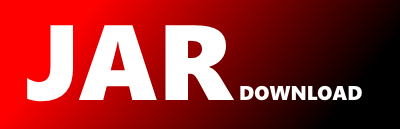
com.pulumi.azurenative.keyvault.KeyvaultFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.keyvault;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.keyvault.inputs.GetKeyArgs;
import com.pulumi.azurenative.keyvault.inputs.GetKeyPlainArgs;
import com.pulumi.azurenative.keyvault.inputs.GetMHSMPrivateEndpointConnectionArgs;
import com.pulumi.azurenative.keyvault.inputs.GetMHSMPrivateEndpointConnectionPlainArgs;
import com.pulumi.azurenative.keyvault.inputs.GetManagedHsmArgs;
import com.pulumi.azurenative.keyvault.inputs.GetManagedHsmPlainArgs;
import com.pulumi.azurenative.keyvault.inputs.GetPrivateEndpointConnectionArgs;
import com.pulumi.azurenative.keyvault.inputs.GetPrivateEndpointConnectionPlainArgs;
import com.pulumi.azurenative.keyvault.inputs.GetSecretArgs;
import com.pulumi.azurenative.keyvault.inputs.GetSecretPlainArgs;
import com.pulumi.azurenative.keyvault.inputs.GetVaultArgs;
import com.pulumi.azurenative.keyvault.inputs.GetVaultPlainArgs;
import com.pulumi.azurenative.keyvault.outputs.GetKeyResult;
import com.pulumi.azurenative.keyvault.outputs.GetMHSMPrivateEndpointConnectionResult;
import com.pulumi.azurenative.keyvault.outputs.GetManagedHsmResult;
import com.pulumi.azurenative.keyvault.outputs.GetPrivateEndpointConnectionResult;
import com.pulumi.azurenative.keyvault.outputs.GetSecretResult;
import com.pulumi.azurenative.keyvault.outputs.GetVaultResult;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import java.util.concurrent.CompletableFuture;
public final class KeyvaultFunctions {
/**
* Gets the current version of the specified key from the specified key vault.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2023-07-01, 2024-04-01-preview.
*
*/
public static Output getKey(GetKeyArgs args) {
return getKey(args, InvokeOptions.Empty);
}
/**
* Gets the current version of the specified key from the specified key vault.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2023-07-01, 2024-04-01-preview.
*
*/
public static CompletableFuture getKeyPlain(GetKeyPlainArgs args) {
return getKeyPlain(args, InvokeOptions.Empty);
}
/**
* Gets the current version of the specified key from the specified key vault.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2023-07-01, 2024-04-01-preview.
*
*/
public static Output getKey(GetKeyArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:keyvault:getKey", TypeShape.of(GetKeyResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the current version of the specified key from the specified key vault.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2023-07-01, 2024-04-01-preview.
*
*/
public static CompletableFuture getKeyPlain(GetKeyPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:keyvault:getKey", TypeShape.of(GetKeyResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the specified private endpoint connection associated with the managed HSM Pool.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2023-07-01, 2024-04-01-preview.
*
*/
public static Output getMHSMPrivateEndpointConnection(GetMHSMPrivateEndpointConnectionArgs args) {
return getMHSMPrivateEndpointConnection(args, InvokeOptions.Empty);
}
/**
* Gets the specified private endpoint connection associated with the managed HSM Pool.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2023-07-01, 2024-04-01-preview.
*
*/
public static CompletableFuture getMHSMPrivateEndpointConnectionPlain(GetMHSMPrivateEndpointConnectionPlainArgs args) {
return getMHSMPrivateEndpointConnectionPlain(args, InvokeOptions.Empty);
}
/**
* Gets the specified private endpoint connection associated with the managed HSM Pool.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2023-07-01, 2024-04-01-preview.
*
*/
public static Output getMHSMPrivateEndpointConnection(GetMHSMPrivateEndpointConnectionArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:keyvault:getMHSMPrivateEndpointConnection", TypeShape.of(GetMHSMPrivateEndpointConnectionResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the specified private endpoint connection associated with the managed HSM Pool.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2023-07-01, 2024-04-01-preview.
*
*/
public static CompletableFuture getMHSMPrivateEndpointConnectionPlain(GetMHSMPrivateEndpointConnectionPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:keyvault:getMHSMPrivateEndpointConnection", TypeShape.of(GetMHSMPrivateEndpointConnectionResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the specified managed HSM Pool.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2023-07-01, 2024-04-01-preview.
*
*/
public static Output getManagedHsm(GetManagedHsmArgs args) {
return getManagedHsm(args, InvokeOptions.Empty);
}
/**
* Gets the specified managed HSM Pool.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2023-07-01, 2024-04-01-preview.
*
*/
public static CompletableFuture getManagedHsmPlain(GetManagedHsmPlainArgs args) {
return getManagedHsmPlain(args, InvokeOptions.Empty);
}
/**
* Gets the specified managed HSM Pool.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2023-07-01, 2024-04-01-preview.
*
*/
public static Output getManagedHsm(GetManagedHsmArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:keyvault:getManagedHsm", TypeShape.of(GetManagedHsmResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the specified managed HSM Pool.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2023-07-01, 2024-04-01-preview.
*
*/
public static CompletableFuture getManagedHsmPlain(GetManagedHsmPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:keyvault:getManagedHsm", TypeShape.of(GetManagedHsmResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the specified private endpoint connection associated with the key vault.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2023-07-01, 2024-04-01-preview.
*
*/
public static Output getPrivateEndpointConnection(GetPrivateEndpointConnectionArgs args) {
return getPrivateEndpointConnection(args, InvokeOptions.Empty);
}
/**
* Gets the specified private endpoint connection associated with the key vault.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2023-07-01, 2024-04-01-preview.
*
*/
public static CompletableFuture getPrivateEndpointConnectionPlain(GetPrivateEndpointConnectionPlainArgs args) {
return getPrivateEndpointConnectionPlain(args, InvokeOptions.Empty);
}
/**
* Gets the specified private endpoint connection associated with the key vault.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2023-07-01, 2024-04-01-preview.
*
*/
public static Output getPrivateEndpointConnection(GetPrivateEndpointConnectionArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:keyvault:getPrivateEndpointConnection", TypeShape.of(GetPrivateEndpointConnectionResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the specified private endpoint connection associated with the key vault.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2023-07-01, 2024-04-01-preview.
*
*/
public static CompletableFuture getPrivateEndpointConnectionPlain(GetPrivateEndpointConnectionPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:keyvault:getPrivateEndpointConnection", TypeShape.of(GetPrivateEndpointConnectionResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the specified secret. NOTE: This API is intended for internal use in ARM deployments. Users should use the data-plane REST service for interaction with vault secrets.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2023-07-01, 2024-04-01-preview.
*
*/
public static Output getSecret(GetSecretArgs args) {
return getSecret(args, InvokeOptions.Empty);
}
/**
* Gets the specified secret. NOTE: This API is intended for internal use in ARM deployments. Users should use the data-plane REST service for interaction with vault secrets.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2023-07-01, 2024-04-01-preview.
*
*/
public static CompletableFuture getSecretPlain(GetSecretPlainArgs args) {
return getSecretPlain(args, InvokeOptions.Empty);
}
/**
* Gets the specified secret. NOTE: This API is intended for internal use in ARM deployments. Users should use the data-plane REST service for interaction with vault secrets.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2023-07-01, 2024-04-01-preview.
*
*/
public static Output getSecret(GetSecretArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:keyvault:getSecret", TypeShape.of(GetSecretResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the specified secret. NOTE: This API is intended for internal use in ARM deployments. Users should use the data-plane REST service for interaction with vault secrets.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2023-07-01, 2024-04-01-preview.
*
*/
public static CompletableFuture getSecretPlain(GetSecretPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:keyvault:getSecret", TypeShape.of(GetSecretResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the specified Azure key vault.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2018-02-14-preview, 2023-07-01, 2024-04-01-preview.
*
*/
public static Output getVault(GetVaultArgs args) {
return getVault(args, InvokeOptions.Empty);
}
/**
* Gets the specified Azure key vault.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2018-02-14-preview, 2023-07-01, 2024-04-01-preview.
*
*/
public static CompletableFuture getVaultPlain(GetVaultPlainArgs args) {
return getVaultPlain(args, InvokeOptions.Empty);
}
/**
* Gets the specified Azure key vault.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2018-02-14-preview, 2023-07-01, 2024-04-01-preview.
*
*/
public static Output getVault(GetVaultArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:keyvault:getVault", TypeShape.of(GetVaultResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the specified Azure key vault.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2018-02-14-preview, 2023-07-01, 2024-04-01-preview.
*
*/
public static CompletableFuture getVaultPlain(GetVaultPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:keyvault:getVault", TypeShape.of(GetVaultResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy