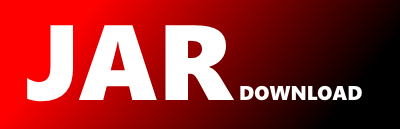
com.pulumi.azurenative.kusto.outputs.GetEventHubDataConnectionResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.kusto.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetEventHubDataConnectionResult {
/**
* @return The event hub messages compression type
*
*/
private @Nullable String compression;
/**
* @return The event hub consumer group.
*
*/
private String consumerGroup;
/**
* @return The data format of the message. Optionally the data format can be added to each message.
*
*/
private @Nullable String dataFormat;
/**
* @return Indication for database routing information from the data connection, by default only database routing information is allowed
*
*/
private @Nullable String databaseRouting;
/**
* @return The resource ID of the event hub to be used to create a data connection.
*
*/
private String eventHubResourceId;
/**
* @return System properties of the event hub
*
*/
private @Nullable List eventSystemProperties;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return Kind of the endpoint for the data connection
* Expected value is 'EventHub'.
*
*/
private String kind;
/**
* @return Resource location.
*
*/
private @Nullable String location;
/**
* @return The object ID of the managedIdentityResourceId
*
*/
private String managedIdentityObjectId;
/**
* @return The resource ID of a managed identity (system or user assigned) to be used to authenticate with event hub.
*
*/
private @Nullable String managedIdentityResourceId;
/**
* @return The mapping rule to be used to ingest the data. Optionally the mapping information can be added to each message.
*
*/
private @Nullable String mappingRuleName;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return The provisioned state of the resource.
*
*/
private String provisioningState;
/**
* @return When defined, the data connection retrieves existing Event hub events created since the Retrieval start date. It can only retrieve events retained by the Event hub, based on its retention period.
*
*/
private @Nullable String retrievalStartDate;
/**
* @return The table where the data should be ingested. Optionally the table information can be added to each message.
*
*/
private @Nullable String tableName;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
private GetEventHubDataConnectionResult() {}
/**
* @return The event hub messages compression type
*
*/
public Optional compression() {
return Optional.ofNullable(this.compression);
}
/**
* @return The event hub consumer group.
*
*/
public String consumerGroup() {
return this.consumerGroup;
}
/**
* @return The data format of the message. Optionally the data format can be added to each message.
*
*/
public Optional dataFormat() {
return Optional.ofNullable(this.dataFormat);
}
/**
* @return Indication for database routing information from the data connection, by default only database routing information is allowed
*
*/
public Optional databaseRouting() {
return Optional.ofNullable(this.databaseRouting);
}
/**
* @return The resource ID of the event hub to be used to create a data connection.
*
*/
public String eventHubResourceId() {
return this.eventHubResourceId;
}
/**
* @return System properties of the event hub
*
*/
public List eventSystemProperties() {
return this.eventSystemProperties == null ? List.of() : this.eventSystemProperties;
}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return Kind of the endpoint for the data connection
* Expected value is 'EventHub'.
*
*/
public String kind() {
return this.kind;
}
/**
* @return Resource location.
*
*/
public Optional location() {
return Optional.ofNullable(this.location);
}
/**
* @return The object ID of the managedIdentityResourceId
*
*/
public String managedIdentityObjectId() {
return this.managedIdentityObjectId;
}
/**
* @return The resource ID of a managed identity (system or user assigned) to be used to authenticate with event hub.
*
*/
public Optional managedIdentityResourceId() {
return Optional.ofNullable(this.managedIdentityResourceId);
}
/**
* @return The mapping rule to be used to ingest the data. Optionally the mapping information can be added to each message.
*
*/
public Optional mappingRuleName() {
return Optional.ofNullable(this.mappingRuleName);
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return The provisioned state of the resource.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return When defined, the data connection retrieves existing Event hub events created since the Retrieval start date. It can only retrieve events retained by the Event hub, based on its retention period.
*
*/
public Optional retrievalStartDate() {
return Optional.ofNullable(this.retrievalStartDate);
}
/**
* @return The table where the data should be ingested. Optionally the table information can be added to each message.
*
*/
public Optional tableName() {
return Optional.ofNullable(this.tableName);
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetEventHubDataConnectionResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String compression;
private String consumerGroup;
private @Nullable String dataFormat;
private @Nullable String databaseRouting;
private String eventHubResourceId;
private @Nullable List eventSystemProperties;
private String id;
private String kind;
private @Nullable String location;
private String managedIdentityObjectId;
private @Nullable String managedIdentityResourceId;
private @Nullable String mappingRuleName;
private String name;
private String provisioningState;
private @Nullable String retrievalStartDate;
private @Nullable String tableName;
private String type;
public Builder() {}
public Builder(GetEventHubDataConnectionResult defaults) {
Objects.requireNonNull(defaults);
this.compression = defaults.compression;
this.consumerGroup = defaults.consumerGroup;
this.dataFormat = defaults.dataFormat;
this.databaseRouting = defaults.databaseRouting;
this.eventHubResourceId = defaults.eventHubResourceId;
this.eventSystemProperties = defaults.eventSystemProperties;
this.id = defaults.id;
this.kind = defaults.kind;
this.location = defaults.location;
this.managedIdentityObjectId = defaults.managedIdentityObjectId;
this.managedIdentityResourceId = defaults.managedIdentityResourceId;
this.mappingRuleName = defaults.mappingRuleName;
this.name = defaults.name;
this.provisioningState = defaults.provisioningState;
this.retrievalStartDate = defaults.retrievalStartDate;
this.tableName = defaults.tableName;
this.type = defaults.type;
}
@CustomType.Setter
public Builder compression(@Nullable String compression) {
this.compression = compression;
return this;
}
@CustomType.Setter
public Builder consumerGroup(String consumerGroup) {
if (consumerGroup == null) {
throw new MissingRequiredPropertyException("GetEventHubDataConnectionResult", "consumerGroup");
}
this.consumerGroup = consumerGroup;
return this;
}
@CustomType.Setter
public Builder dataFormat(@Nullable String dataFormat) {
this.dataFormat = dataFormat;
return this;
}
@CustomType.Setter
public Builder databaseRouting(@Nullable String databaseRouting) {
this.databaseRouting = databaseRouting;
return this;
}
@CustomType.Setter
public Builder eventHubResourceId(String eventHubResourceId) {
if (eventHubResourceId == null) {
throw new MissingRequiredPropertyException("GetEventHubDataConnectionResult", "eventHubResourceId");
}
this.eventHubResourceId = eventHubResourceId;
return this;
}
@CustomType.Setter
public Builder eventSystemProperties(@Nullable List eventSystemProperties) {
this.eventSystemProperties = eventSystemProperties;
return this;
}
public Builder eventSystemProperties(String... eventSystemProperties) {
return eventSystemProperties(List.of(eventSystemProperties));
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetEventHubDataConnectionResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder kind(String kind) {
if (kind == null) {
throw new MissingRequiredPropertyException("GetEventHubDataConnectionResult", "kind");
}
this.kind = kind;
return this;
}
@CustomType.Setter
public Builder location(@Nullable String location) {
this.location = location;
return this;
}
@CustomType.Setter
public Builder managedIdentityObjectId(String managedIdentityObjectId) {
if (managedIdentityObjectId == null) {
throw new MissingRequiredPropertyException("GetEventHubDataConnectionResult", "managedIdentityObjectId");
}
this.managedIdentityObjectId = managedIdentityObjectId;
return this;
}
@CustomType.Setter
public Builder managedIdentityResourceId(@Nullable String managedIdentityResourceId) {
this.managedIdentityResourceId = managedIdentityResourceId;
return this;
}
@CustomType.Setter
public Builder mappingRuleName(@Nullable String mappingRuleName) {
this.mappingRuleName = mappingRuleName;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetEventHubDataConnectionResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetEventHubDataConnectionResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder retrievalStartDate(@Nullable String retrievalStartDate) {
this.retrievalStartDate = retrievalStartDate;
return this;
}
@CustomType.Setter
public Builder tableName(@Nullable String tableName) {
this.tableName = tableName;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetEventHubDataConnectionResult", "type");
}
this.type = type;
return this;
}
public GetEventHubDataConnectionResult build() {
final var _resultValue = new GetEventHubDataConnectionResult();
_resultValue.compression = compression;
_resultValue.consumerGroup = consumerGroup;
_resultValue.dataFormat = dataFormat;
_resultValue.databaseRouting = databaseRouting;
_resultValue.eventHubResourceId = eventHubResourceId;
_resultValue.eventSystemProperties = eventSystemProperties;
_resultValue.id = id;
_resultValue.kind = kind;
_resultValue.location = location;
_resultValue.managedIdentityObjectId = managedIdentityObjectId;
_resultValue.managedIdentityResourceId = managedIdentityResourceId;
_resultValue.mappingRuleName = mappingRuleName;
_resultValue.name = name;
_resultValue.provisioningState = provisioningState;
_resultValue.retrievalStartDate = retrievalStartDate;
_resultValue.tableName = tableName;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy