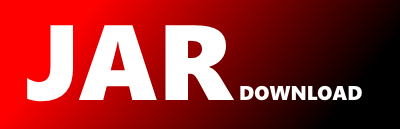
com.pulumi.azurenative.logic.outputs.EdifactSchemaReferenceResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.logic.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class EdifactSchemaReferenceResponse {
/**
* @return The association assigned code.
*
*/
private @Nullable String associationAssignedCode;
/**
* @return The message id.
*
*/
private String messageId;
/**
* @return The message release version.
*
*/
private String messageRelease;
/**
* @return The message version.
*
*/
private String messageVersion;
/**
* @return The schema name.
*
*/
private String schemaName;
/**
* @return The sender application id.
*
*/
private @Nullable String senderApplicationId;
/**
* @return The sender application qualifier.
*
*/
private @Nullable String senderApplicationQualifier;
private EdifactSchemaReferenceResponse() {}
/**
* @return The association assigned code.
*
*/
public Optional associationAssignedCode() {
return Optional.ofNullable(this.associationAssignedCode);
}
/**
* @return The message id.
*
*/
public String messageId() {
return this.messageId;
}
/**
* @return The message release version.
*
*/
public String messageRelease() {
return this.messageRelease;
}
/**
* @return The message version.
*
*/
public String messageVersion() {
return this.messageVersion;
}
/**
* @return The schema name.
*
*/
public String schemaName() {
return this.schemaName;
}
/**
* @return The sender application id.
*
*/
public Optional senderApplicationId() {
return Optional.ofNullable(this.senderApplicationId);
}
/**
* @return The sender application qualifier.
*
*/
public Optional senderApplicationQualifier() {
return Optional.ofNullable(this.senderApplicationQualifier);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(EdifactSchemaReferenceResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String associationAssignedCode;
private String messageId;
private String messageRelease;
private String messageVersion;
private String schemaName;
private @Nullable String senderApplicationId;
private @Nullable String senderApplicationQualifier;
public Builder() {}
public Builder(EdifactSchemaReferenceResponse defaults) {
Objects.requireNonNull(defaults);
this.associationAssignedCode = defaults.associationAssignedCode;
this.messageId = defaults.messageId;
this.messageRelease = defaults.messageRelease;
this.messageVersion = defaults.messageVersion;
this.schemaName = defaults.schemaName;
this.senderApplicationId = defaults.senderApplicationId;
this.senderApplicationQualifier = defaults.senderApplicationQualifier;
}
@CustomType.Setter
public Builder associationAssignedCode(@Nullable String associationAssignedCode) {
this.associationAssignedCode = associationAssignedCode;
return this;
}
@CustomType.Setter
public Builder messageId(String messageId) {
if (messageId == null) {
throw new MissingRequiredPropertyException("EdifactSchemaReferenceResponse", "messageId");
}
this.messageId = messageId;
return this;
}
@CustomType.Setter
public Builder messageRelease(String messageRelease) {
if (messageRelease == null) {
throw new MissingRequiredPropertyException("EdifactSchemaReferenceResponse", "messageRelease");
}
this.messageRelease = messageRelease;
return this;
}
@CustomType.Setter
public Builder messageVersion(String messageVersion) {
if (messageVersion == null) {
throw new MissingRequiredPropertyException("EdifactSchemaReferenceResponse", "messageVersion");
}
this.messageVersion = messageVersion;
return this;
}
@CustomType.Setter
public Builder schemaName(String schemaName) {
if (schemaName == null) {
throw new MissingRequiredPropertyException("EdifactSchemaReferenceResponse", "schemaName");
}
this.schemaName = schemaName;
return this;
}
@CustomType.Setter
public Builder senderApplicationId(@Nullable String senderApplicationId) {
this.senderApplicationId = senderApplicationId;
return this;
}
@CustomType.Setter
public Builder senderApplicationQualifier(@Nullable String senderApplicationQualifier) {
this.senderApplicationQualifier = senderApplicationQualifier;
return this;
}
public EdifactSchemaReferenceResponse build() {
final var _resultValue = new EdifactSchemaReferenceResponse();
_resultValue.associationAssignedCode = associationAssignedCode;
_resultValue.messageId = messageId;
_resultValue.messageRelease = messageRelease;
_resultValue.messageVersion = messageVersion;
_resultValue.schemaName = schemaName;
_resultValue.senderApplicationId = senderApplicationId;
_resultValue.senderApplicationQualifier = senderApplicationQualifier;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy