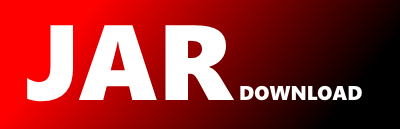
com.pulumi.azurenative.managednetworkfabric.outputs.BgpConfigurationResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.managednetworkfabric.outputs;
import com.pulumi.azurenative.managednetworkfabric.outputs.BfdConfigurationResponse;
import com.pulumi.azurenative.managednetworkfabric.outputs.NeighborAddressResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class BgpConfigurationResponse {
/**
* @return Allows for routes to be received and processed even if the router detects its own ASN in the AS-Path. 0 is disable, Possible values are 1-10, default is 2.
*
*/
private @Nullable Integer allowAS;
/**
* @return Enable Or Disable state.
*
*/
private @Nullable String allowASOverride;
/**
* @return Switch configuration description.
*
*/
private @Nullable String annotation;
/**
* @return BFD configuration properties
*
*/
private @Nullable BfdConfigurationResponse bfdConfiguration;
/**
* @return Originate a defaultRoute. Ex: "True" | "False".
*
*/
private @Nullable String defaultRouteOriginate;
/**
* @return ASN of Network Fabric. Example: 65048.
*
*/
private Integer fabricASN;
/**
* @return BGP Ipv4 ListenRange.
*
*/
private @Nullable List ipv4ListenRangePrefixes;
/**
* @return List with stringified ipv4NeighborAddresses.
*
*/
private @Nullable List ipv4NeighborAddress;
/**
* @return BGP Ipv6 ListenRange.
*
*/
private @Nullable List ipv6ListenRangePrefixes;
/**
* @return List with stringified IPv6 Neighbor Address.
*
*/
private @Nullable List ipv6NeighborAddress;
/**
* @return Peer ASN. Example: 65047.
*
*/
private Integer peerASN;
private BgpConfigurationResponse() {}
/**
* @return Allows for routes to be received and processed even if the router detects its own ASN in the AS-Path. 0 is disable, Possible values are 1-10, default is 2.
*
*/
public Optional allowAS() {
return Optional.ofNullable(this.allowAS);
}
/**
* @return Enable Or Disable state.
*
*/
public Optional allowASOverride() {
return Optional.ofNullable(this.allowASOverride);
}
/**
* @return Switch configuration description.
*
*/
public Optional annotation() {
return Optional.ofNullable(this.annotation);
}
/**
* @return BFD configuration properties
*
*/
public Optional bfdConfiguration() {
return Optional.ofNullable(this.bfdConfiguration);
}
/**
* @return Originate a defaultRoute. Ex: "True" | "False".
*
*/
public Optional defaultRouteOriginate() {
return Optional.ofNullable(this.defaultRouteOriginate);
}
/**
* @return ASN of Network Fabric. Example: 65048.
*
*/
public Integer fabricASN() {
return this.fabricASN;
}
/**
* @return BGP Ipv4 ListenRange.
*
*/
public List ipv4ListenRangePrefixes() {
return this.ipv4ListenRangePrefixes == null ? List.of() : this.ipv4ListenRangePrefixes;
}
/**
* @return List with stringified ipv4NeighborAddresses.
*
*/
public List ipv4NeighborAddress() {
return this.ipv4NeighborAddress == null ? List.of() : this.ipv4NeighborAddress;
}
/**
* @return BGP Ipv6 ListenRange.
*
*/
public List ipv6ListenRangePrefixes() {
return this.ipv6ListenRangePrefixes == null ? List.of() : this.ipv6ListenRangePrefixes;
}
/**
* @return List with stringified IPv6 Neighbor Address.
*
*/
public List ipv6NeighborAddress() {
return this.ipv6NeighborAddress == null ? List.of() : this.ipv6NeighborAddress;
}
/**
* @return Peer ASN. Example: 65047.
*
*/
public Integer peerASN() {
return this.peerASN;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(BgpConfigurationResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Integer allowAS;
private @Nullable String allowASOverride;
private @Nullable String annotation;
private @Nullable BfdConfigurationResponse bfdConfiguration;
private @Nullable String defaultRouteOriginate;
private Integer fabricASN;
private @Nullable List ipv4ListenRangePrefixes;
private @Nullable List ipv4NeighborAddress;
private @Nullable List ipv6ListenRangePrefixes;
private @Nullable List ipv6NeighborAddress;
private Integer peerASN;
public Builder() {}
public Builder(BgpConfigurationResponse defaults) {
Objects.requireNonNull(defaults);
this.allowAS = defaults.allowAS;
this.allowASOverride = defaults.allowASOverride;
this.annotation = defaults.annotation;
this.bfdConfiguration = defaults.bfdConfiguration;
this.defaultRouteOriginate = defaults.defaultRouteOriginate;
this.fabricASN = defaults.fabricASN;
this.ipv4ListenRangePrefixes = defaults.ipv4ListenRangePrefixes;
this.ipv4NeighborAddress = defaults.ipv4NeighborAddress;
this.ipv6ListenRangePrefixes = defaults.ipv6ListenRangePrefixes;
this.ipv6NeighborAddress = defaults.ipv6NeighborAddress;
this.peerASN = defaults.peerASN;
}
@CustomType.Setter
public Builder allowAS(@Nullable Integer allowAS) {
this.allowAS = allowAS;
return this;
}
@CustomType.Setter
public Builder allowASOverride(@Nullable String allowASOverride) {
this.allowASOverride = allowASOverride;
return this;
}
@CustomType.Setter
public Builder annotation(@Nullable String annotation) {
this.annotation = annotation;
return this;
}
@CustomType.Setter
public Builder bfdConfiguration(@Nullable BfdConfigurationResponse bfdConfiguration) {
this.bfdConfiguration = bfdConfiguration;
return this;
}
@CustomType.Setter
public Builder defaultRouteOriginate(@Nullable String defaultRouteOriginate) {
this.defaultRouteOriginate = defaultRouteOriginate;
return this;
}
@CustomType.Setter
public Builder fabricASN(Integer fabricASN) {
if (fabricASN == null) {
throw new MissingRequiredPropertyException("BgpConfigurationResponse", "fabricASN");
}
this.fabricASN = fabricASN;
return this;
}
@CustomType.Setter
public Builder ipv4ListenRangePrefixes(@Nullable List ipv4ListenRangePrefixes) {
this.ipv4ListenRangePrefixes = ipv4ListenRangePrefixes;
return this;
}
public Builder ipv4ListenRangePrefixes(String... ipv4ListenRangePrefixes) {
return ipv4ListenRangePrefixes(List.of(ipv4ListenRangePrefixes));
}
@CustomType.Setter
public Builder ipv4NeighborAddress(@Nullable List ipv4NeighborAddress) {
this.ipv4NeighborAddress = ipv4NeighborAddress;
return this;
}
public Builder ipv4NeighborAddress(NeighborAddressResponse... ipv4NeighborAddress) {
return ipv4NeighborAddress(List.of(ipv4NeighborAddress));
}
@CustomType.Setter
public Builder ipv6ListenRangePrefixes(@Nullable List ipv6ListenRangePrefixes) {
this.ipv6ListenRangePrefixes = ipv6ListenRangePrefixes;
return this;
}
public Builder ipv6ListenRangePrefixes(String... ipv6ListenRangePrefixes) {
return ipv6ListenRangePrefixes(List.of(ipv6ListenRangePrefixes));
}
@CustomType.Setter
public Builder ipv6NeighborAddress(@Nullable List ipv6NeighborAddress) {
this.ipv6NeighborAddress = ipv6NeighborAddress;
return this;
}
public Builder ipv6NeighborAddress(NeighborAddressResponse... ipv6NeighborAddress) {
return ipv6NeighborAddress(List.of(ipv6NeighborAddress));
}
@CustomType.Setter
public Builder peerASN(Integer peerASN) {
if (peerASN == null) {
throw new MissingRequiredPropertyException("BgpConfigurationResponse", "peerASN");
}
this.peerASN = peerASN;
return this;
}
public BgpConfigurationResponse build() {
final var _resultValue = new BgpConfigurationResponse();
_resultValue.allowAS = allowAS;
_resultValue.allowASOverride = allowASOverride;
_resultValue.annotation = annotation;
_resultValue.bfdConfiguration = bfdConfiguration;
_resultValue.defaultRouteOriginate = defaultRouteOriginate;
_resultValue.fabricASN = fabricASN;
_resultValue.ipv4ListenRangePrefixes = ipv4ListenRangePrefixes;
_resultValue.ipv4NeighborAddress = ipv4NeighborAddress;
_resultValue.ipv6ListenRangePrefixes = ipv6ListenRangePrefixes;
_resultValue.ipv6NeighborAddress = ipv6NeighborAddress;
_resultValue.peerASN = peerASN;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy