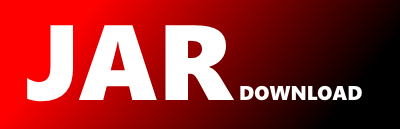
com.pulumi.azurenative.kubernetesconfiguration.inputs.ServicePrincipalDefinitionArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.kubernetesconfiguration.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Parameters to authenticate using Service Principal.
*
*/
public final class ServicePrincipalDefinitionArgs extends com.pulumi.resources.ResourceArgs {
public static final ServicePrincipalDefinitionArgs Empty = new ServicePrincipalDefinitionArgs();
/**
* Base64-encoded certificate used to authenticate a Service Principal
*
*/
@Import(name="clientCertificate")
private @Nullable Output clientCertificate;
/**
* @return Base64-encoded certificate used to authenticate a Service Principal
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy