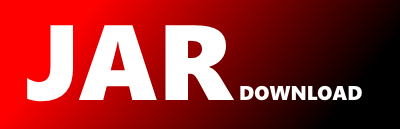
com.pulumi.azurenative.machinelearningservices.outputs.KubernetesOnlineDeploymentResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.machinelearningservices.outputs;
import com.pulumi.azurenative.machinelearningservices.outputs.CodeConfigurationResponse;
import com.pulumi.azurenative.machinelearningservices.outputs.ContainerResourceRequirementsResponse;
import com.pulumi.azurenative.machinelearningservices.outputs.DefaultScaleSettingsResponse;
import com.pulumi.azurenative.machinelearningservices.outputs.OnlineRequestSettingsResponse;
import com.pulumi.azurenative.machinelearningservices.outputs.ProbeSettingsResponse;
import com.pulumi.azurenative.machinelearningservices.outputs.TargetUtilizationScaleSettingsResponse;
import com.pulumi.core.Either;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class KubernetesOnlineDeploymentResponse {
/**
* @return If true, enables Application Insights logging.
*
*/
private @Nullable Boolean appInsightsEnabled;
/**
* @return Code configuration for the endpoint deployment.
*
*/
private @Nullable CodeConfigurationResponse codeConfiguration;
/**
* @return The resource requirements for the container (cpu and memory).
*
*/
private @Nullable ContainerResourceRequirementsResponse containerResourceRequirements;
/**
* @return Description of the endpoint deployment.
*
*/
private @Nullable String description;
/**
* @return If Enabled, allow egress public network access. If Disabled, this will create secure egress. Default: Enabled.
*
*/
private @Nullable String egressPublicNetworkAccess;
/**
* @return Enum to determine endpoint compute type.
* Expected value is 'Kubernetes'.
*
*/
private String endpointComputeType;
/**
* @return ARM resource ID or AssetId of the environment specification for the endpoint deployment.
*
*/
private @Nullable String environmentId;
/**
* @return Environment variables configuration for the deployment.
*
*/
private @Nullable Map environmentVariables;
/**
* @return Compute instance type.
*
*/
private @Nullable String instanceType;
/**
* @return Liveness probe monitors the health of the container regularly.
*
*/
private @Nullable ProbeSettingsResponse livenessProbe;
/**
* @return The URI path to the model.
*
*/
private @Nullable String model;
/**
* @return The path to mount the model in custom container.
*
*/
private @Nullable String modelMountPath;
/**
* @return Property dictionary. Properties can be added, but not removed or altered.
*
*/
private @Nullable Map properties;
/**
* @return Provisioning state for the endpoint deployment.
*
*/
private String provisioningState;
/**
* @return Readiness probe validates if the container is ready to serve traffic. The properties and defaults are the same as liveness probe.
*
*/
private @Nullable ProbeSettingsResponse readinessProbe;
/**
* @return Request settings for the deployment.
*
*/
private @Nullable OnlineRequestSettingsResponse requestSettings;
/**
* @return Scale settings for the deployment.
* If it is null or not provided,
* it defaults to TargetUtilizationScaleSettings for KubernetesOnlineDeployment
* and to DefaultScaleSettings for ManagedOnlineDeployment.
*
*/
private @Nullable Either scaleSettings;
private KubernetesOnlineDeploymentResponse() {}
/**
* @return If true, enables Application Insights logging.
*
*/
public Optional appInsightsEnabled() {
return Optional.ofNullable(this.appInsightsEnabled);
}
/**
* @return Code configuration for the endpoint deployment.
*
*/
public Optional codeConfiguration() {
return Optional.ofNullable(this.codeConfiguration);
}
/**
* @return The resource requirements for the container (cpu and memory).
*
*/
public Optional containerResourceRequirements() {
return Optional.ofNullable(this.containerResourceRequirements);
}
/**
* @return Description of the endpoint deployment.
*
*/
public Optional description() {
return Optional.ofNullable(this.description);
}
/**
* @return If Enabled, allow egress public network access. If Disabled, this will create secure egress. Default: Enabled.
*
*/
public Optional egressPublicNetworkAccess() {
return Optional.ofNullable(this.egressPublicNetworkAccess);
}
/**
* @return Enum to determine endpoint compute type.
* Expected value is 'Kubernetes'.
*
*/
public String endpointComputeType() {
return this.endpointComputeType;
}
/**
* @return ARM resource ID or AssetId of the environment specification for the endpoint deployment.
*
*/
public Optional environmentId() {
return Optional.ofNullable(this.environmentId);
}
/**
* @return Environment variables configuration for the deployment.
*
*/
public Map environmentVariables() {
return this.environmentVariables == null ? Map.of() : this.environmentVariables;
}
/**
* @return Compute instance type.
*
*/
public Optional instanceType() {
return Optional.ofNullable(this.instanceType);
}
/**
* @return Liveness probe monitors the health of the container regularly.
*
*/
public Optional livenessProbe() {
return Optional.ofNullable(this.livenessProbe);
}
/**
* @return The URI path to the model.
*
*/
public Optional model() {
return Optional.ofNullable(this.model);
}
/**
* @return The path to mount the model in custom container.
*
*/
public Optional modelMountPath() {
return Optional.ofNullable(this.modelMountPath);
}
/**
* @return Property dictionary. Properties can be added, but not removed or altered.
*
*/
public Map properties() {
return this.properties == null ? Map.of() : this.properties;
}
/**
* @return Provisioning state for the endpoint deployment.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return Readiness probe validates if the container is ready to serve traffic. The properties and defaults are the same as liveness probe.
*
*/
public Optional readinessProbe() {
return Optional.ofNullable(this.readinessProbe);
}
/**
* @return Request settings for the deployment.
*
*/
public Optional requestSettings() {
return Optional.ofNullable(this.requestSettings);
}
/**
* @return Scale settings for the deployment.
* If it is null or not provided,
* it defaults to TargetUtilizationScaleSettings for KubernetesOnlineDeployment
* and to DefaultScaleSettings for ManagedOnlineDeployment.
*
*/
public Optional> scaleSettings() {
return Optional.ofNullable(this.scaleSettings);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(KubernetesOnlineDeploymentResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Boolean appInsightsEnabled;
private @Nullable CodeConfigurationResponse codeConfiguration;
private @Nullable ContainerResourceRequirementsResponse containerResourceRequirements;
private @Nullable String description;
private @Nullable String egressPublicNetworkAccess;
private String endpointComputeType;
private @Nullable String environmentId;
private @Nullable Map environmentVariables;
private @Nullable String instanceType;
private @Nullable ProbeSettingsResponse livenessProbe;
private @Nullable String model;
private @Nullable String modelMountPath;
private @Nullable Map properties;
private String provisioningState;
private @Nullable ProbeSettingsResponse readinessProbe;
private @Nullable OnlineRequestSettingsResponse requestSettings;
private @Nullable Either scaleSettings;
public Builder() {}
public Builder(KubernetesOnlineDeploymentResponse defaults) {
Objects.requireNonNull(defaults);
this.appInsightsEnabled = defaults.appInsightsEnabled;
this.codeConfiguration = defaults.codeConfiguration;
this.containerResourceRequirements = defaults.containerResourceRequirements;
this.description = defaults.description;
this.egressPublicNetworkAccess = defaults.egressPublicNetworkAccess;
this.endpointComputeType = defaults.endpointComputeType;
this.environmentId = defaults.environmentId;
this.environmentVariables = defaults.environmentVariables;
this.instanceType = defaults.instanceType;
this.livenessProbe = defaults.livenessProbe;
this.model = defaults.model;
this.modelMountPath = defaults.modelMountPath;
this.properties = defaults.properties;
this.provisioningState = defaults.provisioningState;
this.readinessProbe = defaults.readinessProbe;
this.requestSettings = defaults.requestSettings;
this.scaleSettings = defaults.scaleSettings;
}
@CustomType.Setter
public Builder appInsightsEnabled(@Nullable Boolean appInsightsEnabled) {
this.appInsightsEnabled = appInsightsEnabled;
return this;
}
@CustomType.Setter
public Builder codeConfiguration(@Nullable CodeConfigurationResponse codeConfiguration) {
this.codeConfiguration = codeConfiguration;
return this;
}
@CustomType.Setter
public Builder containerResourceRequirements(@Nullable ContainerResourceRequirementsResponse containerResourceRequirements) {
this.containerResourceRequirements = containerResourceRequirements;
return this;
}
@CustomType.Setter
public Builder description(@Nullable String description) {
this.description = description;
return this;
}
@CustomType.Setter
public Builder egressPublicNetworkAccess(@Nullable String egressPublicNetworkAccess) {
this.egressPublicNetworkAccess = egressPublicNetworkAccess;
return this;
}
@CustomType.Setter
public Builder endpointComputeType(String endpointComputeType) {
if (endpointComputeType == null) {
throw new MissingRequiredPropertyException("KubernetesOnlineDeploymentResponse", "endpointComputeType");
}
this.endpointComputeType = endpointComputeType;
return this;
}
@CustomType.Setter
public Builder environmentId(@Nullable String environmentId) {
this.environmentId = environmentId;
return this;
}
@CustomType.Setter
public Builder environmentVariables(@Nullable Map environmentVariables) {
this.environmentVariables = environmentVariables;
return this;
}
@CustomType.Setter
public Builder instanceType(@Nullable String instanceType) {
this.instanceType = instanceType;
return this;
}
@CustomType.Setter
public Builder livenessProbe(@Nullable ProbeSettingsResponse livenessProbe) {
this.livenessProbe = livenessProbe;
return this;
}
@CustomType.Setter
public Builder model(@Nullable String model) {
this.model = model;
return this;
}
@CustomType.Setter
public Builder modelMountPath(@Nullable String modelMountPath) {
this.modelMountPath = modelMountPath;
return this;
}
@CustomType.Setter
public Builder properties(@Nullable Map properties) {
this.properties = properties;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("KubernetesOnlineDeploymentResponse", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder readinessProbe(@Nullable ProbeSettingsResponse readinessProbe) {
this.readinessProbe = readinessProbe;
return this;
}
@CustomType.Setter
public Builder requestSettings(@Nullable OnlineRequestSettingsResponse requestSettings) {
this.requestSettings = requestSettings;
return this;
}
@CustomType.Setter
public Builder scaleSettings(@Nullable Either scaleSettings) {
this.scaleSettings = scaleSettings;
return this;
}
public KubernetesOnlineDeploymentResponse build() {
final var _resultValue = new KubernetesOnlineDeploymentResponse();
_resultValue.appInsightsEnabled = appInsightsEnabled;
_resultValue.codeConfiguration = codeConfiguration;
_resultValue.containerResourceRequirements = containerResourceRequirements;
_resultValue.description = description;
_resultValue.egressPublicNetworkAccess = egressPublicNetworkAccess;
_resultValue.endpointComputeType = endpointComputeType;
_resultValue.environmentId = environmentId;
_resultValue.environmentVariables = environmentVariables;
_resultValue.instanceType = instanceType;
_resultValue.livenessProbe = livenessProbe;
_resultValue.model = model;
_resultValue.modelMountPath = modelMountPath;
_resultValue.properties = properties;
_resultValue.provisioningState = provisioningState;
_resultValue.readinessProbe = readinessProbe;
_resultValue.requestSettings = requestSettings;
_resultValue.scaleSettings = scaleSettings;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy