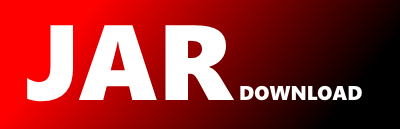
com.pulumi.azurenative.logic.inputs.AS2SecuritySettingsArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.logic.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* The AS2 agreement security settings.
*
*/
public final class AS2SecuritySettingsArgs extends com.pulumi.resources.ResourceArgs {
public static final AS2SecuritySettingsArgs Empty = new AS2SecuritySettingsArgs();
/**
* The value indicating whether to enable NRR for inbound decoded messages.
*
*/
@Import(name="enableNRRForInboundDecodedMessages", required=true)
private Output enableNRRForInboundDecodedMessages;
/**
* @return The value indicating whether to enable NRR for inbound decoded messages.
*
*/
public Output enableNRRForInboundDecodedMessages() {
return this.enableNRRForInboundDecodedMessages;
}
/**
* The value indicating whether to enable NRR for inbound encoded messages.
*
*/
@Import(name="enableNRRForInboundEncodedMessages", required=true)
private Output enableNRRForInboundEncodedMessages;
/**
* @return The value indicating whether to enable NRR for inbound encoded messages.
*
*/
public Output enableNRRForInboundEncodedMessages() {
return this.enableNRRForInboundEncodedMessages;
}
/**
* The value indicating whether to enable NRR for inbound MDN.
*
*/
@Import(name="enableNRRForInboundMDN", required=true)
private Output enableNRRForInboundMDN;
/**
* @return The value indicating whether to enable NRR for inbound MDN.
*
*/
public Output enableNRRForInboundMDN() {
return this.enableNRRForInboundMDN;
}
/**
* The value indicating whether to enable NRR for outbound decoded messages.
*
*/
@Import(name="enableNRRForOutboundDecodedMessages", required=true)
private Output enableNRRForOutboundDecodedMessages;
/**
* @return The value indicating whether to enable NRR for outbound decoded messages.
*
*/
public Output enableNRRForOutboundDecodedMessages() {
return this.enableNRRForOutboundDecodedMessages;
}
/**
* The value indicating whether to enable NRR for outbound encoded messages.
*
*/
@Import(name="enableNRRForOutboundEncodedMessages", required=true)
private Output enableNRRForOutboundEncodedMessages;
/**
* @return The value indicating whether to enable NRR for outbound encoded messages.
*
*/
public Output enableNRRForOutboundEncodedMessages() {
return this.enableNRRForOutboundEncodedMessages;
}
/**
* The value indicating whether to enable NRR for outbound MDN.
*
*/
@Import(name="enableNRRForOutboundMDN", required=true)
private Output enableNRRForOutboundMDN;
/**
* @return The value indicating whether to enable NRR for outbound MDN.
*
*/
public Output enableNRRForOutboundMDN() {
return this.enableNRRForOutboundMDN;
}
/**
* The name of the encryption certificate.
*
*/
@Import(name="encryptionCertificateName")
private @Nullable Output encryptionCertificateName;
/**
* @return The name of the encryption certificate.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy