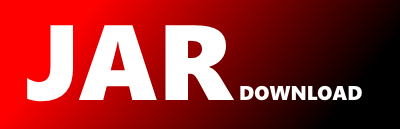
com.pulumi.azurenative.machinelearning.outputs.CommitmentPlanPropertiesResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.machinelearning.outputs;
import com.pulumi.azurenative.machinelearning.outputs.PlanQuantityResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
@CustomType
public final class CommitmentPlanPropertiesResponse {
/**
* @return Indicates whether usage beyond the commitment plan's included quantities will be charged.
*
*/
private Boolean chargeForOverage;
/**
* @return Indicates whether the commitment plan will incur a charge.
*
*/
private Boolean chargeForPlan;
/**
* @return The date at which this commitment plan was created, in ISO 8601 format.
*
*/
private String creationDate;
/**
* @return The included resource quantities this plan gives you.
*
*/
private Map includedQuantities;
/**
* @return The maximum number of commitment associations that can be children of this commitment plan.
*
*/
private Integer maxAssociationLimit;
/**
* @return The maximum scale-out capacity for this commitment plan.
*
*/
private Integer maxCapacityLimit;
/**
* @return The minimum scale-out capacity for this commitment plan.
*
*/
private Integer minCapacityLimit;
/**
* @return The Azure meter which will be used to charge for this commitment plan.
*
*/
private String planMeter;
/**
* @return The frequency at which this commitment plan's included quantities are refilled.
*
*/
private Integer refillFrequencyInDays;
/**
* @return Indicates whether this commitment plan will be moved into a suspended state if usage goes beyond the commitment plan's included quantities.
*
*/
private Boolean suspendPlanOnOverage;
private CommitmentPlanPropertiesResponse() {}
/**
* @return Indicates whether usage beyond the commitment plan's included quantities will be charged.
*
*/
public Boolean chargeForOverage() {
return this.chargeForOverage;
}
/**
* @return Indicates whether the commitment plan will incur a charge.
*
*/
public Boolean chargeForPlan() {
return this.chargeForPlan;
}
/**
* @return The date at which this commitment plan was created, in ISO 8601 format.
*
*/
public String creationDate() {
return this.creationDate;
}
/**
* @return The included resource quantities this plan gives you.
*
*/
public Map includedQuantities() {
return this.includedQuantities;
}
/**
* @return The maximum number of commitment associations that can be children of this commitment plan.
*
*/
public Integer maxAssociationLimit() {
return this.maxAssociationLimit;
}
/**
* @return The maximum scale-out capacity for this commitment plan.
*
*/
public Integer maxCapacityLimit() {
return this.maxCapacityLimit;
}
/**
* @return The minimum scale-out capacity for this commitment plan.
*
*/
public Integer minCapacityLimit() {
return this.minCapacityLimit;
}
/**
* @return The Azure meter which will be used to charge for this commitment plan.
*
*/
public String planMeter() {
return this.planMeter;
}
/**
* @return The frequency at which this commitment plan's included quantities are refilled.
*
*/
public Integer refillFrequencyInDays() {
return this.refillFrequencyInDays;
}
/**
* @return Indicates whether this commitment plan will be moved into a suspended state if usage goes beyond the commitment plan's included quantities.
*
*/
public Boolean suspendPlanOnOverage() {
return this.suspendPlanOnOverage;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(CommitmentPlanPropertiesResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Boolean chargeForOverage;
private Boolean chargeForPlan;
private String creationDate;
private Map includedQuantities;
private Integer maxAssociationLimit;
private Integer maxCapacityLimit;
private Integer minCapacityLimit;
private String planMeter;
private Integer refillFrequencyInDays;
private Boolean suspendPlanOnOverage;
public Builder() {}
public Builder(CommitmentPlanPropertiesResponse defaults) {
Objects.requireNonNull(defaults);
this.chargeForOverage = defaults.chargeForOverage;
this.chargeForPlan = defaults.chargeForPlan;
this.creationDate = defaults.creationDate;
this.includedQuantities = defaults.includedQuantities;
this.maxAssociationLimit = defaults.maxAssociationLimit;
this.maxCapacityLimit = defaults.maxCapacityLimit;
this.minCapacityLimit = defaults.minCapacityLimit;
this.planMeter = defaults.planMeter;
this.refillFrequencyInDays = defaults.refillFrequencyInDays;
this.suspendPlanOnOverage = defaults.suspendPlanOnOverage;
}
@CustomType.Setter
public Builder chargeForOverage(Boolean chargeForOverage) {
if (chargeForOverage == null) {
throw new MissingRequiredPropertyException("CommitmentPlanPropertiesResponse", "chargeForOverage");
}
this.chargeForOverage = chargeForOverage;
return this;
}
@CustomType.Setter
public Builder chargeForPlan(Boolean chargeForPlan) {
if (chargeForPlan == null) {
throw new MissingRequiredPropertyException("CommitmentPlanPropertiesResponse", "chargeForPlan");
}
this.chargeForPlan = chargeForPlan;
return this;
}
@CustomType.Setter
public Builder creationDate(String creationDate) {
if (creationDate == null) {
throw new MissingRequiredPropertyException("CommitmentPlanPropertiesResponse", "creationDate");
}
this.creationDate = creationDate;
return this;
}
@CustomType.Setter
public Builder includedQuantities(Map includedQuantities) {
if (includedQuantities == null) {
throw new MissingRequiredPropertyException("CommitmentPlanPropertiesResponse", "includedQuantities");
}
this.includedQuantities = includedQuantities;
return this;
}
@CustomType.Setter
public Builder maxAssociationLimit(Integer maxAssociationLimit) {
if (maxAssociationLimit == null) {
throw new MissingRequiredPropertyException("CommitmentPlanPropertiesResponse", "maxAssociationLimit");
}
this.maxAssociationLimit = maxAssociationLimit;
return this;
}
@CustomType.Setter
public Builder maxCapacityLimit(Integer maxCapacityLimit) {
if (maxCapacityLimit == null) {
throw new MissingRequiredPropertyException("CommitmentPlanPropertiesResponse", "maxCapacityLimit");
}
this.maxCapacityLimit = maxCapacityLimit;
return this;
}
@CustomType.Setter
public Builder minCapacityLimit(Integer minCapacityLimit) {
if (minCapacityLimit == null) {
throw new MissingRequiredPropertyException("CommitmentPlanPropertiesResponse", "minCapacityLimit");
}
this.minCapacityLimit = minCapacityLimit;
return this;
}
@CustomType.Setter
public Builder planMeter(String planMeter) {
if (planMeter == null) {
throw new MissingRequiredPropertyException("CommitmentPlanPropertiesResponse", "planMeter");
}
this.planMeter = planMeter;
return this;
}
@CustomType.Setter
public Builder refillFrequencyInDays(Integer refillFrequencyInDays) {
if (refillFrequencyInDays == null) {
throw new MissingRequiredPropertyException("CommitmentPlanPropertiesResponse", "refillFrequencyInDays");
}
this.refillFrequencyInDays = refillFrequencyInDays;
return this;
}
@CustomType.Setter
public Builder suspendPlanOnOverage(Boolean suspendPlanOnOverage) {
if (suspendPlanOnOverage == null) {
throw new MissingRequiredPropertyException("CommitmentPlanPropertiesResponse", "suspendPlanOnOverage");
}
this.suspendPlanOnOverage = suspendPlanOnOverage;
return this;
}
public CommitmentPlanPropertiesResponse build() {
final var _resultValue = new CommitmentPlanPropertiesResponse();
_resultValue.chargeForOverage = chargeForOverage;
_resultValue.chargeForPlan = chargeForPlan;
_resultValue.creationDate = creationDate;
_resultValue.includedQuantities = includedQuantities;
_resultValue.maxAssociationLimit = maxAssociationLimit;
_resultValue.maxCapacityLimit = maxCapacityLimit;
_resultValue.minCapacityLimit = minCapacityLimit;
_resultValue.planMeter = planMeter;
_resultValue.refillFrequencyInDays = refillFrequencyInDays;
_resultValue.suspendPlanOnOverage = suspendPlanOnOverage;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy