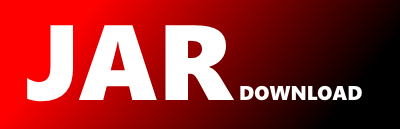
com.pulumi.azurenative.marketplace.PrivateStoreCollectionOfferArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.marketplace;
import com.pulumi.azurenative.marketplace.inputs.PlanArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class PrivateStoreCollectionOfferArgs extends com.pulumi.resources.ResourceArgs {
public static final PrivateStoreCollectionOfferArgs Empty = new PrivateStoreCollectionOfferArgs();
/**
* The collection ID
*
*/
@Import(name="collectionId", required=true)
private Output collectionId;
/**
* @return The collection ID
*
*/
public Output collectionId() {
return this.collectionId;
}
/**
* Identifier for purposes of race condition
*
*/
@Import(name="eTag")
private @Nullable Output eTag;
/**
* @return Identifier for purposes of race condition
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy