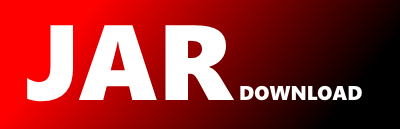
com.pulumi.azurenative.iotoperationsmq.outputs.TemporaryResourceLimitsConfigResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.iotoperationsmq.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.Integer;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class TemporaryResourceLimitsConfigResponse {
/**
* @return Maximum number of messages a client can have inflight.
*
*/
private Integer maxInflightMessages;
/**
* @return Maximum number of patch inflight per node.
*
*/
private Integer maxInflightPatches;
/**
* @return Maximum number of patch a client can have in flight.
*
*/
private Integer maxInflightPatchesPerClient;
/**
* @return Maximum message expiry interval, in seconds.
*
*/
private @Nullable Double maxMessageExpirySecs;
/**
* @return Maximum receive for external clients.
*
*/
private Double maxQueuedMessages;
/**
* @return Maximum receive QoS0 for external clients.
*
*/
private Double maxQueuedQos0Messages;
/**
* @return Maximum session expiry interval, in seconds.
*
*/
private Double maxSessionExpirySecs;
private TemporaryResourceLimitsConfigResponse() {}
/**
* @return Maximum number of messages a client can have inflight.
*
*/
public Integer maxInflightMessages() {
return this.maxInflightMessages;
}
/**
* @return Maximum number of patch inflight per node.
*
*/
public Integer maxInflightPatches() {
return this.maxInflightPatches;
}
/**
* @return Maximum number of patch a client can have in flight.
*
*/
public Integer maxInflightPatchesPerClient() {
return this.maxInflightPatchesPerClient;
}
/**
* @return Maximum message expiry interval, in seconds.
*
*/
public Optional maxMessageExpirySecs() {
return Optional.ofNullable(this.maxMessageExpirySecs);
}
/**
* @return Maximum receive for external clients.
*
*/
public Double maxQueuedMessages() {
return this.maxQueuedMessages;
}
/**
* @return Maximum receive QoS0 for external clients.
*
*/
public Double maxQueuedQos0Messages() {
return this.maxQueuedQos0Messages;
}
/**
* @return Maximum session expiry interval, in seconds.
*
*/
public Double maxSessionExpirySecs() {
return this.maxSessionExpirySecs;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(TemporaryResourceLimitsConfigResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Integer maxInflightMessages;
private Integer maxInflightPatches;
private Integer maxInflightPatchesPerClient;
private @Nullable Double maxMessageExpirySecs;
private Double maxQueuedMessages;
private Double maxQueuedQos0Messages;
private Double maxSessionExpirySecs;
public Builder() {}
public Builder(TemporaryResourceLimitsConfigResponse defaults) {
Objects.requireNonNull(defaults);
this.maxInflightMessages = defaults.maxInflightMessages;
this.maxInflightPatches = defaults.maxInflightPatches;
this.maxInflightPatchesPerClient = defaults.maxInflightPatchesPerClient;
this.maxMessageExpirySecs = defaults.maxMessageExpirySecs;
this.maxQueuedMessages = defaults.maxQueuedMessages;
this.maxQueuedQos0Messages = defaults.maxQueuedQos0Messages;
this.maxSessionExpirySecs = defaults.maxSessionExpirySecs;
}
@CustomType.Setter
public Builder maxInflightMessages(Integer maxInflightMessages) {
if (maxInflightMessages == null) {
throw new MissingRequiredPropertyException("TemporaryResourceLimitsConfigResponse", "maxInflightMessages");
}
this.maxInflightMessages = maxInflightMessages;
return this;
}
@CustomType.Setter
public Builder maxInflightPatches(Integer maxInflightPatches) {
if (maxInflightPatches == null) {
throw new MissingRequiredPropertyException("TemporaryResourceLimitsConfigResponse", "maxInflightPatches");
}
this.maxInflightPatches = maxInflightPatches;
return this;
}
@CustomType.Setter
public Builder maxInflightPatchesPerClient(Integer maxInflightPatchesPerClient) {
if (maxInflightPatchesPerClient == null) {
throw new MissingRequiredPropertyException("TemporaryResourceLimitsConfigResponse", "maxInflightPatchesPerClient");
}
this.maxInflightPatchesPerClient = maxInflightPatchesPerClient;
return this;
}
@CustomType.Setter
public Builder maxMessageExpirySecs(@Nullable Double maxMessageExpirySecs) {
this.maxMessageExpirySecs = maxMessageExpirySecs;
return this;
}
@CustomType.Setter
public Builder maxQueuedMessages(Double maxQueuedMessages) {
if (maxQueuedMessages == null) {
throw new MissingRequiredPropertyException("TemporaryResourceLimitsConfigResponse", "maxQueuedMessages");
}
this.maxQueuedMessages = maxQueuedMessages;
return this;
}
@CustomType.Setter
public Builder maxQueuedQos0Messages(Double maxQueuedQos0Messages) {
if (maxQueuedQos0Messages == null) {
throw new MissingRequiredPropertyException("TemporaryResourceLimitsConfigResponse", "maxQueuedQos0Messages");
}
this.maxQueuedQos0Messages = maxQueuedQos0Messages;
return this;
}
@CustomType.Setter
public Builder maxSessionExpirySecs(Double maxSessionExpirySecs) {
if (maxSessionExpirySecs == null) {
throw new MissingRequiredPropertyException("TemporaryResourceLimitsConfigResponse", "maxSessionExpirySecs");
}
this.maxSessionExpirySecs = maxSessionExpirySecs;
return this;
}
public TemporaryResourceLimitsConfigResponse build() {
final var _resultValue = new TemporaryResourceLimitsConfigResponse();
_resultValue.maxInflightMessages = maxInflightMessages;
_resultValue.maxInflightPatches = maxInflightPatches;
_resultValue.maxInflightPatchesPerClient = maxInflightPatchesPerClient;
_resultValue.maxMessageExpirySecs = maxMessageExpirySecs;
_resultValue.maxQueuedMessages = maxQueuedMessages;
_resultValue.maxQueuedQos0Messages = maxQueuedQos0Messages;
_resultValue.maxSessionExpirySecs = maxSessionExpirySecs;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy