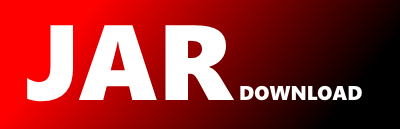
com.pulumi.azurenative.machinelearningservices.outputs.ForecastingSettingsResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.machinelearningservices.outputs;
import com.pulumi.azurenative.machinelearningservices.outputs.AutoForecastHorizonResponse;
import com.pulumi.azurenative.machinelearningservices.outputs.AutoSeasonalityResponse;
import com.pulumi.azurenative.machinelearningservices.outputs.AutoTargetLagsResponse;
import com.pulumi.azurenative.machinelearningservices.outputs.AutoTargetRollingWindowSizeResponse;
import com.pulumi.azurenative.machinelearningservices.outputs.CustomForecastHorizonResponse;
import com.pulumi.azurenative.machinelearningservices.outputs.CustomSeasonalityResponse;
import com.pulumi.azurenative.machinelearningservices.outputs.CustomTargetLagsResponse;
import com.pulumi.azurenative.machinelearningservices.outputs.CustomTargetRollingWindowSizeResponse;
import com.pulumi.core.Either;
import com.pulumi.core.annotations.CustomType;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ForecastingSettingsResponse {
/**
* @return Country or region for holidays for forecasting tasks.
* These should be ISO 3166 two-letter country/region codes, for example 'US' or 'GB'.
*
*/
private @Nullable String countryOrRegionForHolidays;
/**
* @return Number of periods between the origin time of one CV fold and the next fold. For
* example, if `CVStepSize` = 3 for daily data, the origin time for each fold will be
* three days apart.
*
*/
private @Nullable Integer cvStepSize;
/**
* @return Flag for generating lags for the numeric features with 'auto' or null.
*
*/
private @Nullable String featureLags;
/**
* @return The desired maximum forecast horizon in units of time-series frequency.
*
*/
private @Nullable Either forecastHorizon;
/**
* @return When forecasting, this parameter represents the period with which the forecast is desired, for example daily, weekly, yearly, etc. The forecast frequency is dataset frequency by default.
*
*/
private @Nullable String frequency;
/**
* @return Set time series seasonality as an integer multiple of the series frequency.
* If seasonality is set to 'auto', it will be inferred.
*
*/
private @Nullable Either seasonality;
/**
* @return The parameter defining how if AutoML should handle short time series.
*
*/
private @Nullable String shortSeriesHandlingConfig;
/**
* @return The function to be used to aggregate the time series target column to conform to a user specified frequency.
* If the TargetAggregateFunction is set i.e. not 'None', but the freq parameter is not set, the error is raised. The possible target aggregation functions are: "sum", "max", "min" and "mean".
*
*/
private @Nullable String targetAggregateFunction;
/**
* @return The number of past periods to lag from the target column.
*
*/
private @Nullable Either targetLags;
/**
* @return The number of past periods used to create a rolling window average of the target column.
*
*/
private @Nullable Either targetRollingWindowSize;
/**
* @return The name of the time column. This parameter is required when forecasting to specify the datetime column in the input data used for building the time series and inferring its frequency.
*
*/
private @Nullable String timeColumnName;
/**
* @return The names of columns used to group a timeseries. It can be used to create multiple series.
* If grain is not defined, the data set is assumed to be one time-series. This parameter is used with task type forecasting.
*
*/
private @Nullable List timeSeriesIdColumnNames;
/**
* @return Configure STL Decomposition of the time-series target column.
*
*/
private @Nullable String useStl;
private ForecastingSettingsResponse() {}
/**
* @return Country or region for holidays for forecasting tasks.
* These should be ISO 3166 two-letter country/region codes, for example 'US' or 'GB'.
*
*/
public Optional countryOrRegionForHolidays() {
return Optional.ofNullable(this.countryOrRegionForHolidays);
}
/**
* @return Number of periods between the origin time of one CV fold and the next fold. For
* example, if `CVStepSize` = 3 for daily data, the origin time for each fold will be
* three days apart.
*
*/
public Optional cvStepSize() {
return Optional.ofNullable(this.cvStepSize);
}
/**
* @return Flag for generating lags for the numeric features with 'auto' or null.
*
*/
public Optional featureLags() {
return Optional.ofNullable(this.featureLags);
}
/**
* @return The desired maximum forecast horizon in units of time-series frequency.
*
*/
public Optional> forecastHorizon() {
return Optional.ofNullable(this.forecastHorizon);
}
/**
* @return When forecasting, this parameter represents the period with which the forecast is desired, for example daily, weekly, yearly, etc. The forecast frequency is dataset frequency by default.
*
*/
public Optional frequency() {
return Optional.ofNullable(this.frequency);
}
/**
* @return Set time series seasonality as an integer multiple of the series frequency.
* If seasonality is set to 'auto', it will be inferred.
*
*/
public Optional> seasonality() {
return Optional.ofNullable(this.seasonality);
}
/**
* @return The parameter defining how if AutoML should handle short time series.
*
*/
public Optional shortSeriesHandlingConfig() {
return Optional.ofNullable(this.shortSeriesHandlingConfig);
}
/**
* @return The function to be used to aggregate the time series target column to conform to a user specified frequency.
* If the TargetAggregateFunction is set i.e. not 'None', but the freq parameter is not set, the error is raised. The possible target aggregation functions are: "sum", "max", "min" and "mean".
*
*/
public Optional targetAggregateFunction() {
return Optional.ofNullable(this.targetAggregateFunction);
}
/**
* @return The number of past periods to lag from the target column.
*
*/
public Optional> targetLags() {
return Optional.ofNullable(this.targetLags);
}
/**
* @return The number of past periods used to create a rolling window average of the target column.
*
*/
public Optional> targetRollingWindowSize() {
return Optional.ofNullable(this.targetRollingWindowSize);
}
/**
* @return The name of the time column. This parameter is required when forecasting to specify the datetime column in the input data used for building the time series and inferring its frequency.
*
*/
public Optional timeColumnName() {
return Optional.ofNullable(this.timeColumnName);
}
/**
* @return The names of columns used to group a timeseries. It can be used to create multiple series.
* If grain is not defined, the data set is assumed to be one time-series. This parameter is used with task type forecasting.
*
*/
public List timeSeriesIdColumnNames() {
return this.timeSeriesIdColumnNames == null ? List.of() : this.timeSeriesIdColumnNames;
}
/**
* @return Configure STL Decomposition of the time-series target column.
*
*/
public Optional useStl() {
return Optional.ofNullable(this.useStl);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ForecastingSettingsResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String countryOrRegionForHolidays;
private @Nullable Integer cvStepSize;
private @Nullable String featureLags;
private @Nullable Either forecastHorizon;
private @Nullable String frequency;
private @Nullable Either seasonality;
private @Nullable String shortSeriesHandlingConfig;
private @Nullable String targetAggregateFunction;
private @Nullable Either targetLags;
private @Nullable Either targetRollingWindowSize;
private @Nullable String timeColumnName;
private @Nullable List timeSeriesIdColumnNames;
private @Nullable String useStl;
public Builder() {}
public Builder(ForecastingSettingsResponse defaults) {
Objects.requireNonNull(defaults);
this.countryOrRegionForHolidays = defaults.countryOrRegionForHolidays;
this.cvStepSize = defaults.cvStepSize;
this.featureLags = defaults.featureLags;
this.forecastHorizon = defaults.forecastHorizon;
this.frequency = defaults.frequency;
this.seasonality = defaults.seasonality;
this.shortSeriesHandlingConfig = defaults.shortSeriesHandlingConfig;
this.targetAggregateFunction = defaults.targetAggregateFunction;
this.targetLags = defaults.targetLags;
this.targetRollingWindowSize = defaults.targetRollingWindowSize;
this.timeColumnName = defaults.timeColumnName;
this.timeSeriesIdColumnNames = defaults.timeSeriesIdColumnNames;
this.useStl = defaults.useStl;
}
@CustomType.Setter
public Builder countryOrRegionForHolidays(@Nullable String countryOrRegionForHolidays) {
this.countryOrRegionForHolidays = countryOrRegionForHolidays;
return this;
}
@CustomType.Setter
public Builder cvStepSize(@Nullable Integer cvStepSize) {
this.cvStepSize = cvStepSize;
return this;
}
@CustomType.Setter
public Builder featureLags(@Nullable String featureLags) {
this.featureLags = featureLags;
return this;
}
@CustomType.Setter
public Builder forecastHorizon(@Nullable Either forecastHorizon) {
this.forecastHorizon = forecastHorizon;
return this;
}
@CustomType.Setter
public Builder frequency(@Nullable String frequency) {
this.frequency = frequency;
return this;
}
@CustomType.Setter
public Builder seasonality(@Nullable Either seasonality) {
this.seasonality = seasonality;
return this;
}
@CustomType.Setter
public Builder shortSeriesHandlingConfig(@Nullable String shortSeriesHandlingConfig) {
this.shortSeriesHandlingConfig = shortSeriesHandlingConfig;
return this;
}
@CustomType.Setter
public Builder targetAggregateFunction(@Nullable String targetAggregateFunction) {
this.targetAggregateFunction = targetAggregateFunction;
return this;
}
@CustomType.Setter
public Builder targetLags(@Nullable Either targetLags) {
this.targetLags = targetLags;
return this;
}
@CustomType.Setter
public Builder targetRollingWindowSize(@Nullable Either targetRollingWindowSize) {
this.targetRollingWindowSize = targetRollingWindowSize;
return this;
}
@CustomType.Setter
public Builder timeColumnName(@Nullable String timeColumnName) {
this.timeColumnName = timeColumnName;
return this;
}
@CustomType.Setter
public Builder timeSeriesIdColumnNames(@Nullable List timeSeriesIdColumnNames) {
this.timeSeriesIdColumnNames = timeSeriesIdColumnNames;
return this;
}
public Builder timeSeriesIdColumnNames(String... timeSeriesIdColumnNames) {
return timeSeriesIdColumnNames(List.of(timeSeriesIdColumnNames));
}
@CustomType.Setter
public Builder useStl(@Nullable String useStl) {
this.useStl = useStl;
return this;
}
public ForecastingSettingsResponse build() {
final var _resultValue = new ForecastingSettingsResponse();
_resultValue.countryOrRegionForHolidays = countryOrRegionForHolidays;
_resultValue.cvStepSize = cvStepSize;
_resultValue.featureLags = featureLags;
_resultValue.forecastHorizon = forecastHorizon;
_resultValue.frequency = frequency;
_resultValue.seasonality = seasonality;
_resultValue.shortSeriesHandlingConfig = shortSeriesHandlingConfig;
_resultValue.targetAggregateFunction = targetAggregateFunction;
_resultValue.targetLags = targetLags;
_resultValue.targetRollingWindowSize = targetRollingWindowSize;
_resultValue.timeColumnName = timeColumnName;
_resultValue.timeSeriesIdColumnNames = timeSeriesIdColumnNames;
_resultValue.useStl = useStl;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy