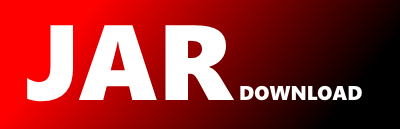
com.pulumi.azurenative.labservices.inputs.AutoShutdownProfileArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.labservices.inputs;
import com.pulumi.azurenative.labservices.enums.EnableState;
import com.pulumi.azurenative.labservices.enums.ShutdownOnIdleMode;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Profile for how to handle shutting down virtual machines.
*
*/
public final class AutoShutdownProfileArgs extends com.pulumi.resources.ResourceArgs {
public static final AutoShutdownProfileArgs Empty = new AutoShutdownProfileArgs();
/**
* The amount of time a VM will stay running after a user disconnects if this behavior is enabled.
*
*/
@Import(name="disconnectDelay")
private @Nullable Output disconnectDelay;
/**
* @return The amount of time a VM will stay running after a user disconnects if this behavior is enabled.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy