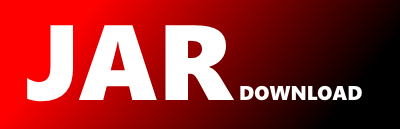
com.pulumi.azurenative.machinelearning.outputs.GetWorkspaceResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.machinelearning.outputs;
import com.pulumi.azurenative.machinelearning.outputs.SkuResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetWorkspaceResult {
/**
* @return The creation time for this workspace resource.
*
*/
private String creationTime;
/**
* @return The resource ID.
*
*/
private String id;
/**
* @return The key vault identifier used for encrypted workspaces.
*
*/
private @Nullable String keyVaultIdentifierId;
/**
* @return The location of the resource. This cannot be changed after the resource is created.
*
*/
private String location;
/**
* @return The name of the resource.
*
*/
private String name;
/**
* @return The email id of the owner for this workspace.
*
*/
private String ownerEmail;
/**
* @return The sku of the workspace.
*
*/
private @Nullable SkuResponse sku;
/**
* @return The regional endpoint for the machine learning studio service which hosts this workspace.
*
*/
private String studioEndpoint;
/**
* @return The tags of the resource.
*
*/
private @Nullable Map tags;
/**
* @return The type of the resource.
*
*/
private String type;
/**
* @return The fully qualified arm id of the storage account associated with this workspace.
*
*/
private String userStorageAccountId;
/**
* @return The immutable id associated with this workspace.
*
*/
private String workspaceId;
/**
* @return The current state of workspace resource.
*
*/
private String workspaceState;
/**
* @return The type of this workspace.
*
*/
private String workspaceType;
private GetWorkspaceResult() {}
/**
* @return The creation time for this workspace resource.
*
*/
public String creationTime() {
return this.creationTime;
}
/**
* @return The resource ID.
*
*/
public String id() {
return this.id;
}
/**
* @return The key vault identifier used for encrypted workspaces.
*
*/
public Optional keyVaultIdentifierId() {
return Optional.ofNullable(this.keyVaultIdentifierId);
}
/**
* @return The location of the resource. This cannot be changed after the resource is created.
*
*/
public String location() {
return this.location;
}
/**
* @return The name of the resource.
*
*/
public String name() {
return this.name;
}
/**
* @return The email id of the owner for this workspace.
*
*/
public String ownerEmail() {
return this.ownerEmail;
}
/**
* @return The sku of the workspace.
*
*/
public Optional sku() {
return Optional.ofNullable(this.sku);
}
/**
* @return The regional endpoint for the machine learning studio service which hosts this workspace.
*
*/
public String studioEndpoint() {
return this.studioEndpoint;
}
/**
* @return The tags of the resource.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return The type of the resource.
*
*/
public String type() {
return this.type;
}
/**
* @return The fully qualified arm id of the storage account associated with this workspace.
*
*/
public String userStorageAccountId() {
return this.userStorageAccountId;
}
/**
* @return The immutable id associated with this workspace.
*
*/
public String workspaceId() {
return this.workspaceId;
}
/**
* @return The current state of workspace resource.
*
*/
public String workspaceState() {
return this.workspaceState;
}
/**
* @return The type of this workspace.
*
*/
public String workspaceType() {
return this.workspaceType;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetWorkspaceResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String creationTime;
private String id;
private @Nullable String keyVaultIdentifierId;
private String location;
private String name;
private String ownerEmail;
private @Nullable SkuResponse sku;
private String studioEndpoint;
private @Nullable Map tags;
private String type;
private String userStorageAccountId;
private String workspaceId;
private String workspaceState;
private String workspaceType;
public Builder() {}
public Builder(GetWorkspaceResult defaults) {
Objects.requireNonNull(defaults);
this.creationTime = defaults.creationTime;
this.id = defaults.id;
this.keyVaultIdentifierId = defaults.keyVaultIdentifierId;
this.location = defaults.location;
this.name = defaults.name;
this.ownerEmail = defaults.ownerEmail;
this.sku = defaults.sku;
this.studioEndpoint = defaults.studioEndpoint;
this.tags = defaults.tags;
this.type = defaults.type;
this.userStorageAccountId = defaults.userStorageAccountId;
this.workspaceId = defaults.workspaceId;
this.workspaceState = defaults.workspaceState;
this.workspaceType = defaults.workspaceType;
}
@CustomType.Setter
public Builder creationTime(String creationTime) {
if (creationTime == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "creationTime");
}
this.creationTime = creationTime;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder keyVaultIdentifierId(@Nullable String keyVaultIdentifierId) {
this.keyVaultIdentifierId = keyVaultIdentifierId;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder ownerEmail(String ownerEmail) {
if (ownerEmail == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "ownerEmail");
}
this.ownerEmail = ownerEmail;
return this;
}
@CustomType.Setter
public Builder sku(@Nullable SkuResponse sku) {
this.sku = sku;
return this;
}
@CustomType.Setter
public Builder studioEndpoint(String studioEndpoint) {
if (studioEndpoint == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "studioEndpoint");
}
this.studioEndpoint = studioEndpoint;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder userStorageAccountId(String userStorageAccountId) {
if (userStorageAccountId == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "userStorageAccountId");
}
this.userStorageAccountId = userStorageAccountId;
return this;
}
@CustomType.Setter
public Builder workspaceId(String workspaceId) {
if (workspaceId == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "workspaceId");
}
this.workspaceId = workspaceId;
return this;
}
@CustomType.Setter
public Builder workspaceState(String workspaceState) {
if (workspaceState == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "workspaceState");
}
this.workspaceState = workspaceState;
return this;
}
@CustomType.Setter
public Builder workspaceType(String workspaceType) {
if (workspaceType == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "workspaceType");
}
this.workspaceType = workspaceType;
return this;
}
public GetWorkspaceResult build() {
final var _resultValue = new GetWorkspaceResult();
_resultValue.creationTime = creationTime;
_resultValue.id = id;
_resultValue.keyVaultIdentifierId = keyVaultIdentifierId;
_resultValue.location = location;
_resultValue.name = name;
_resultValue.ownerEmail = ownerEmail;
_resultValue.sku = sku;
_resultValue.studioEndpoint = studioEndpoint;
_resultValue.tags = tags;
_resultValue.type = type;
_resultValue.userStorageAccountId = userStorageAccountId;
_resultValue.workspaceId = workspaceId;
_resultValue.workspaceState = workspaceState;
_resultValue.workspaceType = workspaceType;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy