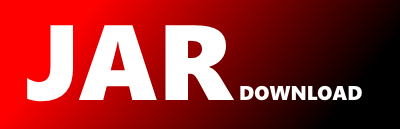
com.pulumi.azurenative.logic.inputs.AS2MdnSettingsArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.logic.inputs;
import com.pulumi.azurenative.logic.enums.HashingAlgorithm;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* The AS2 agreement mdn settings.
*
*/
public final class AS2MdnSettingsArgs extends com.pulumi.resources.ResourceArgs {
public static final AS2MdnSettingsArgs Empty = new AS2MdnSettingsArgs();
/**
* The disposition notification to header value.
*
*/
@Import(name="dispositionNotificationTo")
private @Nullable Output dispositionNotificationTo;
/**
* @return The disposition notification to header value.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy