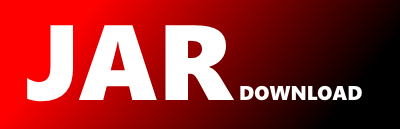
com.pulumi.azurenative.kusto.EventHubDataConnection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.kusto;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.kusto.EventHubDataConnectionArgs;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Class representing an event hub data connection.
* Azure REST API version: 2022-12-29. Prior API version in Azure Native 1.x: 2021-01-01.
*
* ## Example Usage
* ### KustoDataConnectionsCosmosDbCreateOrUpdate
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.kusto.EventHubDataConnection;
* import com.pulumi.azurenative.kusto.EventHubDataConnectionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var eventHubDataConnection = new EventHubDataConnection("eventHubDataConnection", EventHubDataConnectionArgs.builder()
* .clusterName("kustoCluster")
* .dataConnectionName("dataConnectionTest")
* .databaseName("KustoDatabase1")
* .resourceGroupName("kustorptest")
* .build());
*
* }
* }
*
* }
*
* ### KustoDataConnectionsCreateOrUpdate
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.kusto.EventHubDataConnection;
* import com.pulumi.azurenative.kusto.EventHubDataConnectionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var eventHubDataConnection = new EventHubDataConnection("eventHubDataConnection", EventHubDataConnectionArgs.builder()
* .clusterName("kustoCluster")
* .consumerGroup("testConsumerGroup1")
* .dataConnectionName("dataConnectionTest")
* .databaseName("KustoDatabase8")
* .eventHubResourceId("/subscriptions/12345678-1234-1234-1234-123456789098/resourceGroups/kustorptest/providers/Microsoft.EventHub/namespaces/eventhubTestns1/eventhubs/eventhubTest1")
* .kind("EventHub")
* .location("westus")
* .managedIdentityResourceId("/subscriptions/12345678-1234-1234-1234-123456789098/resourceGroups/kustorptest/providers/Microsoft.ManagedIdentity/userAssignedIdentities/managedidentityTest1")
* .resourceGroupName("kustorptest")
* .build());
*
* }
* }
*
* }
*
* ### KustoDataConnectionsEventGridCreateOrUpdate
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.kusto.EventHubDataConnection;
* import com.pulumi.azurenative.kusto.EventHubDataConnectionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var eventHubDataConnection = new EventHubDataConnection("eventHubDataConnection", EventHubDataConnectionArgs.builder()
* .clusterName("kustoCluster")
* .dataConnectionName("dataConnectionTest")
* .databaseName("KustoDatabase8")
* .resourceGroupName("kustorptest")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:kusto:EventHubDataConnection kustoCluster/KustoDatabase8/dataConnectionTest /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Kusto/clusters/{clusterName}/databases/{databaseName}/dataConnections/{dataConnectionName}
* ```
*
*/
@ResourceType(type="azure-native:kusto:EventHubDataConnection")
public class EventHubDataConnection extends com.pulumi.resources.CustomResource {
/**
* The event hub messages compression type
*
*/
@Export(name="compression", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> compression;
/**
* @return The event hub messages compression type
*
*/
public Output> compression() {
return Codegen.optional(this.compression);
}
/**
* The event hub consumer group.
*
*/
@Export(name="consumerGroup", refs={String.class}, tree="[0]")
private Output consumerGroup;
/**
* @return The event hub consumer group.
*
*/
public Output consumerGroup() {
return this.consumerGroup;
}
/**
* The data format of the message. Optionally the data format can be added to each message.
*
*/
@Export(name="dataFormat", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> dataFormat;
/**
* @return The data format of the message. Optionally the data format can be added to each message.
*
*/
public Output> dataFormat() {
return Codegen.optional(this.dataFormat);
}
/**
* Indication for database routing information from the data connection, by default only database routing information is allowed
*
*/
@Export(name="databaseRouting", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> databaseRouting;
/**
* @return Indication for database routing information from the data connection, by default only database routing information is allowed
*
*/
public Output> databaseRouting() {
return Codegen.optional(this.databaseRouting);
}
/**
* The resource ID of the event hub to be used to create a data connection.
*
*/
@Export(name="eventHubResourceId", refs={String.class}, tree="[0]")
private Output eventHubResourceId;
/**
* @return The resource ID of the event hub to be used to create a data connection.
*
*/
public Output eventHubResourceId() {
return this.eventHubResourceId;
}
/**
* System properties of the event hub
*
*/
@Export(name="eventSystemProperties", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> eventSystemProperties;
/**
* @return System properties of the event hub
*
*/
public Output>> eventSystemProperties() {
return Codegen.optional(this.eventSystemProperties);
}
/**
* Kind of the endpoint for the data connection
* Expected value is 'EventHub'.
*
*/
@Export(name="kind", refs={String.class}, tree="[0]")
private Output kind;
/**
* @return Kind of the endpoint for the data connection
* Expected value is 'EventHub'.
*
*/
public Output kind() {
return this.kind;
}
/**
* Resource location.
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> location;
/**
* @return Resource location.
*
*/
public Output> location() {
return Codegen.optional(this.location);
}
/**
* The object ID of the managedIdentityResourceId
*
*/
@Export(name="managedIdentityObjectId", refs={String.class}, tree="[0]")
private Output managedIdentityObjectId;
/**
* @return The object ID of the managedIdentityResourceId
*
*/
public Output managedIdentityObjectId() {
return this.managedIdentityObjectId;
}
/**
* The resource ID of a managed identity (system or user assigned) to be used to authenticate with event hub.
*
*/
@Export(name="managedIdentityResourceId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> managedIdentityResourceId;
/**
* @return The resource ID of a managed identity (system or user assigned) to be used to authenticate with event hub.
*
*/
public Output> managedIdentityResourceId() {
return Codegen.optional(this.managedIdentityResourceId);
}
/**
* The mapping rule to be used to ingest the data. Optionally the mapping information can be added to each message.
*
*/
@Export(name="mappingRuleName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> mappingRuleName;
/**
* @return The mapping rule to be used to ingest the data. Optionally the mapping information can be added to each message.
*
*/
public Output> mappingRuleName() {
return Codegen.optional(this.mappingRuleName);
}
/**
* The name of the resource
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the resource
*
*/
public Output name() {
return this.name;
}
/**
* The provisioned state of the resource.
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return The provisioned state of the resource.
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* When defined, the data connection retrieves existing Event hub events created since the Retrieval start date. It can only retrieve events retained by the Event hub, based on its retention period.
*
*/
@Export(name="retrievalStartDate", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> retrievalStartDate;
/**
* @return When defined, the data connection retrieves existing Event hub events created since the Retrieval start date. It can only retrieve events retained by the Event hub, based on its retention period.
*
*/
public Output> retrievalStartDate() {
return Codegen.optional(this.retrievalStartDate);
}
/**
* The table where the data should be ingested. Optionally the table information can be added to each message.
*
*/
@Export(name="tableName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> tableName;
/**
* @return The table where the data should be ingested. Optionally the table information can be added to each message.
*
*/
public Output> tableName() {
return Codegen.optional(this.tableName);
}
/**
* The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public Output type() {
return this.type;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public EventHubDataConnection(java.lang.String name) {
this(name, EventHubDataConnectionArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public EventHubDataConnection(java.lang.String name, EventHubDataConnectionArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public EventHubDataConnection(java.lang.String name, EventHubDataConnectionArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:kusto:EventHubDataConnection", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private EventHubDataConnection(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:kusto:EventHubDataConnection", name, null, makeResourceOptions(options, id), false);
}
private static EventHubDataConnectionArgs makeArgs(EventHubDataConnectionArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
var builder = args == null ? EventHubDataConnectionArgs.builder() : EventHubDataConnectionArgs.builder(args);
return builder
.kind("EventHub")
.build();
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:kusto/v20190121:EventHubDataConnection").build()),
Output.of(Alias.builder().type("azure-native:kusto/v20190515:EventHubDataConnection").build()),
Output.of(Alias.builder().type("azure-native:kusto/v20190907:EventHubDataConnection").build()),
Output.of(Alias.builder().type("azure-native:kusto/v20191109:EventHubDataConnection").build()),
Output.of(Alias.builder().type("azure-native:kusto/v20200215:EventHubDataConnection").build()),
Output.of(Alias.builder().type("azure-native:kusto/v20200614:EventHubDataConnection").build()),
Output.of(Alias.builder().type("azure-native:kusto/v20200918:EventHubDataConnection").build()),
Output.of(Alias.builder().type("azure-native:kusto/v20210101:EventHubDataConnection").build()),
Output.of(Alias.builder().type("azure-native:kusto/v20210827:EventHubDataConnection").build()),
Output.of(Alias.builder().type("azure-native:kusto/v20220201:EventHubDataConnection").build()),
Output.of(Alias.builder().type("azure-native:kusto/v20220707:EventHubDataConnection").build()),
Output.of(Alias.builder().type("azure-native:kusto/v20221111:EventHubDataConnection").build()),
Output.of(Alias.builder().type("azure-native:kusto/v20221229:EventHubDataConnection").build()),
Output.of(Alias.builder().type("azure-native:kusto/v20230502:EventHubDataConnection").build()),
Output.of(Alias.builder().type("azure-native:kusto/v20230815:EventHubDataConnection").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static EventHubDataConnection get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new EventHubDataConnection(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy