumes/#generic-ephemeral-volumes> for details.
*
*/
@Import(name="ephemeralVolumeClaimSpec")
private @Nullable Output ephemeralVolumeClaimSpec;
/**
* @return Use the specified persistent volume claim template to mount a "generic ephemeral volume" for the message buffer. See <https://kubernetes.io/docs/concepts/storage/ephemeral-volumes/#generic-ephemeral-volumes> for details.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy