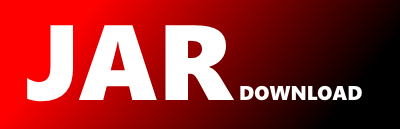
com.pulumi.azurenative.managednetworkfabric.inputs.NetworkTapRuleMatchConfigurationArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.managednetworkfabric.inputs;
import com.pulumi.azurenative.managednetworkfabric.enums.IPAddressType;
import com.pulumi.azurenative.managednetworkfabric.inputs.NetworkTapRuleActionArgs;
import com.pulumi.azurenative.managednetworkfabric.inputs.NetworkTapRuleMatchConditionArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Defines the match configuration that are supported to filter the traffic.
*
*/
public final class NetworkTapRuleMatchConfigurationArgs extends com.pulumi.resources.ResourceArgs {
public static final NetworkTapRuleMatchConfigurationArgs Empty = new NetworkTapRuleMatchConfigurationArgs();
/**
* List of actions that need to be performed for the matched conditions.
*
*/
@Import(name="actions")
private @Nullable Output> actions;
/**
* @return List of actions that need to be performed for the matched conditions.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy