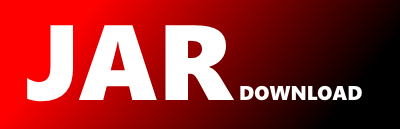
com.pulumi.azurenative.keyvault.outputs.GetKeyResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.keyvault.outputs;
import com.pulumi.azurenative.keyvault.outputs.KeyAttributesResponse;
import com.pulumi.azurenative.keyvault.outputs.KeyReleasePolicyResponse;
import com.pulumi.azurenative.keyvault.outputs.RotationPolicyResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetKeyResult {
/**
* @return The attributes of the key.
*
*/
private @Nullable KeyAttributesResponse attributes;
/**
* @return The elliptic curve name. For valid values, see JsonWebKeyCurveName.
*
*/
private @Nullable String curveName;
/**
* @return Fully qualified identifier of the key vault resource.
*
*/
private String id;
private @Nullable List keyOps;
/**
* @return The key size in bits. For example: 2048, 3072, or 4096 for RSA.
*
*/
private @Nullable Integer keySize;
/**
* @return The URI to retrieve the current version of the key.
*
*/
private String keyUri;
/**
* @return The URI to retrieve the specific version of the key.
*
*/
private String keyUriWithVersion;
/**
* @return The type of the key. For valid values, see JsonWebKeyType.
*
*/
private @Nullable String kty;
/**
* @return Azure location of the key vault resource.
*
*/
private String location;
/**
* @return Name of the key vault resource.
*
*/
private String name;
/**
* @return Key release policy in response. It will be used for both output and input. Omitted if empty
*
*/
private @Nullable KeyReleasePolicyResponse releasePolicy;
/**
* @return Key rotation policy in response. It will be used for both output and input. Omitted if empty
*
*/
private @Nullable RotationPolicyResponse rotationPolicy;
/**
* @return Tags assigned to the key vault resource.
*
*/
private Map tags;
/**
* @return Resource type of the key vault resource.
*
*/
private String type;
private GetKeyResult() {}
/**
* @return The attributes of the key.
*
*/
public Optional attributes() {
return Optional.ofNullable(this.attributes);
}
/**
* @return The elliptic curve name. For valid values, see JsonWebKeyCurveName.
*
*/
public Optional curveName() {
return Optional.ofNullable(this.curveName);
}
/**
* @return Fully qualified identifier of the key vault resource.
*
*/
public String id() {
return this.id;
}
public List keyOps() {
return this.keyOps == null ? List.of() : this.keyOps;
}
/**
* @return The key size in bits. For example: 2048, 3072, or 4096 for RSA.
*
*/
public Optional keySize() {
return Optional.ofNullable(this.keySize);
}
/**
* @return The URI to retrieve the current version of the key.
*
*/
public String keyUri() {
return this.keyUri;
}
/**
* @return The URI to retrieve the specific version of the key.
*
*/
public String keyUriWithVersion() {
return this.keyUriWithVersion;
}
/**
* @return The type of the key. For valid values, see JsonWebKeyType.
*
*/
public Optional kty() {
return Optional.ofNullable(this.kty);
}
/**
* @return Azure location of the key vault resource.
*
*/
public String location() {
return this.location;
}
/**
* @return Name of the key vault resource.
*
*/
public String name() {
return this.name;
}
/**
* @return Key release policy in response. It will be used for both output and input. Omitted if empty
*
*/
public Optional releasePolicy() {
return Optional.ofNullable(this.releasePolicy);
}
/**
* @return Key rotation policy in response. It will be used for both output and input. Omitted if empty
*
*/
public Optional rotationPolicy() {
return Optional.ofNullable(this.rotationPolicy);
}
/**
* @return Tags assigned to the key vault resource.
*
*/
public Map tags() {
return this.tags;
}
/**
* @return Resource type of the key vault resource.
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetKeyResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable KeyAttributesResponse attributes;
private @Nullable String curveName;
private String id;
private @Nullable List keyOps;
private @Nullable Integer keySize;
private String keyUri;
private String keyUriWithVersion;
private @Nullable String kty;
private String location;
private String name;
private @Nullable KeyReleasePolicyResponse releasePolicy;
private @Nullable RotationPolicyResponse rotationPolicy;
private Map tags;
private String type;
public Builder() {}
public Builder(GetKeyResult defaults) {
Objects.requireNonNull(defaults);
this.attributes = defaults.attributes;
this.curveName = defaults.curveName;
this.id = defaults.id;
this.keyOps = defaults.keyOps;
this.keySize = defaults.keySize;
this.keyUri = defaults.keyUri;
this.keyUriWithVersion = defaults.keyUriWithVersion;
this.kty = defaults.kty;
this.location = defaults.location;
this.name = defaults.name;
this.releasePolicy = defaults.releasePolicy;
this.rotationPolicy = defaults.rotationPolicy;
this.tags = defaults.tags;
this.type = defaults.type;
}
@CustomType.Setter
public Builder attributes(@Nullable KeyAttributesResponse attributes) {
this.attributes = attributes;
return this;
}
@CustomType.Setter
public Builder curveName(@Nullable String curveName) {
this.curveName = curveName;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetKeyResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder keyOps(@Nullable List keyOps) {
this.keyOps = keyOps;
return this;
}
public Builder keyOps(String... keyOps) {
return keyOps(List.of(keyOps));
}
@CustomType.Setter
public Builder keySize(@Nullable Integer keySize) {
this.keySize = keySize;
return this;
}
@CustomType.Setter
public Builder keyUri(String keyUri) {
if (keyUri == null) {
throw new MissingRequiredPropertyException("GetKeyResult", "keyUri");
}
this.keyUri = keyUri;
return this;
}
@CustomType.Setter
public Builder keyUriWithVersion(String keyUriWithVersion) {
if (keyUriWithVersion == null) {
throw new MissingRequiredPropertyException("GetKeyResult", "keyUriWithVersion");
}
this.keyUriWithVersion = keyUriWithVersion;
return this;
}
@CustomType.Setter
public Builder kty(@Nullable String kty) {
this.kty = kty;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetKeyResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetKeyResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder releasePolicy(@Nullable KeyReleasePolicyResponse releasePolicy) {
this.releasePolicy = releasePolicy;
return this;
}
@CustomType.Setter
public Builder rotationPolicy(@Nullable RotationPolicyResponse rotationPolicy) {
this.rotationPolicy = rotationPolicy;
return this;
}
@CustomType.Setter
public Builder tags(Map tags) {
if (tags == null) {
throw new MissingRequiredPropertyException("GetKeyResult", "tags");
}
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetKeyResult", "type");
}
this.type = type;
return this;
}
public GetKeyResult build() {
final var _resultValue = new GetKeyResult();
_resultValue.attributes = attributes;
_resultValue.curveName = curveName;
_resultValue.id = id;
_resultValue.keyOps = keyOps;
_resultValue.keySize = keySize;
_resultValue.keyUri = keyUri;
_resultValue.keyUriWithVersion = keyUriWithVersion;
_resultValue.kty = kty;
_resultValue.location = location;
_resultValue.name = name;
_resultValue.releasePolicy = releasePolicy;
_resultValue.rotationPolicy = rotationPolicy;
_resultValue.tags = tags;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy