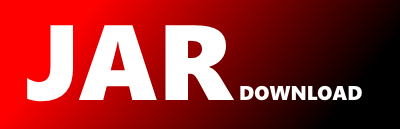
com.pulumi.azurenative.labservices.outputs.RecurrencePatternResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.labservices.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class RecurrencePatternResponse {
/**
* @return When the recurrence will expire. This date is inclusive.
*
*/
private String expirationDate;
/**
* @return The frequency of the recurrence.
*
*/
private String frequency;
/**
* @return The interval to invoke the schedule on. For example, interval = 2 and RecurrenceFrequency.Daily will run every 2 days. When no interval is supplied, an interval of 1 is used.
*
*/
private @Nullable Integer interval;
/**
* @return The week days the schedule runs. Used for when the Frequency is set to Weekly.
*
*/
private @Nullable List weekDays;
private RecurrencePatternResponse() {}
/**
* @return When the recurrence will expire. This date is inclusive.
*
*/
public String expirationDate() {
return this.expirationDate;
}
/**
* @return The frequency of the recurrence.
*
*/
public String frequency() {
return this.frequency;
}
/**
* @return The interval to invoke the schedule on. For example, interval = 2 and RecurrenceFrequency.Daily will run every 2 days. When no interval is supplied, an interval of 1 is used.
*
*/
public Optional interval() {
return Optional.ofNullable(this.interval);
}
/**
* @return The week days the schedule runs. Used for when the Frequency is set to Weekly.
*
*/
public List weekDays() {
return this.weekDays == null ? List.of() : this.weekDays;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(RecurrencePatternResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String expirationDate;
private String frequency;
private @Nullable Integer interval;
private @Nullable List weekDays;
public Builder() {}
public Builder(RecurrencePatternResponse defaults) {
Objects.requireNonNull(defaults);
this.expirationDate = defaults.expirationDate;
this.frequency = defaults.frequency;
this.interval = defaults.interval;
this.weekDays = defaults.weekDays;
}
@CustomType.Setter
public Builder expirationDate(String expirationDate) {
if (expirationDate == null) {
throw new MissingRequiredPropertyException("RecurrencePatternResponse", "expirationDate");
}
this.expirationDate = expirationDate;
return this;
}
@CustomType.Setter
public Builder frequency(String frequency) {
if (frequency == null) {
throw new MissingRequiredPropertyException("RecurrencePatternResponse", "frequency");
}
this.frequency = frequency;
return this;
}
@CustomType.Setter
public Builder interval(@Nullable Integer interval) {
this.interval = interval;
return this;
}
@CustomType.Setter
public Builder weekDays(@Nullable List weekDays) {
this.weekDays = weekDays;
return this;
}
public Builder weekDays(String... weekDays) {
return weekDays(List.of(weekDays));
}
public RecurrencePatternResponse build() {
final var _resultValue = new RecurrencePatternResponse();
_resultValue.expirationDate = expirationDate;
_resultValue.frequency = frequency;
_resultValue.interval = interval;
_resultValue.weekDays = weekDays;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy