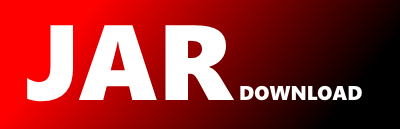
com.pulumi.azurenative.machinelearningservices.outputs.DatastoreResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.machinelearningservices.outputs;
import com.pulumi.azurenative.machinelearningservices.outputs.AzureDataLakeSectionResponse;
import com.pulumi.azurenative.machinelearningservices.outputs.AzureMySqlSectionResponse;
import com.pulumi.azurenative.machinelearningservices.outputs.AzurePostgreSqlSectionResponse;
import com.pulumi.azurenative.machinelearningservices.outputs.AzureSqlDatabaseSectionResponse;
import com.pulumi.azurenative.machinelearningservices.outputs.AzureStorageSectionResponse;
import com.pulumi.azurenative.machinelearningservices.outputs.GlusterFsSectionResponse;
import com.pulumi.azurenative.machinelearningservices.outputs.LinkedInfoResponse;
import com.pulumi.azurenative.machinelearningservices.outputs.UserInfoResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class DatastoreResponse {
private @Nullable AzureDataLakeSectionResponse azureDataLakeSection;
private @Nullable AzureMySqlSectionResponse azureMySqlSection;
private @Nullable AzurePostgreSqlSectionResponse azurePostgreSqlSection;
private @Nullable AzureSqlDatabaseSectionResponse azureSqlDatabaseSection;
private @Nullable AzureStorageSectionResponse azureStorageSection;
/**
* @return The User who created the datastore.
*
*/
private UserInfoResponse createdBy;
/**
* @return The date and time when the datastore was created.
*
*/
private String createdTime;
/**
* @return The datastore type.
*
*/
private @Nullable String dataStoreType;
/**
* @return Description of the datastore.
*
*/
private @Nullable String description;
/**
* @return Data specific to GlusterFS.
*
*/
private @Nullable GlusterFsSectionResponse glusterFsSection;
/**
* @return A read only property that denotes whether the service datastore has been validated with credentials.
*
*/
private @Nullable Boolean hasBeenValidated;
/**
* @return Info about origin if it is linked.
*
*/
private @Nullable LinkedInfoResponse linkedInfo;
/**
* @return The User who modified the datastore.
*
*/
private UserInfoResponse modifiedBy;
/**
* @return The date and time when the datastore was last modified.
*
*/
private String modifiedTime;
/**
* @return Name of the datastore.
*
*/
private @Nullable String name;
/**
* @return Tags for this datastore.
*
*/
private Map tags;
private DatastoreResponse() {}
public Optional azureDataLakeSection() {
return Optional.ofNullable(this.azureDataLakeSection);
}
public Optional azureMySqlSection() {
return Optional.ofNullable(this.azureMySqlSection);
}
public Optional azurePostgreSqlSection() {
return Optional.ofNullable(this.azurePostgreSqlSection);
}
public Optional azureSqlDatabaseSection() {
return Optional.ofNullable(this.azureSqlDatabaseSection);
}
public Optional azureStorageSection() {
return Optional.ofNullable(this.azureStorageSection);
}
/**
* @return The User who created the datastore.
*
*/
public UserInfoResponse createdBy() {
return this.createdBy;
}
/**
* @return The date and time when the datastore was created.
*
*/
public String createdTime() {
return this.createdTime;
}
/**
* @return The datastore type.
*
*/
public Optional dataStoreType() {
return Optional.ofNullable(this.dataStoreType);
}
/**
* @return Description of the datastore.
*
*/
public Optional description() {
return Optional.ofNullable(this.description);
}
/**
* @return Data specific to GlusterFS.
*
*/
public Optional glusterFsSection() {
return Optional.ofNullable(this.glusterFsSection);
}
/**
* @return A read only property that denotes whether the service datastore has been validated with credentials.
*
*/
public Optional hasBeenValidated() {
return Optional.ofNullable(this.hasBeenValidated);
}
/**
* @return Info about origin if it is linked.
*
*/
public Optional linkedInfo() {
return Optional.ofNullable(this.linkedInfo);
}
/**
* @return The User who modified the datastore.
*
*/
public UserInfoResponse modifiedBy() {
return this.modifiedBy;
}
/**
* @return The date and time when the datastore was last modified.
*
*/
public String modifiedTime() {
return this.modifiedTime;
}
/**
* @return Name of the datastore.
*
*/
public Optional name() {
return Optional.ofNullable(this.name);
}
/**
* @return Tags for this datastore.
*
*/
public Map tags() {
return this.tags;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(DatastoreResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable AzureDataLakeSectionResponse azureDataLakeSection;
private @Nullable AzureMySqlSectionResponse azureMySqlSection;
private @Nullable AzurePostgreSqlSectionResponse azurePostgreSqlSection;
private @Nullable AzureSqlDatabaseSectionResponse azureSqlDatabaseSection;
private @Nullable AzureStorageSectionResponse azureStorageSection;
private UserInfoResponse createdBy;
private String createdTime;
private @Nullable String dataStoreType;
private @Nullable String description;
private @Nullable GlusterFsSectionResponse glusterFsSection;
private @Nullable Boolean hasBeenValidated;
private @Nullable LinkedInfoResponse linkedInfo;
private UserInfoResponse modifiedBy;
private String modifiedTime;
private @Nullable String name;
private Map tags;
public Builder() {}
public Builder(DatastoreResponse defaults) {
Objects.requireNonNull(defaults);
this.azureDataLakeSection = defaults.azureDataLakeSection;
this.azureMySqlSection = defaults.azureMySqlSection;
this.azurePostgreSqlSection = defaults.azurePostgreSqlSection;
this.azureSqlDatabaseSection = defaults.azureSqlDatabaseSection;
this.azureStorageSection = defaults.azureStorageSection;
this.createdBy = defaults.createdBy;
this.createdTime = defaults.createdTime;
this.dataStoreType = defaults.dataStoreType;
this.description = defaults.description;
this.glusterFsSection = defaults.glusterFsSection;
this.hasBeenValidated = defaults.hasBeenValidated;
this.linkedInfo = defaults.linkedInfo;
this.modifiedBy = defaults.modifiedBy;
this.modifiedTime = defaults.modifiedTime;
this.name = defaults.name;
this.tags = defaults.tags;
}
@CustomType.Setter
public Builder azureDataLakeSection(@Nullable AzureDataLakeSectionResponse azureDataLakeSection) {
this.azureDataLakeSection = azureDataLakeSection;
return this;
}
@CustomType.Setter
public Builder azureMySqlSection(@Nullable AzureMySqlSectionResponse azureMySqlSection) {
this.azureMySqlSection = azureMySqlSection;
return this;
}
@CustomType.Setter
public Builder azurePostgreSqlSection(@Nullable AzurePostgreSqlSectionResponse azurePostgreSqlSection) {
this.azurePostgreSqlSection = azurePostgreSqlSection;
return this;
}
@CustomType.Setter
public Builder azureSqlDatabaseSection(@Nullable AzureSqlDatabaseSectionResponse azureSqlDatabaseSection) {
this.azureSqlDatabaseSection = azureSqlDatabaseSection;
return this;
}
@CustomType.Setter
public Builder azureStorageSection(@Nullable AzureStorageSectionResponse azureStorageSection) {
this.azureStorageSection = azureStorageSection;
return this;
}
@CustomType.Setter
public Builder createdBy(UserInfoResponse createdBy) {
if (createdBy == null) {
throw new MissingRequiredPropertyException("DatastoreResponse", "createdBy");
}
this.createdBy = createdBy;
return this;
}
@CustomType.Setter
public Builder createdTime(String createdTime) {
if (createdTime == null) {
throw new MissingRequiredPropertyException("DatastoreResponse", "createdTime");
}
this.createdTime = createdTime;
return this;
}
@CustomType.Setter
public Builder dataStoreType(@Nullable String dataStoreType) {
this.dataStoreType = dataStoreType;
return this;
}
@CustomType.Setter
public Builder description(@Nullable String description) {
this.description = description;
return this;
}
@CustomType.Setter
public Builder glusterFsSection(@Nullable GlusterFsSectionResponse glusterFsSection) {
this.glusterFsSection = glusterFsSection;
return this;
}
@CustomType.Setter
public Builder hasBeenValidated(@Nullable Boolean hasBeenValidated) {
this.hasBeenValidated = hasBeenValidated;
return this;
}
@CustomType.Setter
public Builder linkedInfo(@Nullable LinkedInfoResponse linkedInfo) {
this.linkedInfo = linkedInfo;
return this;
}
@CustomType.Setter
public Builder modifiedBy(UserInfoResponse modifiedBy) {
if (modifiedBy == null) {
throw new MissingRequiredPropertyException("DatastoreResponse", "modifiedBy");
}
this.modifiedBy = modifiedBy;
return this;
}
@CustomType.Setter
public Builder modifiedTime(String modifiedTime) {
if (modifiedTime == null) {
throw new MissingRequiredPropertyException("DatastoreResponse", "modifiedTime");
}
this.modifiedTime = modifiedTime;
return this;
}
@CustomType.Setter
public Builder name(@Nullable String name) {
this.name = name;
return this;
}
@CustomType.Setter
public Builder tags(Map tags) {
if (tags == null) {
throw new MissingRequiredPropertyException("DatastoreResponse", "tags");
}
this.tags = tags;
return this;
}
public DatastoreResponse build() {
final var _resultValue = new DatastoreResponse();
_resultValue.azureDataLakeSection = azureDataLakeSection;
_resultValue.azureMySqlSection = azureMySqlSection;
_resultValue.azurePostgreSqlSection = azurePostgreSqlSection;
_resultValue.azureSqlDatabaseSection = azureSqlDatabaseSection;
_resultValue.azureStorageSection = azureStorageSection;
_resultValue.createdBy = createdBy;
_resultValue.createdTime = createdTime;
_resultValue.dataStoreType = dataStoreType;
_resultValue.description = description;
_resultValue.glusterFsSection = glusterFsSection;
_resultValue.hasBeenValidated = hasBeenValidated;
_resultValue.linkedInfo = linkedInfo;
_resultValue.modifiedBy = modifiedBy;
_resultValue.modifiedTime = modifiedTime;
_resultValue.name = name;
_resultValue.tags = tags;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy