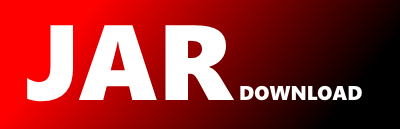
com.pulumi.azurenative.logic.inputs.X12ValidationOverrideArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.logic.inputs;
import com.pulumi.azurenative.logic.enums.TrailingSeparatorPolicy;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
/**
* The X12 validation override settings.
*
*/
public final class X12ValidationOverrideArgs extends com.pulumi.resources.ResourceArgs {
public static final X12ValidationOverrideArgs Empty = new X12ValidationOverrideArgs();
/**
* The value indicating whether to allow leading and trailing spaces and zeroes.
*
*/
@Import(name="allowLeadingAndTrailingSpacesAndZeroes", required=true)
private Output allowLeadingAndTrailingSpacesAndZeroes;
/**
* @return The value indicating whether to allow leading and trailing spaces and zeroes.
*
*/
public Output allowLeadingAndTrailingSpacesAndZeroes() {
return this.allowLeadingAndTrailingSpacesAndZeroes;
}
/**
* The message id on which the validation settings has to be applied.
*
*/
@Import(name="messageId", required=true)
private Output messageId;
/**
* @return The message id on which the validation settings has to be applied.
*
*/
public Output messageId() {
return this.messageId;
}
/**
* The trailing separator policy.
*
*/
@Import(name="trailingSeparatorPolicy", required=true)
private Output> trailingSeparatorPolicy;
/**
* @return The trailing separator policy.
*
*/
public Output> trailingSeparatorPolicy() {
return this.trailingSeparatorPolicy;
}
/**
* The value indicating whether to trim leading and trailing spaces and zeroes.
*
*/
@Import(name="trimLeadingAndTrailingSpacesAndZeroes", required=true)
private Output trimLeadingAndTrailingSpacesAndZeroes;
/**
* @return The value indicating whether to trim leading and trailing spaces and zeroes.
*
*/
public Output trimLeadingAndTrailingSpacesAndZeroes() {
return this.trimLeadingAndTrailingSpacesAndZeroes;
}
/**
* The value indicating whether to validate character Set.
*
*/
@Import(name="validateCharacterSet", required=true)
private Output validateCharacterSet;
/**
* @return The value indicating whether to validate character Set.
*
*/
public Output validateCharacterSet() {
return this.validateCharacterSet;
}
/**
* The value indicating whether to validate EDI types.
*
*/
@Import(name="validateEDITypes", required=true)
private Output validateEDITypes;
/**
* @return The value indicating whether to validate EDI types.
*
*/
public Output validateEDITypes() {
return this.validateEDITypes;
}
/**
* The value indicating whether to validate XSD types.
*
*/
@Import(name="validateXSDTypes", required=true)
private Output validateXSDTypes;
/**
* @return The value indicating whether to validate XSD types.
*
*/
public Output validateXSDTypes() {
return this.validateXSDTypes;
}
private X12ValidationOverrideArgs() {}
private X12ValidationOverrideArgs(X12ValidationOverrideArgs $) {
this.allowLeadingAndTrailingSpacesAndZeroes = $.allowLeadingAndTrailingSpacesAndZeroes;
this.messageId = $.messageId;
this.trailingSeparatorPolicy = $.trailingSeparatorPolicy;
this.trimLeadingAndTrailingSpacesAndZeroes = $.trimLeadingAndTrailingSpacesAndZeroes;
this.validateCharacterSet = $.validateCharacterSet;
this.validateEDITypes = $.validateEDITypes;
this.validateXSDTypes = $.validateXSDTypes;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(X12ValidationOverrideArgs defaults) {
return new Builder(defaults);
}
public static final class Builder {
private X12ValidationOverrideArgs $;
public Builder() {
$ = new X12ValidationOverrideArgs();
}
public Builder(X12ValidationOverrideArgs defaults) {
$ = new X12ValidationOverrideArgs(Objects.requireNonNull(defaults));
}
/**
* @param allowLeadingAndTrailingSpacesAndZeroes The value indicating whether to allow leading and trailing spaces and zeroes.
*
* @return builder
*
*/
public Builder allowLeadingAndTrailingSpacesAndZeroes(Output allowLeadingAndTrailingSpacesAndZeroes) {
$.allowLeadingAndTrailingSpacesAndZeroes = allowLeadingAndTrailingSpacesAndZeroes;
return this;
}
/**
* @param allowLeadingAndTrailingSpacesAndZeroes The value indicating whether to allow leading and trailing spaces and zeroes.
*
* @return builder
*
*/
public Builder allowLeadingAndTrailingSpacesAndZeroes(Boolean allowLeadingAndTrailingSpacesAndZeroes) {
return allowLeadingAndTrailingSpacesAndZeroes(Output.of(allowLeadingAndTrailingSpacesAndZeroes));
}
/**
* @param messageId The message id on which the validation settings has to be applied.
*
* @return builder
*
*/
public Builder messageId(Output messageId) {
$.messageId = messageId;
return this;
}
/**
* @param messageId The message id on which the validation settings has to be applied.
*
* @return builder
*
*/
public Builder messageId(String messageId) {
return messageId(Output.of(messageId));
}
/**
* @param trailingSeparatorPolicy The trailing separator policy.
*
* @return builder
*
*/
public Builder trailingSeparatorPolicy(Output> trailingSeparatorPolicy) {
$.trailingSeparatorPolicy = trailingSeparatorPolicy;
return this;
}
/**
* @param trailingSeparatorPolicy The trailing separator policy.
*
* @return builder
*
*/
public Builder trailingSeparatorPolicy(Either trailingSeparatorPolicy) {
return trailingSeparatorPolicy(Output.of(trailingSeparatorPolicy));
}
/**
* @param trailingSeparatorPolicy The trailing separator policy.
*
* @return builder
*
*/
public Builder trailingSeparatorPolicy(String trailingSeparatorPolicy) {
return trailingSeparatorPolicy(Either.ofLeft(trailingSeparatorPolicy));
}
/**
* @param trailingSeparatorPolicy The trailing separator policy.
*
* @return builder
*
*/
public Builder trailingSeparatorPolicy(TrailingSeparatorPolicy trailingSeparatorPolicy) {
return trailingSeparatorPolicy(Either.ofRight(trailingSeparatorPolicy));
}
/**
* @param trimLeadingAndTrailingSpacesAndZeroes The value indicating whether to trim leading and trailing spaces and zeroes.
*
* @return builder
*
*/
public Builder trimLeadingAndTrailingSpacesAndZeroes(Output trimLeadingAndTrailingSpacesAndZeroes) {
$.trimLeadingAndTrailingSpacesAndZeroes = trimLeadingAndTrailingSpacesAndZeroes;
return this;
}
/**
* @param trimLeadingAndTrailingSpacesAndZeroes The value indicating whether to trim leading and trailing spaces and zeroes.
*
* @return builder
*
*/
public Builder trimLeadingAndTrailingSpacesAndZeroes(Boolean trimLeadingAndTrailingSpacesAndZeroes) {
return trimLeadingAndTrailingSpacesAndZeroes(Output.of(trimLeadingAndTrailingSpacesAndZeroes));
}
/**
* @param validateCharacterSet The value indicating whether to validate character Set.
*
* @return builder
*
*/
public Builder validateCharacterSet(Output validateCharacterSet) {
$.validateCharacterSet = validateCharacterSet;
return this;
}
/**
* @param validateCharacterSet The value indicating whether to validate character Set.
*
* @return builder
*
*/
public Builder validateCharacterSet(Boolean validateCharacterSet) {
return validateCharacterSet(Output.of(validateCharacterSet));
}
/**
* @param validateEDITypes The value indicating whether to validate EDI types.
*
* @return builder
*
*/
public Builder validateEDITypes(Output validateEDITypes) {
$.validateEDITypes = validateEDITypes;
return this;
}
/**
* @param validateEDITypes The value indicating whether to validate EDI types.
*
* @return builder
*
*/
public Builder validateEDITypes(Boolean validateEDITypes) {
return validateEDITypes(Output.of(validateEDITypes));
}
/**
* @param validateXSDTypes The value indicating whether to validate XSD types.
*
* @return builder
*
*/
public Builder validateXSDTypes(Output validateXSDTypes) {
$.validateXSDTypes = validateXSDTypes;
return this;
}
/**
* @param validateXSDTypes The value indicating whether to validate XSD types.
*
* @return builder
*
*/
public Builder validateXSDTypes(Boolean validateXSDTypes) {
return validateXSDTypes(Output.of(validateXSDTypes));
}
public X12ValidationOverrideArgs build() {
if ($.allowLeadingAndTrailingSpacesAndZeroes == null) {
throw new MissingRequiredPropertyException("X12ValidationOverrideArgs", "allowLeadingAndTrailingSpacesAndZeroes");
}
if ($.messageId == null) {
throw new MissingRequiredPropertyException("X12ValidationOverrideArgs", "messageId");
}
if ($.trailingSeparatorPolicy == null) {
throw new MissingRequiredPropertyException("X12ValidationOverrideArgs", "trailingSeparatorPolicy");
}
if ($.trimLeadingAndTrailingSpacesAndZeroes == null) {
throw new MissingRequiredPropertyException("X12ValidationOverrideArgs", "trimLeadingAndTrailingSpacesAndZeroes");
}
if ($.validateCharacterSet == null) {
throw new MissingRequiredPropertyException("X12ValidationOverrideArgs", "validateCharacterSet");
}
if ($.validateEDITypes == null) {
throw new MissingRequiredPropertyException("X12ValidationOverrideArgs", "validateEDITypes");
}
if ($.validateXSDTypes == null) {
throw new MissingRequiredPropertyException("X12ValidationOverrideArgs", "validateXSDTypes");
}
return $;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy