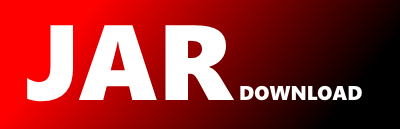
com.pulumi.azurenative.machinelearningservices.outputs.ClassificationTrainingSettingsResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.machinelearningservices.outputs;
import com.pulumi.azurenative.machinelearningservices.outputs.StackEnsembleSettingsResponse;
import com.pulumi.core.annotations.CustomType;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ClassificationTrainingSettingsResponse {
/**
* @return Allowed models for classification task.
*
*/
private @Nullable List allowedTrainingAlgorithms;
/**
* @return Blocked models for classification task.
*
*/
private @Nullable List blockedTrainingAlgorithms;
/**
* @return Enable recommendation of DNN models.
*
*/
private @Nullable Boolean enableDnnTraining;
/**
* @return Flag to turn on explainability on best model.
*
*/
private @Nullable Boolean enableModelExplainability;
/**
* @return Flag for enabling onnx compatible models.
*
*/
private @Nullable Boolean enableOnnxCompatibleModels;
/**
* @return Enable stack ensemble run.
*
*/
private @Nullable Boolean enableStackEnsemble;
/**
* @return Enable voting ensemble run.
*
*/
private @Nullable Boolean enableVoteEnsemble;
/**
* @return During VotingEnsemble and StackEnsemble model generation, multiple fitted models from the previous child runs are downloaded.
* Configure this parameter with a higher value than 300 secs, if more time is needed.
*
*/
private @Nullable String ensembleModelDownloadTimeout;
/**
* @return Stack ensemble settings for stack ensemble run.
*
*/
private @Nullable StackEnsembleSettingsResponse stackEnsembleSettings;
private ClassificationTrainingSettingsResponse() {}
/**
* @return Allowed models for classification task.
*
*/
public List allowedTrainingAlgorithms() {
return this.allowedTrainingAlgorithms == null ? List.of() : this.allowedTrainingAlgorithms;
}
/**
* @return Blocked models for classification task.
*
*/
public List blockedTrainingAlgorithms() {
return this.blockedTrainingAlgorithms == null ? List.of() : this.blockedTrainingAlgorithms;
}
/**
* @return Enable recommendation of DNN models.
*
*/
public Optional enableDnnTraining() {
return Optional.ofNullable(this.enableDnnTraining);
}
/**
* @return Flag to turn on explainability on best model.
*
*/
public Optional enableModelExplainability() {
return Optional.ofNullable(this.enableModelExplainability);
}
/**
* @return Flag for enabling onnx compatible models.
*
*/
public Optional enableOnnxCompatibleModels() {
return Optional.ofNullable(this.enableOnnxCompatibleModels);
}
/**
* @return Enable stack ensemble run.
*
*/
public Optional enableStackEnsemble() {
return Optional.ofNullable(this.enableStackEnsemble);
}
/**
* @return Enable voting ensemble run.
*
*/
public Optional enableVoteEnsemble() {
return Optional.ofNullable(this.enableVoteEnsemble);
}
/**
* @return During VotingEnsemble and StackEnsemble model generation, multiple fitted models from the previous child runs are downloaded.
* Configure this parameter with a higher value than 300 secs, if more time is needed.
*
*/
public Optional ensembleModelDownloadTimeout() {
return Optional.ofNullable(this.ensembleModelDownloadTimeout);
}
/**
* @return Stack ensemble settings for stack ensemble run.
*
*/
public Optional stackEnsembleSettings() {
return Optional.ofNullable(this.stackEnsembleSettings);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ClassificationTrainingSettingsResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List allowedTrainingAlgorithms;
private @Nullable List blockedTrainingAlgorithms;
private @Nullable Boolean enableDnnTraining;
private @Nullable Boolean enableModelExplainability;
private @Nullable Boolean enableOnnxCompatibleModels;
private @Nullable Boolean enableStackEnsemble;
private @Nullable Boolean enableVoteEnsemble;
private @Nullable String ensembleModelDownloadTimeout;
private @Nullable StackEnsembleSettingsResponse stackEnsembleSettings;
public Builder() {}
public Builder(ClassificationTrainingSettingsResponse defaults) {
Objects.requireNonNull(defaults);
this.allowedTrainingAlgorithms = defaults.allowedTrainingAlgorithms;
this.blockedTrainingAlgorithms = defaults.blockedTrainingAlgorithms;
this.enableDnnTraining = defaults.enableDnnTraining;
this.enableModelExplainability = defaults.enableModelExplainability;
this.enableOnnxCompatibleModels = defaults.enableOnnxCompatibleModels;
this.enableStackEnsemble = defaults.enableStackEnsemble;
this.enableVoteEnsemble = defaults.enableVoteEnsemble;
this.ensembleModelDownloadTimeout = defaults.ensembleModelDownloadTimeout;
this.stackEnsembleSettings = defaults.stackEnsembleSettings;
}
@CustomType.Setter
public Builder allowedTrainingAlgorithms(@Nullable List allowedTrainingAlgorithms) {
this.allowedTrainingAlgorithms = allowedTrainingAlgorithms;
return this;
}
public Builder allowedTrainingAlgorithms(String... allowedTrainingAlgorithms) {
return allowedTrainingAlgorithms(List.of(allowedTrainingAlgorithms));
}
@CustomType.Setter
public Builder blockedTrainingAlgorithms(@Nullable List blockedTrainingAlgorithms) {
this.blockedTrainingAlgorithms = blockedTrainingAlgorithms;
return this;
}
public Builder blockedTrainingAlgorithms(String... blockedTrainingAlgorithms) {
return blockedTrainingAlgorithms(List.of(blockedTrainingAlgorithms));
}
@CustomType.Setter
public Builder enableDnnTraining(@Nullable Boolean enableDnnTraining) {
this.enableDnnTraining = enableDnnTraining;
return this;
}
@CustomType.Setter
public Builder enableModelExplainability(@Nullable Boolean enableModelExplainability) {
this.enableModelExplainability = enableModelExplainability;
return this;
}
@CustomType.Setter
public Builder enableOnnxCompatibleModels(@Nullable Boolean enableOnnxCompatibleModels) {
this.enableOnnxCompatibleModels = enableOnnxCompatibleModels;
return this;
}
@CustomType.Setter
public Builder enableStackEnsemble(@Nullable Boolean enableStackEnsemble) {
this.enableStackEnsemble = enableStackEnsemble;
return this;
}
@CustomType.Setter
public Builder enableVoteEnsemble(@Nullable Boolean enableVoteEnsemble) {
this.enableVoteEnsemble = enableVoteEnsemble;
return this;
}
@CustomType.Setter
public Builder ensembleModelDownloadTimeout(@Nullable String ensembleModelDownloadTimeout) {
this.ensembleModelDownloadTimeout = ensembleModelDownloadTimeout;
return this;
}
@CustomType.Setter
public Builder stackEnsembleSettings(@Nullable StackEnsembleSettingsResponse stackEnsembleSettings) {
this.stackEnsembleSettings = stackEnsembleSettings;
return this;
}
public ClassificationTrainingSettingsResponse build() {
final var _resultValue = new ClassificationTrainingSettingsResponse();
_resultValue.allowedTrainingAlgorithms = allowedTrainingAlgorithms;
_resultValue.blockedTrainingAlgorithms = blockedTrainingAlgorithms;
_resultValue.enableDnnTraining = enableDnnTraining;
_resultValue.enableModelExplainability = enableModelExplainability;
_resultValue.enableOnnxCompatibleModels = enableOnnxCompatibleModels;
_resultValue.enableStackEnsemble = enableStackEnsemble;
_resultValue.enableVoteEnsemble = enableVoteEnsemble;
_resultValue.ensembleModelDownloadTimeout = ensembleModelDownloadTimeout;
_resultValue.stackEnsembleSettings = stackEnsembleSettings;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy