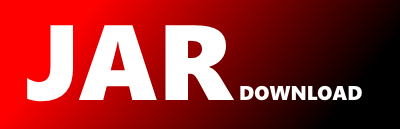
com.pulumi.azurenative.logic.outputs.X12ValidationSettingsResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.logic.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class X12ValidationSettingsResponse {
/**
* @return The value indicating whether to allow leading and trailing spaces and zeroes.
*
*/
private Boolean allowLeadingAndTrailingSpacesAndZeroes;
/**
* @return The value indicating whether to check for duplicate group control number.
*
*/
private Boolean checkDuplicateGroupControlNumber;
/**
* @return The value indicating whether to check for duplicate interchange control number.
*
*/
private Boolean checkDuplicateInterchangeControlNumber;
/**
* @return The value indicating whether to check for duplicate transaction set control number.
*
*/
private Boolean checkDuplicateTransactionSetControlNumber;
/**
* @return The validity period of interchange control number.
*
*/
private Integer interchangeControlNumberValidityDays;
/**
* @return The trailing separator policy.
*
*/
private String trailingSeparatorPolicy;
/**
* @return The value indicating whether to trim leading and trailing spaces and zeroes.
*
*/
private Boolean trimLeadingAndTrailingSpacesAndZeroes;
/**
* @return The value indicating whether to validate character set in the message.
*
*/
private Boolean validateCharacterSet;
/**
* @return The value indicating whether to Whether to validate EDI types.
*
*/
private Boolean validateEDITypes;
/**
* @return The value indicating whether to Whether to validate XSD types.
*
*/
private Boolean validateXSDTypes;
private X12ValidationSettingsResponse() {}
/**
* @return The value indicating whether to allow leading and trailing spaces and zeroes.
*
*/
public Boolean allowLeadingAndTrailingSpacesAndZeroes() {
return this.allowLeadingAndTrailingSpacesAndZeroes;
}
/**
* @return The value indicating whether to check for duplicate group control number.
*
*/
public Boolean checkDuplicateGroupControlNumber() {
return this.checkDuplicateGroupControlNumber;
}
/**
* @return The value indicating whether to check for duplicate interchange control number.
*
*/
public Boolean checkDuplicateInterchangeControlNumber() {
return this.checkDuplicateInterchangeControlNumber;
}
/**
* @return The value indicating whether to check for duplicate transaction set control number.
*
*/
public Boolean checkDuplicateTransactionSetControlNumber() {
return this.checkDuplicateTransactionSetControlNumber;
}
/**
* @return The validity period of interchange control number.
*
*/
public Integer interchangeControlNumberValidityDays() {
return this.interchangeControlNumberValidityDays;
}
/**
* @return The trailing separator policy.
*
*/
public String trailingSeparatorPolicy() {
return this.trailingSeparatorPolicy;
}
/**
* @return The value indicating whether to trim leading and trailing spaces and zeroes.
*
*/
public Boolean trimLeadingAndTrailingSpacesAndZeroes() {
return this.trimLeadingAndTrailingSpacesAndZeroes;
}
/**
* @return The value indicating whether to validate character set in the message.
*
*/
public Boolean validateCharacterSet() {
return this.validateCharacterSet;
}
/**
* @return The value indicating whether to Whether to validate EDI types.
*
*/
public Boolean validateEDITypes() {
return this.validateEDITypes;
}
/**
* @return The value indicating whether to Whether to validate XSD types.
*
*/
public Boolean validateXSDTypes() {
return this.validateXSDTypes;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(X12ValidationSettingsResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Boolean allowLeadingAndTrailingSpacesAndZeroes;
private Boolean checkDuplicateGroupControlNumber;
private Boolean checkDuplicateInterchangeControlNumber;
private Boolean checkDuplicateTransactionSetControlNumber;
private Integer interchangeControlNumberValidityDays;
private String trailingSeparatorPolicy;
private Boolean trimLeadingAndTrailingSpacesAndZeroes;
private Boolean validateCharacterSet;
private Boolean validateEDITypes;
private Boolean validateXSDTypes;
public Builder() {}
public Builder(X12ValidationSettingsResponse defaults) {
Objects.requireNonNull(defaults);
this.allowLeadingAndTrailingSpacesAndZeroes = defaults.allowLeadingAndTrailingSpacesAndZeroes;
this.checkDuplicateGroupControlNumber = defaults.checkDuplicateGroupControlNumber;
this.checkDuplicateInterchangeControlNumber = defaults.checkDuplicateInterchangeControlNumber;
this.checkDuplicateTransactionSetControlNumber = defaults.checkDuplicateTransactionSetControlNumber;
this.interchangeControlNumberValidityDays = defaults.interchangeControlNumberValidityDays;
this.trailingSeparatorPolicy = defaults.trailingSeparatorPolicy;
this.trimLeadingAndTrailingSpacesAndZeroes = defaults.trimLeadingAndTrailingSpacesAndZeroes;
this.validateCharacterSet = defaults.validateCharacterSet;
this.validateEDITypes = defaults.validateEDITypes;
this.validateXSDTypes = defaults.validateXSDTypes;
}
@CustomType.Setter
public Builder allowLeadingAndTrailingSpacesAndZeroes(Boolean allowLeadingAndTrailingSpacesAndZeroes) {
if (allowLeadingAndTrailingSpacesAndZeroes == null) {
throw new MissingRequiredPropertyException("X12ValidationSettingsResponse", "allowLeadingAndTrailingSpacesAndZeroes");
}
this.allowLeadingAndTrailingSpacesAndZeroes = allowLeadingAndTrailingSpacesAndZeroes;
return this;
}
@CustomType.Setter
public Builder checkDuplicateGroupControlNumber(Boolean checkDuplicateGroupControlNumber) {
if (checkDuplicateGroupControlNumber == null) {
throw new MissingRequiredPropertyException("X12ValidationSettingsResponse", "checkDuplicateGroupControlNumber");
}
this.checkDuplicateGroupControlNumber = checkDuplicateGroupControlNumber;
return this;
}
@CustomType.Setter
public Builder checkDuplicateInterchangeControlNumber(Boolean checkDuplicateInterchangeControlNumber) {
if (checkDuplicateInterchangeControlNumber == null) {
throw new MissingRequiredPropertyException("X12ValidationSettingsResponse", "checkDuplicateInterchangeControlNumber");
}
this.checkDuplicateInterchangeControlNumber = checkDuplicateInterchangeControlNumber;
return this;
}
@CustomType.Setter
public Builder checkDuplicateTransactionSetControlNumber(Boolean checkDuplicateTransactionSetControlNumber) {
if (checkDuplicateTransactionSetControlNumber == null) {
throw new MissingRequiredPropertyException("X12ValidationSettingsResponse", "checkDuplicateTransactionSetControlNumber");
}
this.checkDuplicateTransactionSetControlNumber = checkDuplicateTransactionSetControlNumber;
return this;
}
@CustomType.Setter
public Builder interchangeControlNumberValidityDays(Integer interchangeControlNumberValidityDays) {
if (interchangeControlNumberValidityDays == null) {
throw new MissingRequiredPropertyException("X12ValidationSettingsResponse", "interchangeControlNumberValidityDays");
}
this.interchangeControlNumberValidityDays = interchangeControlNumberValidityDays;
return this;
}
@CustomType.Setter
public Builder trailingSeparatorPolicy(String trailingSeparatorPolicy) {
if (trailingSeparatorPolicy == null) {
throw new MissingRequiredPropertyException("X12ValidationSettingsResponse", "trailingSeparatorPolicy");
}
this.trailingSeparatorPolicy = trailingSeparatorPolicy;
return this;
}
@CustomType.Setter
public Builder trimLeadingAndTrailingSpacesAndZeroes(Boolean trimLeadingAndTrailingSpacesAndZeroes) {
if (trimLeadingAndTrailingSpacesAndZeroes == null) {
throw new MissingRequiredPropertyException("X12ValidationSettingsResponse", "trimLeadingAndTrailingSpacesAndZeroes");
}
this.trimLeadingAndTrailingSpacesAndZeroes = trimLeadingAndTrailingSpacesAndZeroes;
return this;
}
@CustomType.Setter
public Builder validateCharacterSet(Boolean validateCharacterSet) {
if (validateCharacterSet == null) {
throw new MissingRequiredPropertyException("X12ValidationSettingsResponse", "validateCharacterSet");
}
this.validateCharacterSet = validateCharacterSet;
return this;
}
@CustomType.Setter
public Builder validateEDITypes(Boolean validateEDITypes) {
if (validateEDITypes == null) {
throw new MissingRequiredPropertyException("X12ValidationSettingsResponse", "validateEDITypes");
}
this.validateEDITypes = validateEDITypes;
return this;
}
@CustomType.Setter
public Builder validateXSDTypes(Boolean validateXSDTypes) {
if (validateXSDTypes == null) {
throw new MissingRequiredPropertyException("X12ValidationSettingsResponse", "validateXSDTypes");
}
this.validateXSDTypes = validateXSDTypes;
return this;
}
public X12ValidationSettingsResponse build() {
final var _resultValue = new X12ValidationSettingsResponse();
_resultValue.allowLeadingAndTrailingSpacesAndZeroes = allowLeadingAndTrailingSpacesAndZeroes;
_resultValue.checkDuplicateGroupControlNumber = checkDuplicateGroupControlNumber;
_resultValue.checkDuplicateInterchangeControlNumber = checkDuplicateInterchangeControlNumber;
_resultValue.checkDuplicateTransactionSetControlNumber = checkDuplicateTransactionSetControlNumber;
_resultValue.interchangeControlNumberValidityDays = interchangeControlNumberValidityDays;
_resultValue.trailingSeparatorPolicy = trailingSeparatorPolicy;
_resultValue.trimLeadingAndTrailingSpacesAndZeroes = trimLeadingAndTrailingSpacesAndZeroes;
_resultValue.validateCharacterSet = validateCharacterSet;
_resultValue.validateEDITypes = validateEDITypes;
_resultValue.validateXSDTypes = validateXSDTypes;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy